187
|
Defines the colors, to display overlapping links
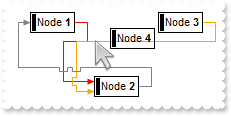
local oSurface,var_Elements
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.AllowLinkControlPoint = 99 /*exAllowChangeTo | exAllowChangeFrom | exEndControlPoint | exStartControlPoint*/
var_Elements = oSurface.Elements
var_Elements.Add("Node <b>1",-64,-64)
var_Elements.Add("Node <b>2")
var_Elements.Add("Node <b>3",64,-64)
var_Elements.Add("Node <b>4",16,-48)
var_Elements.Add("Node <b>5",-1024,-48)
var_Elements.Add("Node <b>6",16,512)
oSurface.Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2),"L1")
oSurface.Links.Add(oSurface.Elements.Item(3),oSurface.Elements.Item(2),"L2")
oSurface.Links.Add(oSurface.Elements.Item(2),oSurface.Elements.Item(1),"L3")
oSurface.ShowLinks = 545 /*exChangeColorOnOverlap | exShowCrossLinksRect | exShowExtendedLinks*/
oSurface.OverlapLinksColors = "red,orange"
oSurface.LinksColor = 0x808080
oSurface.AxisStyle = -1
oSurface.ShowGridLines = false
oSurface.EndUpdate()
|
186
|
The exPreventOverlapMixt flag must always be used alongside either the exPreventOverlap or exChangeColorOnOverlap flag. When used with the exPreventOverlap flag, it ensures that links avoid overlapping with elements or obstacles, enabling their paths to include both rectangular and diagonal lines. When combined with the exChangeColorOnOverlap flag, overlapping links alternately adjust their width in addition to changing colors (sample 2)
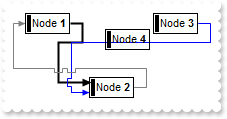
local oSurface,var_Elements
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.AllowLinkControlPoint = 99 /*exAllowChangeTo | exAllowChangeFrom | exEndControlPoint | exStartControlPoint*/
var_Elements = oSurface.Elements
var_Elements.Add("Node <b>1",-64,-64)
var_Elements.Add("Node <b>2")
var_Elements.Add("Node <b>3",64,-64)
var_Elements.Add("Node <b>4",16,-48)
var_Elements.Add("Node <b>5",-1024,-48)
var_Elements.Add("Node <b>6",16,512)
oSurface.Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2),"L1")
oSurface.Links.Add(oSurface.Elements.Item(3),oSurface.Elements.Item(2),"L2")
oSurface.Links.Add(oSurface.Elements.Item(2),oSurface.Elements.Item(1),"L3")
oSurface.ShowLinks = 673 /*exChangeColorOnOverlap | exPreventOverlapMixt | exShowCrossLinksRect | exShowExtendedLinks*/
oSurface.LinksColor = 0x808080
oSurface.AxisStyle = -1
oSurface.ShowGridLines = false
oSurface.EndUpdate()
|
185
|
The exChangeColorOnOverlap flag changes the color for links in areas where they overlap with other links, enhancing clarity and distinction between them
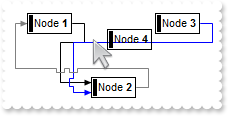
local oSurface,var_Elements
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.AllowLinkControlPoint = 99 /*exAllowChangeTo | exAllowChangeFrom | exEndControlPoint | exStartControlPoint*/
var_Elements = oSurface.Elements
var_Elements.Add("Node <b>1",-64,-64)
var_Elements.Add("Node <b>2")
var_Elements.Add("Node <b>3",64,-64)
var_Elements.Add("Node <b>4",16,-48)
var_Elements.Add("Node <b>5",-1024,-48)
var_Elements.Add("Node <b>6",16,512)
oSurface.Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2),"L1")
oSurface.Links.Add(oSurface.Elements.Item(3),oSurface.Elements.Item(2),"L2")
oSurface.Links.Add(oSurface.Elements.Item(2),oSurface.Elements.Item(1),"L3")
oSurface.ShowLinks = 545 /*exChangeColorOnOverlap | exShowCrossLinksRect | exShowExtendedLinks*/
oSurface.LinksColor = 0x808080
oSurface.AxisStyle = -1
oSurface.ShowGridLines = false
oSurface.EndUpdate()
|
184
|
The exPreventOverlapMixt flag must always be used alongside either the exPreventOverlap or exChangeColorOnOverlap flag. When used with the exPreventOverlap flag, it ensures that links avoid overlapping with elements or obstacles, enabling their paths to include both rectangular and diagonal lines. When combined with the exChangeColorOnOverlap flag, overlapping links alternately adjust their width in addition to changing colors (sample 1)
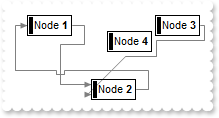
local oSurface,var_Elements
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.AllowLinkControlPoint = 99 /*exAllowChangeTo | exAllowChangeFrom | exEndControlPoint | exStartControlPoint*/
var_Elements = oSurface.Elements
var_Elements.Add("Node <b>1",-64,-64)
var_Elements.Add("Node <b>2")
var_Elements.Add("Node <b>3",64,-64)
var_Elements.Add("Node <b>4",16,-48)
var_Elements.Add("Node <b>5",-1024,-48)
var_Elements.Add("Node <b>6",16,512)
oSurface.Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2),"L1")
oSurface.Links.Add(oSurface.Elements.Item(3),oSurface.Elements.Item(2),"L2")
oSurface.Links.Add(oSurface.Elements.Item(2),oSurface.Elements.Item(1),"L3")
oSurface.ShowLinks = 417 /*exPreventOverlap | exPreventOverlapMixt | exShowCrossLinksRect | exShowExtendedLinks*/
oSurface.LinksColor = 0x808080
oSurface.AxisStyle = -1
oSurface.ShowGridLines = false
oSurface.EndUpdate()
|
183
|
The exPreventOverlap flag adjusts the links to prevent them from overlapping the connected objects. The exPreventOverlap option calculates the path between A and B using the A* (A-star) pathfinding algorithm, which can be a time-consuming operation
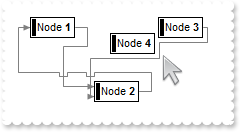
local oSurface,var_Elements
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.AllowLinkControlPoint = 99 /*exAllowChangeTo | exAllowChangeFrom | exEndControlPoint | exStartControlPoint*/
var_Elements = oSurface.Elements
var_Elements.Add("Node <b>1",-64,-64)
var_Elements.Add("Node <b>2")
var_Elements.Add("Node <b>3",64,-64)
var_Elements.Add("Node <b>4",16,-48)
var_Elements.Add("Node <b>5",-1024,-48)
var_Elements.Add("Node <b>6",16,512)
oSurface.Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2),"L1")
oSurface.Links.Add(oSurface.Elements.Item(3),oSurface.Elements.Item(2),"L2")
oSurface.Links.Add(oSurface.Elements.Item(2),oSurface.Elements.Item(1),"L3")
oSurface.ShowLinks = 289 /*exPreventOverlap | exShowCrossLinksRect | exShowExtendedLinks*/
oSurface.LinksColor = 0x808080
oSurface.AxisStyle = -1
oSurface.ShowGridLines = false
oSurface.EndUpdate()
|
182
|
How can I replace or add an icon at runtime
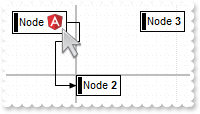
local oSurface,var_Elements
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.ReplaceIcon("gAAAABgYACEHgUJFEEAAWhUJCEJEEJggEhMCYEXjUbjkJQECj8gj8hAEjkshYEpk8kf8ClsulsvAExmcvf83js5nU7nkCeEcn8boMaocXosCB9Hn09pkzcEuoL/fE+OkYB0gB9YhIHrddgVcr9aktZADAD8+P8CgIA==")
oSurface.ReplaceIcon("C:\images\favicon.ico",0)
oSurface.AllowLinkControlPoint = 99 /*exAllowChangeTo | exAllowChangeFrom | exEndControlPoint | exStartControlPoint*/
var_Elements = oSurface.Elements
var_Elements.Add("Node <img>1</img>",-64,-64)
var_Elements.Add("Node <b>2")
var_Elements.Add("Node <b>3",64,-64)
oSurface.Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2),"L1")
oSurface.EndUpdate()
|
181
|
exUndo, An Undo operation is performed (CTR + Z), exRedo, A Redo operation is performed (CTR + Y). exUndoRedoUpdate, The Undo/Redo queue is updated
/*
with (this.EXSURFACEACTIVEXCONTROL1.nativeObject)
LayoutEndChanging = class::nativeObject_LayoutEndChanging
endwith
*/
// Notifies your application once the control's layout has been changed.
function nativeObject_LayoutEndChanging(Operation)
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
? "LayoutEndChanging"
? Str(oSurface.FormatABC("value case (default:value;32:`exUndoRedoUpdate`;33:`exUndo`;34:`exRedo`)",Operation))
return
/*
with (this.EXSURFACEACTIVEXCONTROL1.nativeObject)
LayoutStartChanging = class::nativeObject_LayoutStartChanging
endwith
*/
// Occurs when the control's layout is about to be changed.
function nativeObject_LayoutStartChanging(Operation)
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
? "LayoutStartChanging"
? Str(oSurface.FormatABC("value case (default:value;32:`exUndoRedoUpdate`;33:`exUndo`;34:`exRedo`)",Operation))
return
local oSurface,var_Elements
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.AllowUndoRedo = true
oSurface.AllowLinkControlPoint = 99 /*exAllowChangeTo | exAllowChangeFrom | exEndControlPoint | exStartControlPoint*/
var_Elements = oSurface.Elements
var_Elements.Add("Node <b>1",-64,-64)
var_Elements.Add("Node <b>2")
var_Elements.Add("Node <b>3",64,-64)
oSurface.Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2),"L1")
oSurface.EndUpdate()
? "Press CTRL+Z to Undo, CTRL+Y to Redo"
|
180
|
exLinkObjects, the user creates an element on the surface. The AllowLinkObjects property specifies the keys combination to allow user to link elements on the surface
/*
with (this.EXSURFACEACTIVEXCONTROL1.nativeObject)
LayoutEndChanging = class::nativeObject_LayoutEndChanging
endwith
*/
// Notifies your application once the control's layout has been changed.
function nativeObject_LayoutEndChanging(Operation)
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
? "LayoutEndChanging"
? Str(oSurface.FormatABC("value = 9 ? `exLinkObjects` : value",Operation))
return
/*
with (this.EXSURFACEACTIVEXCONTROL1.nativeObject)
LayoutStartChanging = class::nativeObject_LayoutStartChanging
endwith
*/
// Occurs when the control's layout is about to be changed.
function nativeObject_LayoutStartChanging(Operation)
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
? "LayoutStartChanging"
? Str(oSurface.FormatABC("value = 9 ? `exLinkObjects` : value",Operation))
return
local oSurface,var_Elements
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
var_Elements = oSurface.Elements
var_Elements.Add("Node <b>1",-64,-64)
var_Elements.Add("Node <b>2")
var_Elements.Add("Node <b>3",64,-64)
oSurface.EndUpdate()
? "Hold SHIFT, click an element, and drag to another element to create a link between them"
|
179
|
exEditObject, the user edits the element's caption
/*
with (this.EXSURFACEACTIVEXCONTROL1.nativeObject)
Click = class::nativeObject_Click
endwith
*/
// Occurs when the user presses and then releases the left mouse button over the control.
function nativeObject_Click()
/* ElementFromPoint(-1,-1).Edit(0) */
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
return
/*
with (this.EXSURFACEACTIVEXCONTROL1.nativeObject)
LayoutEndChanging = class::nativeObject_LayoutEndChanging
endwith
*/
// Notifies your application once the control's layout has been changed.
function nativeObject_LayoutEndChanging(Operation)
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
? "LayoutEndChanging"
? Str(oSurface.FormatABC("value = 8 ? `exEditObject` : value",Operation))
return
/*
with (this.EXSURFACEACTIVEXCONTROL1.nativeObject)
LayoutStartChanging = class::nativeObject_LayoutStartChanging
endwith
*/
// Occurs when the control's layout is about to be changed.
function nativeObject_LayoutStartChanging(Operation)
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
? "LayoutStartChanging"
? Str(oSurface.FormatABC("value = 8 ? `exEditObject` : value",Operation))
return
local oSurface,var_Elements
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.AllowLinkControlPoint = 99 /*exAllowChangeTo | exAllowChangeFrom | exEndControlPoint | exStartControlPoint*/
var_Elements = oSurface.Elements
var_Elements.Add("Node <b>1",-64,-64)
var_Elements.Add("Node <b>2")
var_Elements.Add("Node <b>3",64,-64)
oSurface.Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2),"L1")
oSurface.EndUpdate()
? "Click an element to edit its caption"
|
178
|
exCreateObject, the user creates an element on the surface. The AllowCreateObject property specifies the keys combination to allow user to create elements on the surface
/*
with (this.EXSURFACEACTIVEXCONTROL1.nativeObject)
LayoutEndChanging = class::nativeObject_LayoutEndChanging
endwith
*/
// Notifies your application once the control's layout has been changed.
function nativeObject_LayoutEndChanging(Operation)
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
? "LayoutEndChanging"
? Str(oSurface.FormatABC("value = 7 ? `exCreateObject` : value",Operation))
return
/*
with (this.EXSURFACEACTIVEXCONTROL1.nativeObject)
LayoutStartChanging = class::nativeObject_LayoutStartChanging
endwith
*/
// Occurs when the control's layout is about to be changed.
function nativeObject_LayoutStartChanging(Operation)
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
? "LayoutStartChanging"
? Str(oSurface.FormatABC("value = 7 ? `exCreateObject` : value",Operation))
return
local oSurface,var_Element,var_Elements
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.AllowLinkControlPoint = 99 /*exAllowChangeTo | exAllowChangeFrom | exEndControlPoint | exStartControlPoint*/
var_Elements = oSurface.Elements
var_Elements.Add("Node <b>1",-64,-64)
// var_Elements.Add("Node <b>2").Selected = true
var_Element = var_Elements.Add("Node <b>2")
with (oSurface)
TemplateDef = [dim var_Element]
TemplateDef = var_Element
Template = [var_Element.Selected = True]
endwith
var_Elements.Add("Node <b>3",64,-64)
oSurface.Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2),"L1")
oSurface.EndUpdate()
? "Double-click on the surface and immediately drag to a new position to create an element"
|
177
|
exSelectNothing, the user clicks an empty zone of the surface. The AllowSelectNothing property specifies the keys combination to allow user to select nothing on the surface
/*
with (this.EXSURFACEACTIVEXCONTROL1.nativeObject)
LayoutEndChanging = class::nativeObject_LayoutEndChanging
endwith
*/
// Notifies your application once the control's layout has been changed.
function nativeObject_LayoutEndChanging(Operation)
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
? "LayoutEndChanging"
? Str(oSurface.FormatABC("value = 6 ? `exSelectNothing` : value",Operation))
return
/*
with (this.EXSURFACEACTIVEXCONTROL1.nativeObject)
LayoutStartChanging = class::nativeObject_LayoutStartChanging
endwith
*/
// Occurs when the control's layout is about to be changed.
function nativeObject_LayoutStartChanging(Operation)
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
? "LayoutStartChanging"
? Str(oSurface.FormatABC("value = 6 ? `exSelectNothing` : value",Operation))
return
local oSurface,var_Element,var_Elements
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.AllowSelectNothing = true
oSurface.AllowLinkControlPoint = 99 /*exAllowChangeTo | exAllowChangeFrom | exEndControlPoint | exStartControlPoint*/
var_Elements = oSurface.Elements
var_Elements.Add("Node <b>1",-64,-64)
// var_Elements.Add("Node <b>2").Selected = true
var_Element = var_Elements.Add("Node <b>2")
with (oSurface)
TemplateDef = [dim var_Element]
TemplateDef = var_Element
Template = [var_Element.Selected = True]
endwith
var_Elements.Add("Node <b>3",64,-64)
oSurface.Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2),"L1")
oSurface.EndUpdate()
? "Select an element, and then click outside to select nothing"
|
176
|
exSelectObject, the user clicks the object to get it selected. The AllowSelectObject property specifies the keys combination to allow user to select the object
/*
with (this.EXSURFACEACTIVEXCONTROL1.nativeObject)
LayoutEndChanging = class::nativeObject_LayoutEndChanging
endwith
*/
// Notifies your application once the control's layout has been changed.
function nativeObject_LayoutEndChanging(Operation)
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
? "LayoutEndChanging"
? Str(oSurface.FormatABC("value = 5 ? `exSelectObject` : value",Operation))
return
/*
with (this.EXSURFACEACTIVEXCONTROL1.nativeObject)
LayoutStartChanging = class::nativeObject_LayoutStartChanging
endwith
*/
// Occurs when the control's layout is about to be changed.
function nativeObject_LayoutStartChanging(Operation)
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
? "LayoutStartChanging"
? Str(oSurface.FormatABC("value = 5 ? `exSelectObject` : value",Operation))
return
local oSurface,var_Elements
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.AllowLinkControlPoint = 99 /*exAllowChangeTo | exAllowChangeFrom | exEndControlPoint | exStartControlPoint*/
var_Elements = oSurface.Elements
var_Elements.Add("Node <b>1",-64,-64)
var_Elements.Add("Node <b>2")
var_Elements.Add("Node <b>3",64,-64)
oSurface.Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2),"L1")
oSurface.EndUpdate()
? "Hold ALT and click, then drag to select elements within the drawn rectangle. Click an element to select it. CTRL + CLick to unselect it"
|
175
|
exMoveObject, the user moves the object. The AllowMoveObject property specifies the keys combination to allow user to move the object
/*
with (this.EXSURFACEACTIVEXCONTROL1.nativeObject)
LayoutEndChanging = class::nativeObject_LayoutEndChanging
endwith
*/
// Notifies your application once the control's layout has been changed.
function nativeObject_LayoutEndChanging(Operation)
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
? "LayoutEndChanging"
? Str(oSurface.FormatABC("value = 4 ? `exMoveObject` : value",Operation))
return
/*
with (this.EXSURFACEACTIVEXCONTROL1.nativeObject)
LayoutStartChanging = class::nativeObject_LayoutStartChanging
endwith
*/
// Occurs when the control's layout is about to be changed.
function nativeObject_LayoutStartChanging(Operation)
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
? "LayoutStartChanging"
? Str(oSurface.FormatABC("value = 4 ? `exMoveObject` : value",Operation))
return
local oSurface,var_Elements
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.AllowLinkControlPoint = 99 /*exAllowChangeTo | exAllowChangeFrom | exEndControlPoint | exStartControlPoint*/
var_Elements = oSurface.Elements
var_Elements.Add("Node <b>1",-64,-64)
var_Elements.Add("Node <b>2")
var_Elements.Add("Node <b>3",64,-64)
oSurface.Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2),"L1")
oSurface.EndUpdate()
? "Move an element"
|
174
|
exResizeObject, the user resizes the object. The AllowResizeObject property specifies the keys combination to allow user to resize the object
/*
with (this.EXSURFACEACTIVEXCONTROL1.nativeObject)
LayoutEndChanging = class::nativeObject_LayoutEndChanging
endwith
*/
// Notifies your application once the control's layout has been changed.
function nativeObject_LayoutEndChanging(Operation)
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
? "LayoutEndChanging"
? Str(oSurface.FormatABC("value = 3 ? `exResizeObject` : value",Operation))
return
/*
with (this.EXSURFACEACTIVEXCONTROL1.nativeObject)
LayoutStartChanging = class::nativeObject_LayoutStartChanging
endwith
*/
// Occurs when the control's layout is about to be changed.
function nativeObject_LayoutStartChanging(Operation)
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
? "LayoutStartChanging"
? Str(oSurface.FormatABC("value = 3 ? `exResizeObject` : value",Operation))
return
local oSurface,var_Element,var_Element1,var_Element2,var_Elements
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.AllowLinkControlPoint = 99 /*exAllowChangeTo | exAllowChangeFrom | exEndControlPoint | exStartControlPoint*/
var_Elements = oSurface.Elements
// var_Elements.Add("Node <b>1",-64,-64).AutoSize = false
var_Element = var_Elements.Add("Node <b>1",-64,-64)
with (oSurface)
TemplateDef = [dim var_Element]
TemplateDef = var_Element
Template = [var_Element.AutoSize = False]
endwith
// var_Elements.Add("Node <b>2").AutoSize = false
var_Element1 = var_Elements.Add("Node <b>2")
with (oSurface)
TemplateDef = [dim var_Element1]
TemplateDef = var_Element1
Template = [var_Element1.AutoSize = False]
endwith
// var_Elements.Add("Node <b>3",64,-64).AutoSize = false
var_Element2 = var_Elements.Add("Node <b>3",64,-64)
with (oSurface)
TemplateDef = [dim var_Element2]
TemplateDef = var_Element2
Template = [var_Element2.AutoSize = False]
endwith
oSurface.Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2),"L1")
oSurface.EndUpdate()
? "Resize an element"
|
173
|
exSurfaceHome, the user clicks the Home button on the control's toolbar, so the surface is restored to original position. The Home method has the same effect
/*
with (this.EXSURFACEACTIVEXCONTROL1.nativeObject)
LayoutEndChanging = class::nativeObject_LayoutEndChanging
endwith
*/
// Notifies your application once the control's layout has been changed.
function nativeObject_LayoutEndChanging(Operation)
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
? "LayoutEndChanging"
? Str(oSurface.FormatABC("value = 2 ? `exSurfaceHome` : value",Operation))
return
/*
with (this.EXSURFACEACTIVEXCONTROL1.nativeObject)
LayoutStartChanging = class::nativeObject_LayoutStartChanging
endwith
*/
// Occurs when the control's layout is about to be changed.
function nativeObject_LayoutStartChanging(Operation)
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
? "LayoutStartChanging"
? Str(oSurface.FormatABC("value = 2 ? `exSurfaceHome` : value",Operation))
return
local oSurface,var_Elements
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.AllowLinkControlPoint = 99 /*exAllowChangeTo | exAllowChangeFrom | exEndControlPoint | exStartControlPoint*/
var_Elements = oSurface.Elements
var_Elements.Add("Node <b>1",-64,-64)
var_Elements.Add("Node <b>2")
var_Elements.Add("Node <b>3",64,-64)
oSurface.Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2),"L1")
oSurface.EndUpdate()
? "Click the Home button"
|
172
|
exSurfaceZoom, the user magnifies or shrinks the surface. The AllowZoomSurface property specifies the keys combination to allow user to zoom the surface
/*
with (this.EXSURFACEACTIVEXCONTROL1.nativeObject)
LayoutEndChanging = class::nativeObject_LayoutEndChanging
endwith
*/
// Notifies your application once the control's layout has been changed.
function nativeObject_LayoutEndChanging(Operation)
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
? "LayoutEndChanging"
? Str(oSurface.FormatABC("value = 1 ? `exSurfaceZoom` : value",Operation))
return
/*
with (this.EXSURFACEACTIVEXCONTROL1.nativeObject)
LayoutStartChanging = class::nativeObject_LayoutStartChanging
endwith
*/
// Occurs when the control's layout is about to be changed.
function nativeObject_LayoutStartChanging(Operation)
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
? "LayoutStartChanging"
? Str(oSurface.FormatABC("value = 1 ? `exSurfaceZoom` : value",Operation))
return
local oSurface,var_Elements
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.AllowLinkControlPoint = 99 /*exAllowChangeTo | exAllowChangeFrom | exEndControlPoint | exStartControlPoint*/
var_Elements = oSurface.Elements
var_Elements.Add("Node <b>1",-64,-64)
var_Elements.Add("Node <b>2")
var_Elements.Add("Node <b>3",64,-64)
oSurface.Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2),"L1")
oSurface.EndUpdate()
? "Click and drag the surface to reposition it"
|
171
|
exSurfaceMove, the user scrolls or moves the surface. The AllowMoveSurface property specifies the keys combination to allow user to move / scroll the surface
/*
with (this.EXSURFACEACTIVEXCONTROL1.nativeObject)
LayoutEndChanging = class::nativeObject_LayoutEndChanging
endwith
*/
// Notifies your application once the control's layout has been changed.
function nativeObject_LayoutEndChanging(Operation)
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
? "LayoutEndChanging"
? Str(oSurface.FormatABC("value = 0 ? `exSurfaceMove` : value",Operation))
return
/*
with (this.EXSURFACEACTIVEXCONTROL1.nativeObject)
LayoutStartChanging = class::nativeObject_LayoutStartChanging
endwith
*/
// Occurs when the control's layout is about to be changed.
function nativeObject_LayoutStartChanging(Operation)
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
? "LayoutStartChanging"
? Str(oSurface.FormatABC("value = 0 ? `exSurfaceMove` : value",Operation))
return
local oSurface,var_Elements
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.AllowLinkControlPoint = 99 /*exAllowChangeTo | exAllowChangeFrom | exEndControlPoint | exStartControlPoint*/
var_Elements = oSurface.Elements
var_Elements.Add("Node <b>1",-64,-64)
var_Elements.Add("Node <b>2")
var_Elements.Add("Node <b>3",64,-64)
oSurface.Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2),"L1")
oSurface.EndUpdate()
? "Click and drag the surface to reposition it"
|
170
|
FormatABC method formats the A,B,C values based on the giving expression and returns the result

local oSurface
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
? Str(oSurface.FormatABC("value format ``",1000))
|
169
|
FreezeEvents(Freeze) method prevents firing any event. For instance, FreezeEvents(True) freezes the control's events, no no event is fired, until the FreezeEvents(False) is called
/*
with (this.EXSURFACEACTIVEXCONTROL1.nativeObject)
Event = class::nativeObject_Event
endwith
*/
// Notifies the application once the control fires an event.
function nativeObject_Event(EventID)
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
? Str(oSurface.EventParam(-2))
return
local oSurface,var_Elements
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.FreezeEvents(true)
? "No event is fired after FreezeEvents(True) call"
oSurface.BeginUpdate()
oSurface.AllowLinkControlPoint = 3 /*exEndControlPoint | exStartControlPoint*/
var_Elements = oSurface.Elements
var_Elements.Add("Node <b>1",-64,-64)
var_Elements.Add("Node <b>2")
oSurface.Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2),"L1")
oSurface.EndUpdate()
|
168
|
The exAllowChangeFrom(0x20)/exAllowChangeTo(0x40) flag of LinkControlPointEnum type allows the user to adjust the link's from/to element by dragging and dropping the start control point (requires the exStartControlPoint/exEndControlPoint flag)
/*
with (this.EXSURFACEACTIVEXCONTROL1.nativeObject)
LayoutEndChanging = class::nativeObject_LayoutEndChanging
endwith
*/
// Notifies your application once the control's layout has been changed.
function nativeObject_LayoutEndChanging(Operation)
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
? "LayoutEndChanging"
? Str(Operation)
? Str(oSurface.FocusLink.ID)
return
/*
with (this.EXSURFACEACTIVEXCONTROL1.nativeObject)
LayoutStartChanging = class::nativeObject_LayoutStartChanging
endwith
*/
// Occurs when the control's layout is about to be changed.
function nativeObject_LayoutStartChanging(Operation)
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
? "LayoutStartChanging"
? Str(Operation)
? Str(oSurface.FocusLink.ID)
return
local oSurface,var_Elements
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.AllowLinkControlPoint = 99 /*exAllowChangeTo | exAllowChangeFrom | exEndControlPoint | exStartControlPoint*/
var_Elements = oSurface.Elements
var_Elements.Add("Node <b>1",-64,-64)
var_Elements.Add("Node <b>2")
var_Elements.Add("Node <b>3",64,-64)
oSurface.Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2),"L1")
oSurface.FocusLink = "L1"
oSurface.EndUpdate()
|
167
|
The LayoutStartChanging(exFocusLink)/LayoutEndChanging(exFocusLink) event notifies your application when the user focuses on a new link
/*
with (this.EXSURFACEACTIVEXCONTROL1.nativeObject)
LayoutEndChanging = class::nativeObject_LayoutEndChanging
endwith
*/
// Notifies your application once the control's layout has been changed.
function nativeObject_LayoutEndChanging(Operation)
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
? "LayoutEndChanging"
? Str(Operation)
? Str(oSurface.FocusLink)
return
/*
with (this.EXSURFACEACTIVEXCONTROL1.nativeObject)
LayoutStartChanging = class::nativeObject_LayoutStartChanging
endwith
*/
// Occurs when the control's layout is about to be changed.
function nativeObject_LayoutStartChanging(Operation)
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
? "LayoutStartChanging"
? Str(Operation)
? Str(oSurface.FocusLink)
return
local oSurface,var_Elements
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.AllowLinkControlPoint = 3 /*exEndControlPoint | exStartControlPoint*/
var_Elements = oSurface.Elements
var_Elements.Add("Node <b>1",-64,-64)
var_Elements.Add("Node <b>2")
oSurface.Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2),"L1")
oSurface.EndUpdate()
|
166
|
Focus a link
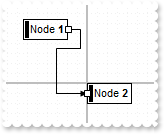
/*
with (this.EXSURFACEACTIVEXCONTROL1.nativeObject)
LayoutEndChanging = class::nativeObject_LayoutEndChanging
endwith
*/
// Notifies your application once the control's layout has been changed.
function nativeObject_LayoutEndChanging(Operation)
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
? "LayoutEndChanging"
? Str(Operation)
return
/*
with (this.EXSURFACEACTIVEXCONTROL1.nativeObject)
LayoutStartChanging = class::nativeObject_LayoutStartChanging
endwith
*/
// Occurs when the control's layout is about to be changed.
function nativeObject_LayoutStartChanging(Operation)
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
? "LayoutStartChanging"
? Str(Operation)
return
local oSurface,var_Elements
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.AllowLinkControlPoint = 3 /*exEndControlPoint | exStartControlPoint*/
var_Elements = oSurface.Elements
var_Elements.Add("Node <b>1",-64,-64)
var_Elements.Add("Node <b>2")
oSurface.Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2),"L1")
oSurface.FocusLink = "L1"
oSurface.EndUpdate()
|
165
|
The caption is displayed on the back, so the picture overrides it. How can I place the caption on the foreground
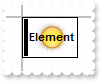
local oSurface,var_Element
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.DrawPartsOrder = "extracaption,extrapicture,picture,check,caption,client"
oSurface.Template = [HTMLPicture("pic1") = "c:\exontrol\images\sun.png"] // oSurface.HTMLPicture("pic1") = "c:\exontrol\images\sun.png"
var_Element = oSurface.Elements.Add("<b>Element")
var_Element.PicturesAlign = 17
var_Element.CaptionAlign = 17
var_Element.Pictures = "pic1"
oSurface.EndUpdate()
|
164
|
Draws a frame arround the link's arrow
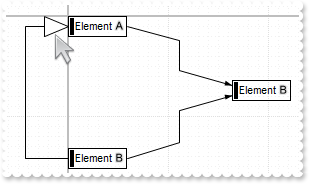
local oSurface,var_Elements,var_Link,var_Link1,var_Link2,var_Links
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.AllowLinkControlPoint = -1 /*0xffffff80 | exAllowChangeTo | exAllowChangeFrom | exOrthoArrange | exMiddleControlPoint | exControlPoint | exEndControlPoint | exStartControlPoint*/
var_Elements = oSurface.Elements
var_Elements.Add("Element <sha ;;0>A")
var_Elements.Add("Element <sha ;;0>B",164,64)
var_Elements.Add("Element <sha ;;0>B",0,132)
var_Links = oSurface.Links
// var_Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2)).CustomPath = "0.5,0.25,0.5,.75"
var_Link = var_Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2))
with (oSurface)
TemplateDef = [dim var_Link]
TemplateDef = var_Link
Template = [var_Link.CustomPath = "0.5,0.25,0.5,.75"]
endwith
// var_Links.Add(oSurface.Elements.Item(3),oSurface.Elements.Item(2)).CustomPath = "0.5,0.25,0.5,.75"
var_Link1 = var_Links.Add(oSurface.Elements.Item(3),oSurface.Elements.Item(2))
with (oSurface)
TemplateDef = [dim var_Link1]
TemplateDef = var_Link1
Template = [var_Link1.CustomPath = "0.5,0.25,0.5,.75"]
endwith
var_Link2 = var_Links.Add(oSurface.Elements.Item(3),oSurface.Elements.Item(1))
var_Link2.ShowLinkType = 3 /*exLinkStraight | exLinkDirect*/
var_Link2.StartPos = 0
var_Link2.ArrowSize = 8
var_Link2.ArrowColor = 0xffffff
var_Link2.ArrowFrameColor = 0x0
oSurface.FitToClient()
oSurface.EndUpdate()
|
163
|
Draws a frame arround the arrow for all links
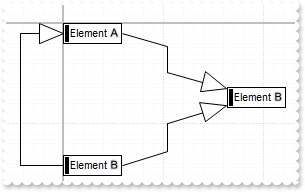
local oSurface,var_Elements,var_Link,var_Link1,var_Link2,var_Links
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.AllowLinkControlPoint = -1 /*0xffffff80 | exAllowChangeTo | exAllowChangeFrom | exOrthoArrange | exMiddleControlPoint | exControlPoint | exEndControlPoint | exStartControlPoint*/
oSurface.LinksArrowSize = 8
oSurface.LinksArrowColor = 0xffffff
oSurface.LinksArrowFrameColor = 0x0
var_Elements = oSurface.Elements
var_Elements.Add("Element <sha ;;0>A")
var_Elements.Add("Element <sha ;;0>B",164,64)
var_Elements.Add("Element <sha ;;0>B",0,132)
var_Links = oSurface.Links
// var_Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2)).CustomPath = "0.5,0.25,0.5,.75"
var_Link = var_Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2))
with (oSurface)
TemplateDef = [dim var_Link]
TemplateDef = var_Link
Template = [var_Link.CustomPath = "0.5,0.25,0.5,.75"]
endwith
// var_Links.Add(oSurface.Elements.Item(3),oSurface.Elements.Item(2)).CustomPath = "0.5,0.25,0.5,.75"
var_Link1 = var_Links.Add(oSurface.Elements.Item(3),oSurface.Elements.Item(2))
with (oSurface)
TemplateDef = [dim var_Link1]
TemplateDef = var_Link1
Template = [var_Link1.CustomPath = "0.5,0.25,0.5,.75"]
endwith
var_Link2 = var_Links.Add(oSurface.Elements.Item(3),oSurface.Elements.Item(1))
var_Link2.ShowLinkType = 3 /*exLinkStraight | exLinkDirect*/
var_Link2.StartPos = 0
oSurface.FitToClient()
oSurface.EndUpdate()
|
162
|
Change the size to display the arrow of the link
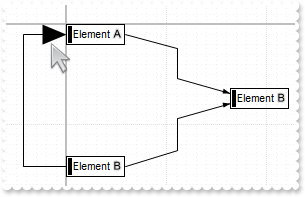
local oSurface,var_Elements,var_Link,var_Link1,var_Link2,var_Links
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.AllowLinkControlPoint = -1 /*0xffffff80 | exAllowChangeTo | exAllowChangeFrom | exOrthoArrange | exMiddleControlPoint | exControlPoint | exEndControlPoint | exStartControlPoint*/
var_Elements = oSurface.Elements
var_Elements.Add("Element <sha ;;0>A")
var_Elements.Add("Element <sha ;;0>B",164,64)
var_Elements.Add("Element <sha ;;0>B",0,132)
var_Links = oSurface.Links
// var_Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2)).CustomPath = "0.5,0.25,0.5,.75"
var_Link = var_Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2))
with (oSurface)
TemplateDef = [dim var_Link]
TemplateDef = var_Link
Template = [var_Link.CustomPath = "0.5,0.25,0.5,.75"]
endwith
// var_Links.Add(oSurface.Elements.Item(3),oSurface.Elements.Item(2)).CustomPath = "0.5,0.25,0.5,.75"
var_Link1 = var_Links.Add(oSurface.Elements.Item(3),oSurface.Elements.Item(2))
with (oSurface)
TemplateDef = [dim var_Link1]
TemplateDef = var_Link1
Template = [var_Link1.CustomPath = "0.5,0.25,0.5,.75"]
endwith
var_Link2 = var_Links.Add(oSurface.Elements.Item(3),oSurface.Elements.Item(1))
var_Link2.ShowLinkType = 3 /*exLinkStraight | exLinkDirect*/
var_Link2.StartPos = 0
var_Link2.ArrowSize = 8
oSurface.FitToClient()
oSurface.EndUpdate()
|
161
|
Change the size to display the arrows for all links
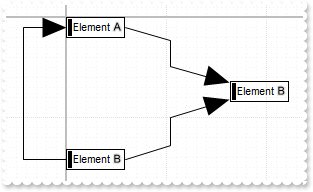
local oSurface,var_Elements,var_Link,var_Link1,var_Link2,var_Links
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.AllowLinkControlPoint = -1 /*0xffffff80 | exAllowChangeTo | exAllowChangeFrom | exOrthoArrange | exMiddleControlPoint | exControlPoint | exEndControlPoint | exStartControlPoint*/
oSurface.LinksArrowSize = 8
var_Elements = oSurface.Elements
var_Elements.Add("Element <sha ;;0>A")
var_Elements.Add("Element <sha ;;0>B",164,64)
var_Elements.Add("Element <sha ;;0>B",0,132)
var_Links = oSurface.Links
// var_Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2)).CustomPath = "0.5,0.25,0.5,.75"
var_Link = var_Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2))
with (oSurface)
TemplateDef = [dim var_Link]
TemplateDef = var_Link
Template = [var_Link.CustomPath = "0.5,0.25,0.5,.75"]
endwith
// var_Links.Add(oSurface.Elements.Item(3),oSurface.Elements.Item(2)).CustomPath = "0.5,0.25,0.5,.75"
var_Link1 = var_Links.Add(oSurface.Elements.Item(3),oSurface.Elements.Item(2))
with (oSurface)
TemplateDef = [dim var_Link1]
TemplateDef = var_Link1
Template = [var_Link1.CustomPath = "0.5,0.25,0.5,.75"]
endwith
var_Link2 = var_Links.Add(oSurface.Elements.Item(3),oSurface.Elements.Item(1))
var_Link2.ShowLinkType = 3 /*exLinkStraight | exLinkDirect*/
var_Link2.StartPos = 0
oSurface.FitToClient()
oSurface.EndUpdate()
|
160
|
Extends the caption on the element's width
local oSurface,var_Element
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
var_Element = oSurface.Elements.Add("<solidline> <c><b>Bank Account</b></solidline><br>+ owner: String <r><a 1;e64=gA8ABzABvABsABpABkg8JABuABlAAgAA4AAwisXjMYH0TAECMYAjsCMwAM4AkMGhEGOUei0Yl8bkQAOAAlsGmsSlp0h0SgkCF8DgsNhUMhEKiESkYAoMlk8phssmcCltLMNTAFOlFDlc2l0amMxjomAAjAA5AA2tMaHcfplZk1blVDqtuoNXjoAAEBA=>▲</a><br><solidline>+ balance: Currency = 0</solidline><br>+ deposit(amount: Currency)<r><a 2;e64=gA8ABjAA+AECMwAM8DABvABshoAOQAEAAHAAGEWjEajMGNoAMoAOgANERMgAOcHAAvAEJhcEh0Qh0Tg0CmkqMMFlUuhkxiMTisXjNCjk6EwAEYAHIAG1MjY7lUsnkwh8/nUClk5gwAAEBA==>▲</a><br>+ withdraw(amount: Currency)")
var_Element.ID = "Account"
var_Element.X = -128
var_Element.CaptionSingleLine = 1
var_Element.AutoSize = false
var_Element.Width = 256
var_Element.Height = var_Element.AutoHeight
var_Element.CaptionAlign = 4 /*0x4 | */
|
159
|
Gets the width/height of the element to fit its content ( as if the AutoSize property is True )
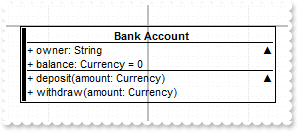
/*
with (this.EXSURFACEACTIVEXCONTROL1.nativeObject)
AnchorClick = class::nativeObject_AnchorClick
endwith
*/
// Occurs when an anchor element is clicked.
function nativeObject_AnchorClick(AnchorID,Options)
local var_Element
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
var_Element = oSurface.Elements.Item("Account")
var_Element.Height = var_Element.AutoHeight
return
local oSurface,var_Element
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
var_Element = oSurface.Elements.Add("<solidline> <c><b>Bank Account</b></solidline><br>+ owner: String <r><a 1;e64=gA8ABzABvABsABpABkg8JABuABlAAgAA4AAwisXjMYH0TAECMYAjsCMwAM4AkMGhEGOUei0Yl8bkQAOAAlsGmsSlp0h0SgkCF8DgsNhUMhEKiESkYAoMlk8phssmcCltLMNTAFOlFDlc2l0amMxjomAAjAA5AA2tMaHcfplZk1blVDqtuoNXjoAAEBA=>▲</a><br><solidline>+ balance: Currency = 0</solidline><br>+ deposit(amount: Currency)<r><a 2;e64=gA8ABjAA+AECMwAM8DABvABshoAOQAEAAHAAGEWjEajMGNoAMoAOgANERMgAOcHAAvAEJhcEh0Qh0Tg0CmkqMMFlUuhkxiMTisXjNCjk6EwAEYAHIAG1MjY7lUsnkwh8/nUClk5gwAAEBA==>▲</a><br>+ withdraw(amount: Currency)")
var_Element.ID = "Account"
var_Element.X = -128
var_Element.CaptionSingleLine = 1
var_Element.AutoSize = false
var_Element.Width = 256
var_Element.Height = var_Element.AutoHeight
var_Element.CaptionAlign = 4 /*0x4 | */
|
158
|
Expandable-caption
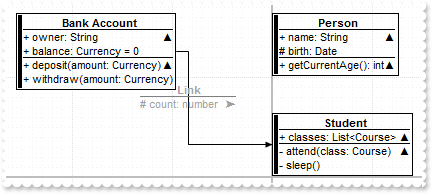
/*
with (this.EXSURFACEACTIVEXCONTROL1.nativeObject)
AnchorClick = class::nativeObject_AnchorClick
endwith
*/
// Occurs when an anchor element is clicked.
function nativeObject_AnchorClick(AnchorID,Options)
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
? Str(AnchorID)
return
local oSurface,var_Element,var_Element1,var_Element2,var_Link
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
var_Element = oSurface.Elements.Add("<solidline> <c><b>Bank Account</b></solidline><br>+ owner: String <r><a 1;e64=gA8ABzABvABsABpABkg8JABuABlAAgAA4AAwisXjMYH0TAECMYAjsCMwAM4AkMGhEGOUei0Yl8bkQAOAAlsGmsSlp0h0SgkCF8DgsNhUMhEKiESkYAoMlk8phssmcCltLMNTAFOlFDlc2l0amMxjomAAjAA5AA2tMaHcfplZk1blVDqtuoNXjoAAEBA=>▲</a><br><solidline>+ balance: Currency = 0</solidline><br>+ deposit(amount: Currency)<r><a 2;e64=gA8ABjAA+AECMwAM8DABvABshoAOQAEAAHAAGEWjEajMGNoAMoAOgANERMgAOcHAAvAEJhcEh0Qh0Tg0CmkqMMFlUuhkxiMTisXjNCjk6EwAEYAHIAG1MjY7lUsnkwh8/nUClk5gwAAEBA==>▲</a><br>+ withdraw(amount: Currency)")
var_Element.ID = "Account"
var_Element.X = -256
var_Element.Y = -164
var_Element.CaptionSingleLine = 1
var_Element.CaptionAlign = 4 /*0x4 | */
var_Element1 = oSurface.Elements.Add("<solidline> <c><b>Person</b></solidline><br>+ name: String <r><a 1;e64=gA8ABzABvABsABpABkg8JABuABlAAgAA4AAwisXjMYH0TAECMYAjsCMwAM4AkMGhEGOUei0Yl8bkQAOAAlsGmsSlp0h0SgkCF8DgsNhUMhEKiESkYAoMlk8phssmcCltLMNTAFOlFDlc2l0amMxjomAAjAA5AA2tMaHcfplZk1blVDqtuoNXjoAAEBA=>▲</a><br><solidline># birth: Date</solidline><br>+ getCurrentAge(): int<r><a 2;e64=gA8ABjAA+AECMwAM8DABvABshoAOQAEAAHAAGEWjEajMGNoAMoAOgANERMgAOcHAAvAEJhcEh0Qh0Tg0CmkqMMFlUuhkxiMTisXjNCjk6EwAEYAHIAG1MjY7lUsnkwh8/nUClk5gwAAEBA==>▲</a>")
var_Element1.ID = "Person"
var_Element1.Y = -164
var_Element1.CaptionSingleLine = 1
var_Element2 = oSurface.Elements.Add("<solidline> <c><b>Student</b></solidline><br><solidline>+ classes: List<Course> <r><a 1;e64=gA8ABzABvABsABpABkg8JABuABlAA+AAgAECMcTi4AMwAM4AjMGhEGOUVAA4AAwk8plcqihwAElg0wiUlOkOiUEgQvgcFhsKhkIhUQiUUnccj0gn0jmMagUlowAMNOpEfkMNkkmlEqrctjQmAAjAA5AA2sssHcbnkdq1Ln1QtVSjQAAEBA==>▲</a></solidline><br>- attend(class: Course)<r><a 2;e64=gA8ABjAA+AECMwAM8DABvABshoAOQAEAAHAAGEWjEajMGNoAMoAOgANERMgAOcHAAvAEJhcEh0Qh0Tg0CmkqMMFlUuhkxiMTisXjNCjk6EwAEYAHIAG1MjY7lUsnkwh8/nUClk5gwAAEBA==>▲</a><br>- sleep()")
var_Element2.ID = "Student"
var_Element2.Y = -64
var_Element2.CaptionSingleLine = 1
var_Link = oSurface.Links.Add(oSurface.Elements.Item("Account"),oSurface.Elements.Item("Student"))
var_Link.Caption = "<fgcolor A0A0A0><solidline 808080> <c><b>Link</b></solidline><br># count: number <r><a ;exp=12992>➤</a>"
|
157
|
Expandable-caption
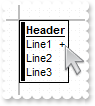
local oSurface,var_Element
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
var_Element = oSurface.Elements.Add("<solidline><b>Header</b></solidline><br>Line1<r><a ;exp=show lines>+</a><br>Line2<br>Line3")
var_Element.CaptionSingleLine = 1
|
156
|
Fullfit the caption on the element's width
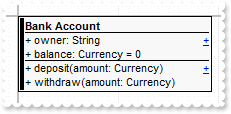
local oSurface,var_Element
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
var_Element = oSurface.Elements.Add("<solidline><b>Bank Account</b></solidline><br>+ owner: String <r><a 1;properties>+</a><br><solidline>+ balance: Currency = 0</solidline><br>+ deposit(amount: Currency)<r><a 2;methods>+</a><br>+ withdraw(amount: Currency)")
var_Element.CaptionSingleLine = 1
var_Element.BackColor = 0xf8f8f8
var_Element.AutoSize = false
var_Element.Width = 194
var_Element.Height = 76
var_Element.CaptionAlign = 4 /*0x4 | */
|
155
|
Wrap the caption by <br> or "\r\n" sequence only
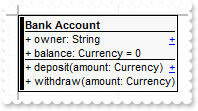
local oSurface,var_Element
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
var_Element = oSurface.Elements.Add("<solidline><b>Bank Account</b></solidline><br>+ owner: String <r><a 1;properties>+</a><br><solidline>+ balance: Currency = 0</solidline><br>+ deposit(amount: Currency)<r><a 2;methods>+</a><br>+ withdraw(amount: Currency)")
var_Element.CaptionSingleLine = 1
var_Element.BackColor = 0xf8f8f8
|
154
|
Display a custom tooltip
/*
with (this.EXSURFACEACTIVEXCONTROL1.nativeObject)
MouseMove = class::nativeObject_MouseMove
endwith
*/
// Occurs when the user moves the mouse.
function nativeObject_MouseMove(Button,Shift,X,Y)
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.ShowToolTip("new content","",null,"+8","+8")
return
local oSurface
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
|
153
|
Shows the tooltip of the object moved relative to its default position
/*
with (this.EXSURFACEACTIVEXCONTROL1.nativeObject)
MouseMove = class::nativeObject_MouseMove
endwith
*/
// Occurs when the user moves the mouse.
function nativeObject_MouseMove(Button,Shift,X,Y)
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.ShowToolTip("<null>","<null>",null,"+8","+8")
return
local oSurface,var_Element
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
// oSurface.Elements.Add("Element with a Tooltip").ToolTip = "This is a bit of text that should be displayed when cursor hovers the element."
var_Element = oSurface.Elements.Add("Element with a Tooltip")
with (oSurface)
TemplateDef = [dim var_Element]
TemplateDef = var_Element
Template = [var_Element.ToolTip = "This is a bit of text that should be displayed when cursor hovers the element."]
endwith
|
152
|
Rename Undo/Redo commands into the control's toolbar
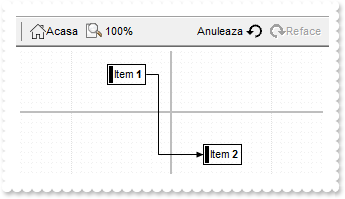
local oSurface,var_Elements
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.AllowUndoRedo = true
oSurface.ToolBarFormat = "-1,100,101,|,103,104"
oSurface.Template = [ToolBarCaption(100) = "<img>1</img>Acasa"] // oSurface.ToolBarCaption(100) = "<img>1</img>Acasa"
oSurface.Template = [ToolBarCaption(103) = "Anuleaza <img>3</img>"] // oSurface.ToolBarCaption(103) = "Anuleaza <img>3</img>"
oSurface.Template = [ToolBarCaption(104) = "<img>4</img>Reface"] // oSurface.ToolBarCaption(104) = "<img>4</img>Reface"
oSurface.Template = [ToolBarToolTip(100) = "Restabileste vizualizarea la origine."] // oSurface.ToolBarToolTip(100) = "Restabileste vizualizarea la origine."
oSurface.Template = [ToolBarToolTip(101) = "Mareste vizualizarea."] // oSurface.ToolBarToolTip(101) = "Mareste vizualizarea."
oSurface.Template = [ToolBarToolTip(103) = "Anuleaza ultima actiune UI. Pentru a anula o actiune apasati Ctrl+Z."] // oSurface.ToolBarToolTip(103) = "Anuleaza ultima actiune UI. Pentru a anula o actiune apasati Ctrl+Z."
oSurface.Template = [ToolBarToolTip(104) = "Inverseaza cea mai recenta operatie de anulare. Pentru a reface o actiune apasati Ctrl+Y."] // oSurface.ToolBarToolTip(104) = "Inverseaza cea mai recenta operatie de anulare. Pentru a reface o actiune apasati Ctrl+Y."
var_Elements = oSurface.Elements
var_Elements.Add("Item <b>1",-64,-48)
var_Elements.Add("Item <b>2",32,32)
oSurface.Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2))
oSurface.EndUpdate()
|
151
|
Add Undo/Redo commands to control's toolbar
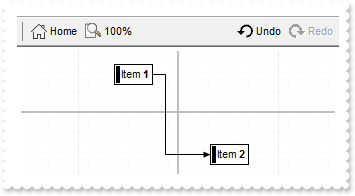
local oSurface,var_Elements
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.AllowUndoRedo = true
oSurface.ToolBarFormat = "-1,100,101,|,103,104"
var_Elements = oSurface.Elements
var_Elements.Add("Item <b>1",-64,-48)
var_Elements.Add("Item <b>2",32,32)
oSurface.Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2))
oSurface.EndUpdate()
|
150
|
Clear Undo/Redo queue (method 2)
local c,oSurface,var_Elements
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.AllowUndoRedo = true
var_Elements = oSurface.Elements
var_Elements.Add("Item <b>1",-64,-64)
var_Elements.Add("Item <b>2")
oSurface.Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2))
c = oSurface.UndoRedoQueueLength
oSurface.UndoRedoQueueLength = 0
oSurface.UndoRedoQueueLength = c
? oSurface.UndoListAction()
oSurface.EndUpdate()
|
149
|
Clear Undo/Redo queue (method 1)
local oSurface,var_Elements
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.AllowUndoRedo = true
var_Elements = oSurface.Elements
var_Elements.Add("Item <b>1",-64,-64)
var_Elements.Add("Item <b>2")
oSurface.Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2))
oSurface.AllowUndoRedo = true
? oSurface.UndoListAction()
oSurface.EndUpdate()
|
148
|
Removes Redo operations
local oSurface,var_Elements
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.AllowUndoRedo = true
var_Elements = oSurface.Elements
var_Elements.Add("Item <b>1",-64,-64)
var_Elements.Add("Item <b>2")
oSurface.Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2))
oSurface.Undo()
oSurface.RedoRemoveAction(10)
? oSurface.RedoListAction()
oSurface.EndUpdate()
|
147
|
Removes Undo operations
local oSurface,var_Elements
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.AllowUndoRedo = true
var_Elements = oSurface.Elements
var_Elements.Add("Item <b>1",-64,-64)
var_Elements.Add("Item <b>2")
oSurface.Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2))
oSurface.UndoRemoveAction(10)
? oSurface.UndoListAction()
oSurface.EndUpdate()
|
146
|
Record the UI operations as a block of undo/redo operations
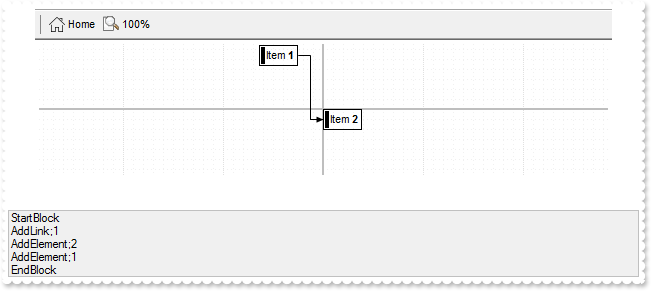
local oSurface,var_Elements
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.AllowUndoRedo = true
oSurface.StartBlockUndoRedo()
var_Elements = oSurface.Elements
var_Elements.Add("Item <b>1",-64,-64)
var_Elements.Add("Item <b>2")
oSurface.Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2))
oSurface.EndBlockUndoRedo()
? oSurface.UndoListAction()
oSurface.EndUpdate()
|
145
|
Groups the next to current Undo/Redo Actions in a single block
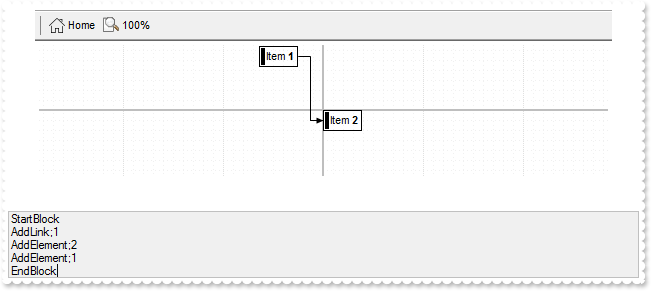
local oSurface,var_Elements
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.AllowUndoRedo = true
var_Elements = oSurface.Elements
var_Elements.Add("Item <b>1",-64,-64)
var_Elements.Add("Item <b>2")
oSurface.Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2))
oSurface.GroupUndoRedoActions(3)
? oSurface.UndoListAction()
oSurface.EndUpdate()
|
144
|
Limits the number of entries within the Undo/Redo queue
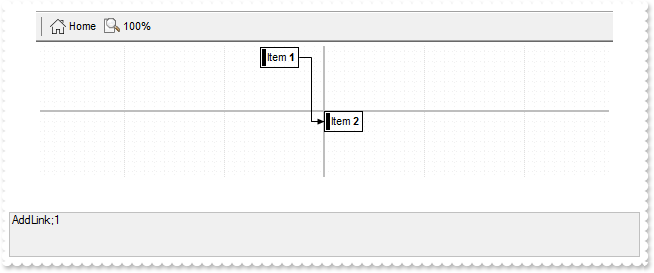
local oSurface,var_Elements
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.AllowUndoRedo = true
oSurface.UndoRedoQueueLength = 1
var_Elements = oSurface.Elements
var_Elements.Add("Item <b>1",-64,-64)
var_Elements.Add("Item <b>2")
oSurface.Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2))
? oSurface.UndoListAction()
oSurface.EndUpdate()
|
143
|
Lists the Redo actions that can be performed on the surface
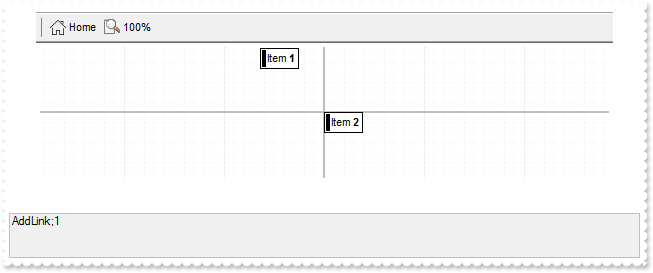
local oSurface,var_Elements
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.AllowUndoRedo = true
var_Elements = oSurface.Elements
var_Elements.Add("Item <b>1",-64,-64)
var_Elements.Add("Item <b>2")
oSurface.Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2))
oSurface.Undo()
? oSurface.RedoListAction()
oSurface.EndUpdate()
|
142
|
Lists the Undo actions that can be performed on the surface
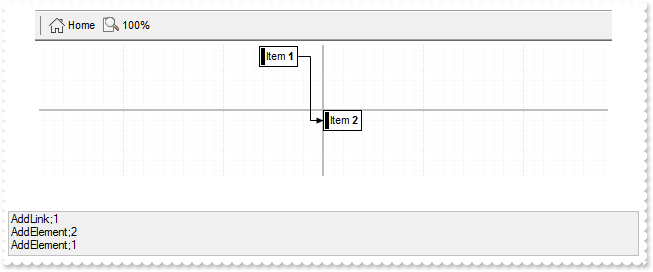
local oSurface,var_Elements
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.AllowUndoRedo = true
var_Elements = oSurface.Elements
var_Elements.Add("Item <b>1",-64,-64)
var_Elements.Add("Item <b>2")
oSurface.Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2))
? oSurface.UndoListAction()
oSurface.EndUpdate()
|
141
|
Checks whether the Undo operation is possible
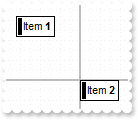
local oSurface,var_Elements
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.AllowUndoRedo = true
var_Elements = oSurface.Elements
var_Elements.Add("Item <b>1",-64,-64)
var_Elements.Add("Item <b>2")
oSurface.Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2))
oSurface.Undo()
? "CanRedo"
? Str(oSurface.CanRedo)
oSurface.EndUpdate()
|
140
|
Call Redo by code
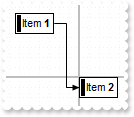
local oSurface,var_Elements
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.AllowUndoRedo = true
var_Elements = oSurface.Elements
var_Elements.Add("Item <b>1",-64,-64)
var_Elements.Add("Item <b>2")
oSurface.Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2))
oSurface.Undo()
oSurface.Redo()
oSurface.EndUpdate()
|
139
|
Checks whether the Undo operation is possible
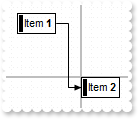
local oSurface,var_Elements
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.AllowUndoRedo = true
var_Elements = oSurface.Elements
var_Elements.Add("Item <b>1",-64,-64)
var_Elements.Add("Item <b>2")
oSurface.Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2))
? "CanUndo"
? Str(oSurface.CanUndo)
oSurface.EndUpdate()
|
138
|
Call Undo by code
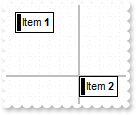
local oSurface,var_Elements
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.AllowUndoRedo = true
var_Elements = oSurface.Elements
var_Elements.Add("Item <b>1",-64,-64)
var_Elements.Add("Item <b>2")
oSurface.Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2))
oSurface.Undo()
oSurface.EndUpdate()
|
137
|
Save the element's properties for Undo/Redo operations, by code
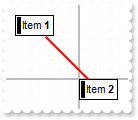
local h,oSurface,var_Elements,var_Link
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.AllowUndoRedo = true
var_Elements = oSurface.Elements
var_Elements.Add("Item <b>1",-64,-64)
var_Elements.Add("Item <b>2")
oSurface.StartBlockUndoRedo()
var_Link = oSurface.Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2))
h = var_Link.StartUpdateLink
var_Link.Color = 0xff
var_Link.Width = 2
var_Link.ShowDir = false
var_Link.ShowLinkType = 2
var_Link.EndUpdateLink(h)
oSurface.EndBlockUndoRedo()
oSurface.EndUpdate()
|
136
|
No color is restored for the link when Undo/Redo operation is executed
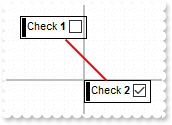
/*
with (this.EXSURFACEACTIVEXCONTROL1.nativeObject)
AddElement = class::nativeObject_AddElement
endwith
*/
// A new element has been added to the surface.
function nativeObject_AddElement(Element)
/* Element.ShowCheckBox = True */
/* Element.CheckBoxAlign = 2 */
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
return
local h,oSurface,var_Element,var_Elements,var_Link
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.AllowUndoRedo = true
var_Elements = oSurface.Elements
var_Elements.Add("Check <b>1",-64,-64)
// var_Elements.Add("Check <b>2").Checked = 1
var_Element = var_Elements.Add("Check <b>2")
with (oSurface)
TemplateDef = [dim var_Element]
TemplateDef = var_Element
Template = [var_Element.Checked = 1]
endwith
oSurface.StartBlockUndoRedo()
var_Link = oSurface.Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2))
h = var_Link.StartUpdateLink
var_Link.Color = 0xff
var_Link.Width = 2
var_Link.ShowDir = false
var_Link.ShowLinkType = 2
var_Link.EndUpdateLink(h)
oSurface.EndBlockUndoRedo()
oSurface.EndUpdate()
|
135
|
Save the element's properties for Undo/Redo operations, by code
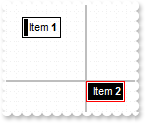
local h,oSurface,var_Element
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.AllowUndoRedo = true
oSurface.Elements.Add("Item <b>1",-64,-64)
oSurface.StartBlockUndoRedo()
var_Element = oSurface.Elements.Add("Item <b>2")
h = var_Element.StartUpdateElement
var_Element.BackColor = 0x0
var_Element.ForeColor = 0xffffff
var_Element.BorderColor = 0xff
var_Element.EndUpdateElement(h)
oSurface.EndBlockUndoRedo()
oSurface.EndUpdate()
|
134
|
No color is restored for the element when Undo/Redo operation is executed
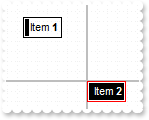
local h,oSurface,var_Element
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.AllowUndoRedo = true
oSurface.Elements.Add("Item <b>1",-64,-64)
oSurface.StartBlockUndoRedo()
var_Element = oSurface.Elements.Add("Item <b>2")
h = var_Element.StartUpdateElement
var_Element.BackColor = 0x0
var_Element.ForeColor = 0xffffff
var_Element.BorderColor = 0xff
var_Element.EndUpdateElement(h)
oSurface.EndBlockUndoRedo()
oSurface.EndUpdate()
|
133
|
How can I ensure that a specified element fits the surface's visible area
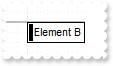
local oSurface,var_Elements,var_Pattern
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
var_Elements = oSurface.Elements
var_Pattern = var_Elements.Add("Element A",-100).Pattern
var_Pattern.Type = 6
var_Pattern.Color = 0xe0e0e0
var_Elements.Add("Element B",2000).EnsureVisible()
|
132
|
LayoutEndChanging(exUndo), LayoutEndChanging(exRedo) or LayoutEndChanging(exUndoRedoUpdate) notifiy your application once a Undo/Redo operation is executed (CTRL+Z, CTRL+Y) or updated
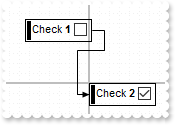
/*
with (this.EXSURFACEACTIVEXCONTROL1.nativeObject)
AddElement = class::nativeObject_AddElement
endwith
*/
// A new element has been added to the surface.
function nativeObject_AddElement(Element)
/* Element.ShowCheckBox = True */
/* Element.CheckBoxAlign = 2 */
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
return
/*
with (this.EXSURFACEACTIVEXCONTROL1.nativeObject)
LayoutEndChanging = class::nativeObject_LayoutEndChanging
endwith
*/
// Notifies your application once the control's layout has been changed.
function nativeObject_LayoutEndChanging(Operation)
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
? "LayoutEndChanging"
? Str(Operation)
return
local oSurface,var_Element,var_Elements
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.AllowUndoRedo = true
var_Elements = oSurface.Elements
var_Elements.Add("Check <b>1",-64,-64)
// var_Elements.Add("Check <b>2").Checked = 1
var_Element = var_Elements.Add("Check <b>2")
with (oSurface)
TemplateDef = [dim var_Element]
TemplateDef = var_Element
Template = [var_Element.Checked = 1]
endwith
oSurface.Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2))
oSurface.EndUpdate()
|
131
|
Turn on the Undo/Redo feature
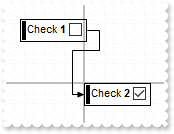
/*
with (this.EXSURFACEACTIVEXCONTROL1.nativeObject)
AddElement = class::nativeObject_AddElement
endwith
*/
// A new element has been added to the surface.
function nativeObject_AddElement(Element)
/* Element.ShowCheckBox = True */
/* Element.CheckBoxAlign = 2 */
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
return
local oSurface,var_Element,var_Elements
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.AllowUndoRedo = true
var_Elements = oSurface.Elements
var_Elements.Add("Check <b>1",-64,-64)
// var_Elements.Add("Check <b>2").Checked = 1
var_Element = var_Elements.Add("Check <b>2")
with (oSurface)
TemplateDef = [dim var_Element]
TemplateDef = var_Element
Template = [var_Element.Checked = 1]
endwith
oSurface.Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2))
oSurface.EndUpdate()
|
130
|
ImageSize property on 32 (specifies the size of control' icons/images/check-boxes/radio-buttons)
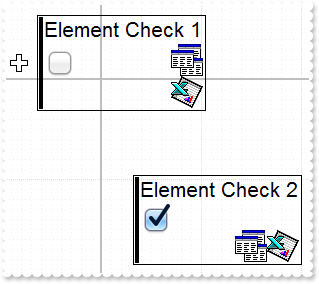
/*
with (this.EXSURFACEACTIVEXCONTROL1.nativeObject)
AddElement = class::nativeObject_AddElement
endwith
*/
// A new element has been added to the surface.
function nativeObject_AddElement(Element)
/* Element.ShowCheckBox = True */
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
return
local oSurface,var_Appearance,var_Element,var_Element1,var_Element2,var_Elements
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.ImageSize = 32
oSurface.Font.Size = 16
oSurface.Images("gBJJgBAIDAAEg4AEEKAD/hz/EMNh8TIRNGwAjEZAEXjAojKAjMLjABhkaABAk0plUrlktl0vmExmUzmk1m03nE5nU7nk9miAoE+oVDolFo1HpFJpU5h8Sf9OqFNqUOqNUqdPq9VrFWrlbr1QpdhAFAkFis1ntFptVrtkrpszrNvmVxqk3uVtm1kmF3sdBvF/wGBmV+j9BYGHwWJulfxdax2NyFdx2JlV6l9Nw7AAGZymdz2Cy2GxErvWcz9ivlwyV21cuxugwktzGIzmvwtl0+53U5y0a0Wazmmyu/3dCyOMyXHx/J5nIr9q3uyqnBxFN3G46ma4vb7mD2Ng4nZze00fDkHC7t7us2rOX5tguetpHRlmz4HVqnXk1PjHO+CMPo9MBMC+j2vC8j7wS8cFNI4kBo05UIvfCT/NsnsApU+0Fqg/T+oy/kPxC0sEQfErKQK96+w28UWRI8UGvO8sTLS9r2PWmsMJTDTask3CsIbIEQRA3shOXEEAO/GclJ9FEKrrA8FRbKMXRIlb0JxCkjS1LMswhCcvuel0cv26cSMa8Ufx+2sQwhEUoSXOCjSbLcnxjKc7sdKUVyq28NtVI71P9P7JxtQEapjQ6fzfM8zPfNE2PhIsLL63E40slk5y7N89LcyU9SvMb3SdUc6VJLj5VLVLfO/PS9KzNFHUa/0XyBD0dxlS9cxhMlTRSoNXypPErWDPyfNS+MwprRNO0FD8wVVZ1AI08URwVRjtJ1WCn21QkkUrXVLVPQS/XIk" ;
+"FgTxT9iONZ9xVTdq+L1eKg3kkF6Upe68XtfV51/MtrVjBlwYFL1ev8y1/P6/lyzzYl02wntj0RVFmS1Qa+M5as93QxEUW9e993rfmQ2+vy65M/mL1lhl/2bj2ByVduMtNhCJT9hdz41nN14Ld12Z9UjfI/oUAaGseiw6+uFLLhcVabJOS5RqOE0BHlZ5VnEr5fOMs3st+aa/bbRzrJGV51Y0b0DbqaWXZD90hIsPbjWu52+6Wyadpe66hhO+P/XioW5rD8ZbrUZuVg6n1dsE/cXmewu1m9PVwnd35/nueXho/NaJzmjc61W76esuT77eG8pTquy9TwWH8LEzG8RDfFalx3Gcfvna9rvG/cptGLd9tuI6TZOP5Fiqi99vea+X4VRcBq/JZZtVQ9cwSs5lsXE372+a9z7PbfB3VVqHyvMctLto8uob6eV0m/cD6MN2v+T33t6sBut42vdv2bJ8a997x2maFJfK+qArbGJPEKE+1qTflMsIdW/GCJX17KcT6/czr/X+u1g29B7j/4BQfWkkx4zIHisjhPCmE0K4SwtXM+d4BvHRwNZOoBph9IJvPek9d40FoMJxf691jj2ywQQcHEWET4XJwkTszlVqm2GokewxtBT1DpQjRxDN0rUVDNKdC3lb6tzNOwh6upMSSYfv4YBCl/bsn9PxiFCEo7SI6Obc9HeOrnY8x4jtHtdpN4GRbaorhsbu18Pph5CiHymI0RpSXGJ/z2oUOxYxG858AyiI+bfJtuTcG5yelBJy" &
+"T8okhqFd4a5yxL0rvulYtKCsZiWxWkc1s1cRoxxwhA31DLE0mR9l9HqX8fJgTDmFMVH0MIsRzVYnwnMi1dyzmhLt2kS2pxIiU62Wj5ptQGlSYFakLonTUJNLKaM5WzlffEkuFkk5wTrhVO2eE7G6lJhxFFYUZ55zmn0WuBCD4pzhirFCKkbomsOoIYmZx5p90LoYWGPdD5g0QmJRKYxbZ6zYoVQ2jVGylSak7KSkFH6RSjpHKFuU+YMyNo5SulkC6I0vonTCitMXPoEpVS2H5FQfEqp2R1opIgAEkJISYARTCukOhmPNI5Ex/wzGHUsicMwA1LHgQ90Y/KpoQHAD+pB/R4NzIaMAB9Xaw1gqaAOsh/A/ptIkWUfhGK1kZH8RgH5GqvgArqRmt4AAPrTroRofBGADkqr6Rmu4D7CEaHARiwpJrEEZsXXwlVjyMWRsaRqwdkLGNBABZmytmyMnaINZqyVpLR2ftKAAAdd6h2osbaskdiq4EZtgSmyNcbVWRJNXe3AA7REar3b0stlAAXBtoRmvJGLjEYAHUWsFcwCD/rnaop9aEICMAPdK5hT6xpeuzdOtAgKuJeGfdq6ggEbkTvAP+p9UCHXrvKkcgIA==")
var_Appearance = oSurface.VisualAppearance
var_Appearance.Add(1,"gBFLBCJwBAEHhEJAAEhABfICg6AADACAxRDgMQBQKAAzQFAYbhkGCGAAGMZxRgmFgAQhFcZQSKUOQTDKMIziaQAGgkNQwCSLIwjNIsBxPFKVQChEYxSjKA40SJNUgyj6CCY+QLIE5PfQgAL9I6eJABCCSQKkYx0HScRiwPBIbAZAYhCZqaKhWgkKI/WBQIABRDVLx5ESiLRtKy7Mq2bpvXBcNxXHalaztO68LxvKyqHb5fJ/PpgL4YHgmC4NQ7EMRwF6rfbyfZ7Xg/ORPTijZ4sdzMHTzJyscx3HqfaBoOaZU5eMLceTUMofHIndxCcasPbsOatVqjG5sYjcGC3La9cz3Pq/bpuDCbMxuaK1TrYXr1TTrcofBDldAxXRKDxRDWVhLnYOw9i6XxzjuXprCaOoKB6EwbiCZZCGOdZYlcT4xHmbhMnwNxtn+G5bmqdZ7n4Pw/i+X5zm+dQ9g4CAFjsfAJheOI8HsDoWDWTB/lwSAQkmA5PEgRYoDyDwYFYFoFmGCBmBqBphDgRJ0gOTIYBGRB/lyRh0iSCZbjYWJzgWDwIjYLoLmMCJGDKDJjBgWgqG6YhyhGHRzA2aJ1mCABOAiOJvhCZBJBYRoRmSCQmEqEQimkAZgg8TZnDCV4UkmCUmBKZYJGYWoWCUUhiFMNZckNUh2GENoaGaGZmgmJhqhqZpGGIEx2GYIxSGGGJdggWJth2Z4JmYeoemeSZ2H6H4hGmQhihyTRHGYLg7CiCgmgqIpokoNoOiOaJ4jqA" ;
+"ochqaZGgaCxpAoZoaiaaJqEmWIcGgShcnCJwqEqFoR3YOoFlgchflqNouiuawHmWSYqGkWZQhcatzmaOoumuSp2j6L5bBaKo0GQKRnGGCxqiyCwmkqMpsksNpOGUGI7A0ew1G0Rxlg0PptgsZuDG2Sx2l6N5tnYNZZjUDRXDCVo5l2FoymqOpukuNpujubwLjmWY5k0ZwxkaFxYlWdp6j6b5Lnafo/nABQdg2FxcUsY5BkmXAkmeQpckwNRrkKTh8CSHZBk4NwyC4KxxgMDwakOMZDn8GgwnGAo2C4cwthMcwmCcMoHBMHRehwTIghySYNksZwcH4HBMEsHx5hyPItiweYxnwSZEH4Mozn0fR+DMAo7EYJ50gkdZelKdNql2UgJn0GIukwH4HicQRai2GI4mSVpNl0dZGledgNgcYpYDWUx3FsOQi5YV5anaTY3G6W53A2RxylydxFjiaxEFCCgBBAQ==")
var_Appearance.Add(2,"gBFLBCJwBAEHhEJAAEhABcoFg6AADACAxRDgMQBQKAAzQFAYbhkGCGAAGMZxRgmFgAQhFcZQSKUOQTDKMIziaQAGgkNQwCSLIwjNIsBxPFKVQChEYxSjKA40SJNUgyj6CCY+QLIE5PfQgAL9I6eJABCCSQKkYx0HScRiwPBIbAZAYhCZqaKhWgkKI/WBQIABRDVLx5ESiLRtKy7Mq2bpvXBcNxXHalaztO68LxvKyqHb5fJ/PpgL4YHgmC4NQ7EMRwF6rfbyfZ7Xg/ORPTijZ4sdzMHTzJyscx3HqfaBoOaZU5eMLceTUMofHIndxCcasPbsLpOS5LNKsaxmWLYdhFdTxQi6LpvfA8BwXC6JY7heRYRbFbYxRjGNi1TS7G4nGKd5WGuL4UHwI4VkaYxii8V4pgQMgVBQdQ5iCTYGi8T4vlWbJ3nuPg+l+H5AlSCg6ByPBoE8Ap3jqYxhBido5g0OgOGOGI4CsSpCCAcgcAuEokiEN5NCKfJ9DyTRjnScg1CEYxOBmBpPCgagdgcIZoHoGIFA4AxQkCAxKAgKBwgGSpIBCZhjF2E5UnQPQMiMCJBCIBwxkSQgsgo+JtDKT4ziiQw+k6EwAnsOgLnkHI+yCQ4iEuE4klkPhShEJBpAoPgymOMoaDgHBjFMBgyD0HYTiCZSZhIIIGC4ChiHSew5kwM5omILZPiOBI0hwZw5kodIdA+M4Uj4PxOmMSJ9DuTQzmyZgviceZagaHVfj4awwmaAh2GUIYmCOEZZDaD" ;
+"RDFGdwcg4EwyHMN4LBOaJbCoaZqgKH8qkMfIyD8DozDyfA7A0Coui0OpMmOZJdCsahKg6NooioChwmEMxLEoXJbDUTRXGSUgykyMgQG0GpPHMdI3D4TRCgSeQ0kmaw+lGNAtCOZJVCiT5DhyRQwAqMg0EoDBBGEGAsASC5yiSCw+k4Mp6lWNQuksTpRjMTxDGzJwGmGMpDDKXYTECSAxl6Q5olkK4PgMMIVkASRMBMBgzEkaZEjsNALhIZA6AeQBgk0ZJEgAAJ0CIAgODMNIsD6DRih9uYwFyAwfCUb5ijmbI+gwdxkk8MZMGeMpPCkDxzBiC5MHMPJLDSSROFMLIoBEQogEMFJPnENYQGgE4DCOaJfC7tYkhGTQ0kyWwykuXpMiyRpKjKR4wngM4JmOWJACCdYtHMWw+Eych4nINYLAEYA8AgdAEEsQZajaQoog4GxPiMVIolcdxNG8XZVkmNoRwWRVBlFeFEeAZQJgnFiHgHwcAhjhHgGMSI5xki2CyA4EQsA3i0HkBsLwKRFgAHcPkHopBJBcBeDUYI7xyDOHqKkWo2hLCsDIBIY4qQ5A8DoMMYwOAqCSBGKgU4yB2iDBwIgB4hxQgAAWNgBoAgsBdEcBUQ4sQ9A/HqD0JI8RpBzH2OYVgahLBHFiJQJweQiDhDUE4SAARQAzFsG0EQwA6AOWSBkFgVAIBCHeGERQFQiCQHeFkC4vkiB8DyB4F4QxVDvGMNEOQexMjlBeOAKQiQLgfDA7QEAaRiBdEkH8" &
+"TI7AZiFBAGYBIABWjYBiGACioQ4C1A+AMMgWhfgxHgPsT4URIB0COKgPgjRwiAB8AYUArxBgCF6J4GY5hrAOCAPAAoGRRCsCIMEXATXfgAF8BMJwURuEQDgD4Q4OBoAeHGFgLIwQrC2D0JoSQ+QvhrHoSgQI8AbDFGID8C4Ah6BQAQAASACwgCYCMAUMARAvCKAiAMCAokeCKBEOAKgCBoDaHuMsEAqwJDiACDURg8R6gPCyDofYWAhgoDIJ4ZAuhoiGAYGgRoQw/A0GMMga4GwxiEDeIYYInATCDBQAoBAwAoDlA0KMBoVRGiDGwDQUYIRsgaGGDgM4LAwDWB8EcIA1APhjEgGQVwgRIgjFIIQHokgZA+CSEkLIKQSjHAwMsCwDAsAEB2ABTIwRwD6A8CMToSxkAxE4HYIw+BsgbBEDAHYBwojCBoIYFgXSjABE4MsHIbQWhlGILQS4UhvBdAUKEEwHgxDAAABQQQUAhgKHiDwE4JS4A7BGLQZwCR4gaBEMUYAqgKApHgGwVAIRNgvBMMQXImwZDtE4I8UIyAZCDCAE8AwrhgAdEEBACQLRCg4FEB4AYtA7CdEiPQMoJAMDNCkOMCAXAFDhH0D0Q4EgfAaGSK4NYzRUj9BuCgAgswOBjB4Fqpw8B2ADAwE4A4Qx2DAE6JIaQPQGhAGKBcIQ5B5gHByKIFARwADbAyKUfgdBKBBGyEcVIAB/ijHoIoSA0gdBNl+OATYERZgBGSDYWIWAUCEGKA4FAhR7CIBt" &
+"YQGYZg4CMAiKEcAOwkBjHWE8Z4lQgA+DkBoTohwwCeAaMEEgBQCCABgHMRwQRhhMEWFQd4HwZgwDqFESItAbAGEANCpINAzANCCJkK4ah+heFYBURwsQrS2CsMYMoWGBhYDWI0EInQgiApXaOVI1QFDsC8MUNoMBMA1HMJga4eh+BeAWOgNNowGjYzCGAAwax+iJBeBVT4gxoBIAGFsJFBxgBiGKFkKQ7g5DFFQEcAo4AzDDACKEQQLgCiJDYB0MgRBCCQAgQEA==")
var_Appearance.Add(3,"gBFLBCJwBAEHhEJAAEhABQ4Fg6AADACAxRDgMQBQKAAzQFAYbhkGCGAAGMZxRgmFgAQhFcZQSKUOQTDKMIziaQAGgkNQwCSLIwjNIsBxPFKVQChEYxSjKA40SJNUgyj6CCY+QLIE5PfQgAL9I6eJABCCSQKkYx0HScRiwPBIbAZAYhCZqaKhWgkKI/WBQIABRDVLx5ESiLRtKy7Mq2bpvXBcNxXHalaztO68LxvKyqHb5fJ/PpgL4YHgmC4NQ7EMRwF6rfbyfZ7Xg/ORPTijZ4sdzMHTzJyscx3HqfaBoOaZU5eMLceTUMofHIndxCcasPbsLpOS5LNKsaxmWLYdhFdTxQi6LpvfA8BwXC6JY7heRYRbFbYxRjGNi1TS7G4nGKd5WGuL4UHwI4VkaYxii8V4pgQMgVBQdQ5iCTYGi8T4vlWbJ3nuPg+l+H5AlSCg6ByPBoE8Ap3jqYxhBido5g0OgOGOGI4CsSpCCAcgcAuEosiYN5NHMOJ+D4TpTnSeQ7CEY4uBmBpPhgagdgcIZoHibIEyUBJZDQIJShoCgcCAcoyAQOYYlcZJ1D0DxDCiQgwEiAZMHEMJLFKPJ9D2DoDnidQ4k+Y5QmKEROBkIhKD0JIZDIS4TGUCQuEeEJjnOIg8CuY4RkYNgwGMM5RllGpThDRYIGKZIpCkJFUH0PINyWcQ3CaaZCG+HBnEOTJhD8Tx4GoeQ/GcaZSHOH5nCmQhshoZhihYYwhiYA4RlkNoNEMUZ3ByDjwEsPxOnMa" ;
+"J9DuDR6F6GYmCmKh0nANtMioP4Gg8aoSiIO5NhodociqaY6GaFYkEyOg8lsNRNTaUgykyMgQG0GpPiONJbD8DpDEyfA6k0KwOkWMQsGsAJU0SagwkoJQJDIPISCQCJTGSUwyGaM4KkmMgtksHpFjAZ4TGCBAbgaSpcksdhNAMIJHHsD5TjSWWMAMOpwjyLwbk6cAz0KRJiDkDYzESCwiggcgcgYIQwCIEINCMCITj6TVxkMXp2j0cQLlCTo7E2F4ymkMZdhMPJHDGHpLAyVg+k4UwrCCSIyByDJ8DuDY8CiWY0kiXAXC6QJwFKGIjCeJpjgyezjlyDw6klHx5myRoMGwZwbkcToTEiew4kwbQfEmUgPkOKJUD4DpTHSHQmgkXI/ASTA1g0XIEDMTBimyfI7jSLYHEiUoPk0Fw/kadAsHGao8A0A5smEMJ2mNyg5gzJZwDgCpChyIZVyIZwFCMJEPASRkBqE+IcHInRcDxA2H4bIsx0AtDsIBpwZwYicD6BscwDwUBgHCIYaIfgtiVH2O4WgUwJjEFeAEQA7y4hMCiBMS4aRdB9A4CYE4LxljyBMHcDItBxinDCLcTYmgejBFQ9UTg9gFBOEmAQTI7A4iZGMGkQAWQ7jYA2HIL8BRAjDG4HcCwARbjZHiNoDw1nLDnGyNINQ+wjCpBMEgcovQUgICQJEcgWRuBvAyJ4d4ugpCUAINcHogxIgnDiM4N4axzD3F2JMTY/hRqYF6FsWIxhYAGGoAALQYgYirBwBE" &
+"BwpAjBEAAIEIYsA2gOHCMAGgXAACIDmMITAUgFABH0D0I4WwvhNFGMAOIvxRD2GKNcMA8gjAPDCPwBogRPAxA8PgRwZRICYDED8RAXQEghEAN8DIgwIBdB4JYWwMgtiQHoFQKAiRFguFKGwGQhglDsEOVwEQQRkCKBwOIHgSREDRBYHEXQcQdD7GIGARQHRxipBrMobgewDCUCADsEYWAzgMHKHQDQxxsDzA6EMfAeQHB4GQDkUYPA0iECiKoGgRhcDdA2GMQA8AOCjDSPgHI4QnApAKBICwHg1A+BcAwcYsgbjGGQNkCIgRsA6EcBEWANADjsB0B8YYzQQDIGSBcEYZBCCPEkFIHQSgkgZAwG4IwBAbAYGGAgL4Ch4g8BOCQAA2KKC0GcAke4AAXAFCoHkDw4xbBFEcJkE4JRSiEFeJYKQVRMgJHODwX4xAgC/AsIIZAeAHDRG0HYI40RKCLEGDUI4jAghwBWIceA+whCpHMFYZYOQxglDMHMBQGxYjVHiAoBIPgfgHGwPsHYJRSB6A0IERQLhCjJHMA4OQoAoCOACLYGYSx8DpBQIMWQdRnDRH+DsE4fB3CeAmM67kAAXBFEIDYDI7wLBtEoEIfYNwjiUGGJQYQMAjCHEAO0C4zxW29CoCgfIxR9AKA6J8BgUAIhDGMIoJ40hqgwCgKETgnBhhqCGI0AIqgZhGDANQDIlBDCRGkCoJISR0g1BSKQOgfAzBRG0DYHARh4DeDAOwANuw8ApCKKkYg/RPhjBs" &
+"H0J4yg5hPGWN0GwFBHQBFaDoQIURljFAoB4GgzRVzbBECQFQRQoguHGHANwDRdCKy8CgSIGwhhoDYJYYI1giBICSAEgI=")
oSurface.Template = [Background(70) = 16777216] // oSurface.Background(70) = 0x1000000
oSurface.Template = [Background(71) = 33554432] // oSurface.Background(71) = 0x2000000
oSurface.Template = [Background(72) = 50331648] // oSurface.Background(72) = 0x3000000
var_Elements = oSurface.Elements
var_Element = oSurface.Elements.Add("Element Check 1",-64,-64)
// var_Elements.Add("Child").Parent = var_Element
var_Element1 = var_Elements.Add("Child")
with (oSurface)
TemplateDef = [dim var_Element1]
TemplateDef = var_Element1
Template = [var_Element1.Parent = var_Element]
endwith
var_Element.Pictures = "1/2"
var_Element.Expanded = false
var_Element2 = var_Elements.Add("Element Check 2",32,96)
var_Element2.Checked = 1
var_Element2.Pictures = "1,2"
oSurface.Home()
oSurface.EndUpdate()
|
129
|
ImageSize property on 16 (default) (specifies the size of control' icons)
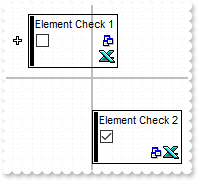
/*
with (this.EXSURFACEACTIVEXCONTROL1.nativeObject)
AddElement = class::nativeObject_AddElement
endwith
*/
// A new element has been added to the surface.
function nativeObject_AddElement(Element)
/* Element.ShowCheckBox = True */
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
return
local oSurface,var_Element,var_Element1,var_Element2,var_Elements
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.ImageSize = 16
oSurface.Images("gBJJgBAIDAAEg4ACEKAD/hz/EMNh8TIRNGwAjEZAEXjAojJAjMLjABAAgjUYkUnlUrlktl0vmExmUzmk1m03nE5nU7nkrQCAntBoVDolFo1HoM/ADAplLptImdMYFOqdSqlXq1QrVbrlGpVWsFNrNdnNjsk7pQAtNroFnt0sh8Yr9iulTuNxs1Eu8OiT/vsnsNVutXlk/oGGtVKxGLxWNtsZtN8iUYuNvy0Zvd+xNYwdwvl4p870GCqc8vOeuVttmp1knyOayWVy+WzN/ze1wOElenm+12WUz/Bv2/3UyyWrzeutux2GSyGP2dQ33C1ur3GD3M4zUNzHdlWjq/E3nGzVpjWv4HA7fRy/Tv2IrN8rPW6nZ3ve7mUlfu20Z8acvQyb+vY9jasYoDwMm+LytVBDqKG3z8O3Cb8P+mkAuY9cCQ2uL4KaxDKvkp8RNLEjqugnrwQo/UWPzFyeQw5sNLZFENrI4kOqU66pw8uzmOKvTqNqjULJvGL1JO48GtTGsbLdEL3scxLlyiw8dQeoUVxdLTtyKmUjwGlslRPJsnK1HbAKbKCrsQo8uQk/CeP44iaR/ATnTNPLvyxPU+z9P9AUDQVBowiofJXQ6Oo+kKMpIkjztE4TKn4P6JowfgPnwD5/nAjB8AOeAPo0eAA1IAFH07UhAIMpYAVIYFHqBUhwVjV1S1EtQAHxW65V0AZwAeuQAnwB5gAPYViEDVhwAHTQBkCjB4gOhwDmCyhH0sACAg==")
var_Elements = oSurface.Elements
var_Element = oSurface.Elements.Add("Element Check 1",-64,-64)
// var_Elements.Add("Child").Parent = var_Element
var_Element1 = var_Elements.Add("Child")
with (oSurface)
TemplateDef = [dim var_Element1]
TemplateDef = var_Element1
Template = [var_Element1.Parent = var_Element]
endwith
var_Element.Pictures = "1/2"
var_Element.Expanded = false
var_Element2 = var_Elements.Add("Element Check 2",0,32)
var_Element2.Checked = 1
var_Element2.Pictures = "1,2"
oSurface.Home()
oSurface.EndUpdate()
|
128
|
We want to have option to start/end connectors at the middle of each side of the elements
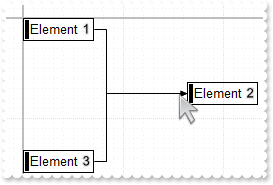
local oSurface,var_Elements,var_Links
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.ShowLinks = -1
var_Elements = oSurface.Elements
var_Elements.Add("Element <sha ;;0>1")
var_Elements.Add("Element <sha ;;0>2",164,64)
var_Elements.Add("Element <sha ;;0>3",0,132)
var_Links = oSurface.Links
var_Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2))
var_Links.Add(oSurface.Elements.Item(3),oSurface.Elements.Item(2))
oSurface.FitToClient()
oSurface.EndUpdate()
|
127
|
How can I determine the position the user clicks within the element's boundaries (Click event)
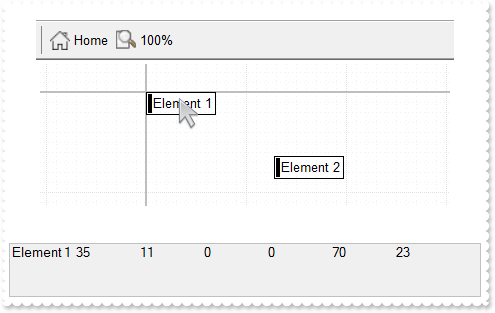
/*
with (this.EXSURFACEACTIVEXCONTROL1.nativeObject)
Click = class::nativeObject_Click
endwith
*/
// Occurs when the user presses and then releases the left mouse button over the control.
function nativeObject_Click()
local X,Y,e
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
e = oSurface.ElementFromPoint(-1,-1)
X = -1
Y = -1
oSurface.PointToPosition(X,Y)
? Str(e)
? Str(X)
? Str(Y)
return
local oSurface,var_Elements
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
var_Elements = oSurface.Elements
var_Elements.Add("Element 1")
var_Elements.Add("Element 2",128,64)
oSurface.FitToClient()
oSurface.EndUpdate()
|
126
|
How can I determine the position the user clicks within the element's boundaries (MouseMove event)
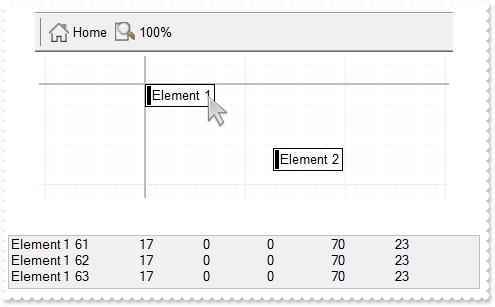
/*
with (this.EXSURFACEACTIVEXCONTROL1.nativeObject)
MouseMove = class::nativeObject_MouseMove
endwith
*/
// Occurs when the user moves the mouse.
function nativeObject_MouseMove(Button,Shift,X,Y)
local e
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
e = oSurface.ElementFromPoint(X,Y)
oSurface.PointToPosition(X,Y)
? Str(e)
? Str(X)
? Str(Y)
return
local oSurface,var_Elements
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
var_Elements = oSurface.Elements
var_Elements.Add("Element 1")
var_Elements.Add("Element 2",128,64)
oSurface.FitToClient()
oSurface.EndUpdate()
|
125
|
How can I convert the screen position (mouse) to surface position
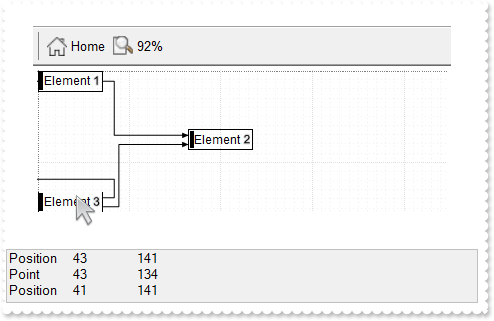
/*
with (this.EXSURFACEACTIVEXCONTROL1.nativeObject)
MouseMove = class::nativeObject_MouseMove
endwith
*/
// Occurs when the user moves the mouse.
function nativeObject_MouseMove(Button,Shift,X,Y)
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
? "Point "
? Str(X)
? Str(Y)
oSurface.PointToPosition(X,Y)
? "Position "
? Str(X)
? Str(Y)
return
local oSurface,var_Elements,var_Links
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
var_Elements = oSurface.Elements
var_Elements.Add("Element <sha ;;0>1")
var_Elements.Add("Element <sha ;;0>2",164,64)
var_Elements.Add("Element <sha ;;0>3",0,132)
var_Links = oSurface.Links
var_Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2))
var_Links.Add(oSurface.Elements.Item(3),oSurface.Elements.Item(2))
var_Links.Add(oSurface.Elements.Item(3),oSurface.Elements.Item(1))
oSurface.FitToClient()
oSurface.AxisStyle = 192 /*0xc0 | */
oSurface.AxisColor = 0x808080
oSurface.EndUpdate()
|
124
|
Is is possible to show just the positive coordinates
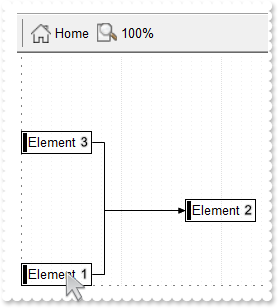
local oSurface,var_Elements,var_Links
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.Coord = 17 /*exAllowPositiveOnly | exCartesian*/
oSurface.AxisColor = 0x808080
oSurface.AxisStyle = 259 /*exLinesThick | exLinesDot4*/
oSurface.ShowLinks = -1
var_Elements = oSurface.Elements
var_Elements.Add("Element <sha ;;0>1")
var_Elements.Add("Element <sha ;;0>2",164,64)
var_Elements.Add("Element <sha ;;0>3",0,132)
var_Links = oSurface.Links
var_Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2))
var_Links.Add(oSurface.Elements.Item(3),oSurface.Elements.Item(2))
oSurface.FitToClient()
oSurface.EndUpdate()
|
123
|
Cartesian coordinates (positive coordinates are shown top-right to the origin)
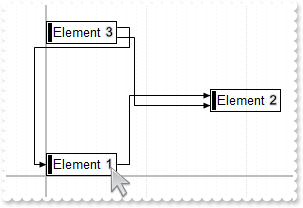
local oSurface,var_Elements,var_Links
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.Coord = 1
var_Elements = oSurface.Elements
var_Elements.Add("Element <sha ;;0>1")
var_Elements.Add("Element <sha ;;0>2",164,64)
var_Elements.Add("Element <sha ;;0>3",0,132)
var_Links = oSurface.Links
var_Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2))
var_Links.Add(oSurface.Elements.Item(3),oSurface.Elements.Item(2))
var_Links.Add(oSurface.Elements.Item(3),oSurface.Elements.Item(1))
oSurface.FitToClient()
oSurface.EndUpdate()
|
122
|
Default coordinates (positive coordinates are shown bottom-right to the origin)
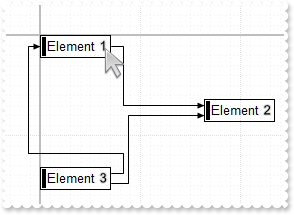
local oSurface,var_Elements,var_Links
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.Coord = 0
var_Elements = oSurface.Elements
var_Elements.Add("Element <sha ;;0>1")
var_Elements.Add("Element <sha ;;0>2",164,64)
var_Elements.Add("Element <sha ;;0>3",0,132)
var_Links = oSurface.Links
var_Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2))
var_Links.Add(oSurface.Elements.Item(3),oSurface.Elements.Item(2))
var_Links.Add(oSurface.Elements.Item(3),oSurface.Elements.Item(1))
oSurface.FitToClient()
oSurface.EndUpdate()
|
121
|
Is it possible to customize the path of the links orthogonally similar with Microsoft Visio tool
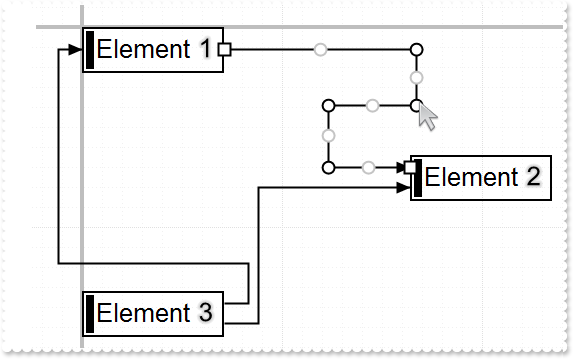
local oSurface,var_Elements,var_Links
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.AllowLinkControlPoint = 31 /*exOrthoArrange | exMiddleControlPoint | exControlPoint | exEndControlPoint | exStartControlPoint*/
var_Elements = oSurface.Elements
var_Elements.Add("Element <sha ;;0>1")
var_Elements.Add("Element <sha ;;0>2",164,64)
var_Elements.Add("Element <sha ;;0>3",0,132)
var_Links = oSurface.Links
var_Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2))
var_Links.Add(oSurface.Elements.Item(3),oSurface.Elements.Item(2))
var_Links.Add(oSurface.Elements.Item(3),oSurface.Elements.Item(1))
oSurface.Zoom = 200
oSurface.FitToClient()
oSurface.EndUpdate()
|
120
|
Does your control supports OLE Drag and Drop
/*
with (this.EXSURFACEACTIVEXCONTROL1.nativeObject)
OLEDragDrop = class::nativeObject_OLEDragDrop
endwith
*/
// Occurs when a source component is dropped onto a target component when the source component determines that a drop can occur.
function nativeObject_OLEDragDrop(Data,Effect,Button,Shift,X,Y)
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
? Str(Data)
return
/*
with (this.EXSURFACEACTIVEXCONTROL1.nativeObject)
OLEStartDrag = class::nativeObject_OLEStartDrag
endwith
*/
// Occurs when the OLEDrag method is called.
function nativeObject_OLEStartDrag(Data,AllowedEffects)
/* Data.SetData("some data to drag") */
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
return
local oSurface,var_Element
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.OLEDropMode = 1
var_Element = oSurface.Elements.Add("Click the Element wait for .5 second until + cursor is shown, to start <b>OLE Drag and Drop</b>")
var_Element.CaptionSingleLine = false
var_Element.AutoSize = false
var_Element.Width = 256
var_Element.Height = 56
|
119
|
Is it possible to disable customizing the path of a specified link
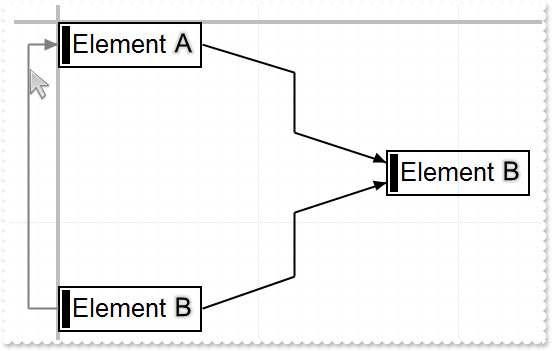
local oSurface,var_Elements,var_Link,var_Link1,var_Link2,var_Links
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.AllowLinkControlPoint = -1 /*0xffffff80 | exAllowChangeTo | exAllowChangeFrom | exOrthoArrange | exMiddleControlPoint | exControlPoint | exEndControlPoint | exStartControlPoint*/
var_Elements = oSurface.Elements
var_Elements.Add("Element <sha ;;0>A")
var_Elements.Add("Element <sha ;;0>B",164,64)
var_Elements.Add("Element <sha ;;0>B",0,132)
var_Links = oSurface.Links
// var_Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2)).CustomPath = "0.5,0.25,0.5,.75"
var_Link = var_Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2))
with (oSurface)
TemplateDef = [dim var_Link]
TemplateDef = var_Link
Template = [var_Link.CustomPath = "0.5,0.25,0.5,.75"]
endwith
// var_Links.Add(oSurface.Elements.Item(3),oSurface.Elements.Item(2)).CustomPath = "0.5,0.25,0.5,.75"
var_Link1 = var_Links.Add(oSurface.Elements.Item(3),oSurface.Elements.Item(2))
with (oSurface)
TemplateDef = [dim var_Link1]
TemplateDef = var_Link1
Template = [var_Link1.CustomPath = "0.5,0.25,0.5,.75"]
endwith
var_Link2 = var_Links.Add(oSurface.Elements.Item(3),oSurface.Elements.Item(1))
var_Link2.ShowLinkType = 3 /*exLinkStraight | exLinkDirect*/
var_Link2.StartPos = 0
var_Link2.Color = 0x808080
var_Link2.AllowControlPoint = 0
oSurface.Zoom = 200
oSurface.FitToClient()
oSurface.EndUpdate()
|
118
|
How do I let user customizes the link's path
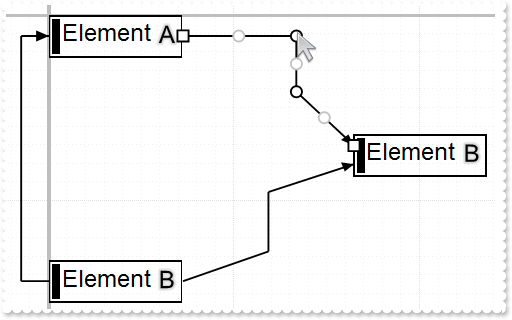
local oSurface,var_Elements,var_Link,var_Link1,var_Link2,var_Links
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.AllowLinkControlPoint = -1 /*0xffffff80 | exAllowChangeTo | exAllowChangeFrom | exOrthoArrange | exMiddleControlPoint | exControlPoint | exEndControlPoint | exStartControlPoint*/
var_Elements = oSurface.Elements
var_Elements.Add("Element <sha ;;0>A")
var_Elements.Add("Element <sha ;;0>B",164,64)
var_Elements.Add("Element <sha ;;0>B",0,132)
var_Links = oSurface.Links
// var_Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2)).CustomPath = "0.5,0.25,0.5,.75"
var_Link = var_Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2))
with (oSurface)
TemplateDef = [dim var_Link]
TemplateDef = var_Link
Template = [var_Link.CustomPath = "0.5,0.25,0.5,.75"]
endwith
// var_Links.Add(oSurface.Elements.Item(3),oSurface.Elements.Item(2)).CustomPath = "0.5,0.25,0.5,.75"
var_Link1 = var_Links.Add(oSurface.Elements.Item(3),oSurface.Elements.Item(2))
with (oSurface)
TemplateDef = [dim var_Link1]
TemplateDef = var_Link1
Template = [var_Link1.CustomPath = "0.5,0.25,0.5,.75"]
endwith
var_Link2 = var_Links.Add(oSurface.Elements.Item(3),oSurface.Elements.Item(1))
var_Link2.ShowLinkType = 3 /*exLinkStraight | exLinkDirect*/
var_Link2.StartPos = 0
oSurface.Zoom = 200
oSurface.FitToClient()
oSurface.EndUpdate()
|
117
|
How can I generate a picture/image/graph from my diagram
local oSurface,var_CopyTo,var_Element,var_Element1,var_Element2,var_Element3,var_Element4,var_Element5,var_Elements,var_Links
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
var_Elements = oSurface.Elements
// var_Elements.Add("Element A").ID = "A"
var_Element = var_Elements.Add("Element A")
with (oSurface)
TemplateDef = [dim var_Element]
TemplateDef = var_Element
Template = [var_Element.ID = "A"]
endwith
// var_Elements.Add("Element B").ID = "B"
var_Element1 = var_Elements.Add("Element B")
with (oSurface)
TemplateDef = [dim var_Element1]
TemplateDef = var_Element1
Template = [var_Element1.ID = "B"]
endwith
// var_Elements.Add("Element C").ID = "C"
var_Element2 = var_Elements.Add("Element C")
with (oSurface)
TemplateDef = [dim var_Element2]
TemplateDef = var_Element2
Template = [var_Element2.ID = "C"]
endwith
// var_Elements.Add("Element D").ID = "D"
var_Element3 = var_Elements.Add("Element D")
with (oSurface)
TemplateDef = [dim var_Element3]
TemplateDef = var_Element3
Template = [var_Element3.ID = "D"]
endwith
// var_Elements.Add("Element E").ID = "E"
var_Element4 = var_Elements.Add("Element E")
with (oSurface)
TemplateDef = [dim var_Element4]
TemplateDef = var_Element4
Template = [var_Element4.ID = "E"]
endwith
// var_Elements.Add("Element E").ID = "F"
var_Element5 = var_Elements.Add("Element E")
with (oSurface)
TemplateDef = [dim var_Element5]
TemplateDef = var_Element5
Template = [var_Element5.ID = "F"]
endwith
var_Links = oSurface.Links
var_Links.Add(oSurface.Elements.Item("A"),oSurface.Elements.Item("B"))
var_Links.Add(oSurface.Elements.Item("A"),oSurface.Elements.Item("C"))
var_Links.Add(oSurface.Elements.Item("B"),oSurface.Elements.Item("D"))
var_Links.Add(oSurface.Elements.Item("B"),oSurface.Elements.Item("C"))
var_Links.Add(oSurface.Elements.Item("A"),oSurface.Elements.Item("E"))
var_Links.Add(oSurface.Elements.Item("A"),oSurface.Elements.Item("F"))
oSurface.Template = [DefArrange(4) = False] // oSurface.DefArrange(4) = false
oSurface.Arrange()
var_CopyTo = oSurface.CopyTo("c:/temp/xtest.jpg")
? "!!!check the file c:/temp/xtest.jpg!!!"
|
116
|
How can I generate a picture/image/graph from my diagram
local oSurface,var_Element,var_Element1,var_Element2,var_Element3,var_Element4,var_Element5,var_Elements,var_Links,var_Print
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
var_Elements = oSurface.Elements
// var_Elements.Add("Element A").ID = "A"
var_Element = var_Elements.Add("Element A")
with (oSurface)
TemplateDef = [dim var_Element]
TemplateDef = var_Element
Template = [var_Element.ID = "A"]
endwith
// var_Elements.Add("Element B").ID = "B"
var_Element1 = var_Elements.Add("Element B")
with (oSurface)
TemplateDef = [dim var_Element1]
TemplateDef = var_Element1
Template = [var_Element1.ID = "B"]
endwith
// var_Elements.Add("Element C").ID = "C"
var_Element2 = var_Elements.Add("Element C")
with (oSurface)
TemplateDef = [dim var_Element2]
TemplateDef = var_Element2
Template = [var_Element2.ID = "C"]
endwith
// var_Elements.Add("Element D").ID = "D"
var_Element3 = var_Elements.Add("Element D")
with (oSurface)
TemplateDef = [dim var_Element3]
TemplateDef = var_Element3
Template = [var_Element3.ID = "D"]
endwith
// var_Elements.Add("Element E").ID = "E"
var_Element4 = var_Elements.Add("Element E")
with (oSurface)
TemplateDef = [dim var_Element4]
TemplateDef = var_Element4
Template = [var_Element4.ID = "E"]
endwith
// var_Elements.Add("Element E").ID = "F"
var_Element5 = var_Elements.Add("Element E")
with (oSurface)
TemplateDef = [dim var_Element5]
TemplateDef = var_Element5
Template = [var_Element5.ID = "F"]
endwith
var_Links = oSurface.Links
var_Links.Add(oSurface.Elements.Item("A"),oSurface.Elements.Item("B"))
var_Links.Add(oSurface.Elements.Item("A"),oSurface.Elements.Item("C"))
var_Links.Add(oSurface.Elements.Item("B"),oSurface.Elements.Item("D"))
var_Links.Add(oSurface.Elements.Item("B"),oSurface.Elements.Item("C"))
var_Links.Add(oSurface.Elements.Item("A"),oSurface.Elements.Item("E"))
var_Links.Add(oSurface.Elements.Item("A"),oSurface.Elements.Item("F"))
oSurface.Template = [DefArrange(4) = False] // oSurface.DefArrange(4) = false
oSurface.Arrange()
var_Print = new OleAutoClient("Exontrol.Print")
var_Print.PrintExt = oSurface
var_Print.CopyTo("c:/temp/xtest.jpg")
? "!!!check the file c:/temp/xtest.jpg!!!"
|
115
|
How can I print the component
local oSurface,var_Element,var_Element1,var_Elements,var_Link,var_Link1,var_Link2,var_Links,var_Print
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
var_Elements = oSurface.Elements
var_Elements.Add("Element <sha ;;0>A")
var_Elements.Add("Element <sha ;;0>B",0,76)
var_Element = var_Elements.Add("Element <sha ;;0>C",-76,32)
var_Element.AutoSize = false
var_Element.Height = 32
var_Element1 = var_Elements.Add("Element <sha ;;0>D",76,32)
var_Element1.AutoSize = false
var_Element1.Height = 32
var_Links = oSurface.Links
var_Link = var_Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2))
var_Link.StartPos = 1
var_Link.EndPos = 1
var_Link1 = var_Links.Add(oSurface.Elements.Item(2),oSurface.Elements.Item(1))
var_Link1.StartPos = 1
var_Link1.EndPos = 1
var_Links.Add(oSurface.Elements.Item(3),oSurface.Elements.Item(4))
var_Link2 = var_Links.Add(oSurface.Elements.Item(4),oSurface.Elements.Item(3))
var_Link2.StartPos = 0
var_Link2.EndPos = 2
var_Print = new OleAutoClient("Exontrol.Print")
var_Print.PrintExt = oSurface
var_Print.Preview()
|
114
|
How can I show direct-links
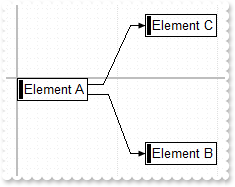
local oSurface,var_Element,var_Element1,var_Element2,var_Elements,var_Links
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.ShowLinksType = 1
var_Elements = oSurface.Elements
// var_Elements.Add("Element A").ID = "A"
var_Element = var_Elements.Add("Element A")
with (oSurface)
TemplateDef = [dim var_Element]
TemplateDef = var_Element
Template = [var_Element.ID = "A"]
endwith
// var_Elements.Add("Element B",128,64).ID = "B"
var_Element1 = var_Elements.Add("Element B",128,64)
with (oSurface)
TemplateDef = [dim var_Element1]
TemplateDef = var_Element1
Template = [var_Element1.ID = "B"]
endwith
// var_Elements.Add("Element C",128,-64).ID = "C"
var_Element2 = var_Elements.Add("Element C",128,-64)
with (oSurface)
TemplateDef = [dim var_Element2]
TemplateDef = var_Element2
Template = [var_Element2.ID = "C"]
endwith
var_Links = oSurface.Links
var_Links.Add(oSurface.Elements.Item("A"),oSurface.Elements.Item("B"))
var_Links.Add(oSurface.Elements.Item("A"),oSurface.Elements.Item("C"))
|
113
|
How can I show straight-links
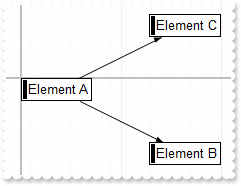
local oSurface,var_Element,var_Element1,var_Element2,var_Elements,var_Links
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.ShowLinksType = 2
var_Elements = oSurface.Elements
// var_Elements.Add("Element A").ID = "A"
var_Element = var_Elements.Add("Element A")
with (oSurface)
TemplateDef = [dim var_Element]
TemplateDef = var_Element
Template = [var_Element.ID = "A"]
endwith
// var_Elements.Add("Element B",128,64).ID = "B"
var_Element1 = var_Elements.Add("Element B",128,64)
with (oSurface)
TemplateDef = [dim var_Element1]
TemplateDef = var_Element1
Template = [var_Element1.ID = "B"]
endwith
// var_Elements.Add("Element C",128,-64).ID = "C"
var_Element2 = var_Elements.Add("Element C",128,-64)
with (oSurface)
TemplateDef = [dim var_Element2]
TemplateDef = var_Element2
Template = [var_Element2.ID = "C"]
endwith
var_Links = oSurface.Links
var_Links.Add(oSurface.Elements.Item("A"),oSurface.Elements.Item("B"))
var_Links.Add(oSurface.Elements.Item("A"),oSurface.Elements.Item("C"))
|
112
|
How can I show round-links
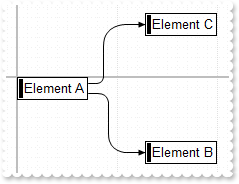
local oSurface,var_Element,var_Element1,var_Element2,var_Elements,var_Links
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.ShowLinksType = -1
var_Elements = oSurface.Elements
// var_Elements.Add("Element A").ID = "A"
var_Element = var_Elements.Add("Element A")
with (oSurface)
TemplateDef = [dim var_Element]
TemplateDef = var_Element
Template = [var_Element.ID = "A"]
endwith
// var_Elements.Add("Element B",128,64).ID = "B"
var_Element1 = var_Elements.Add("Element B",128,64)
with (oSurface)
TemplateDef = [dim var_Element1]
TemplateDef = var_Element1
Template = [var_Element1.ID = "B"]
endwith
// var_Elements.Add("Element C",128,-64).ID = "C"
var_Element2 = var_Elements.Add("Element C",128,-64)
with (oSurface)
TemplateDef = [dim var_Element2]
TemplateDef = var_Element2
Template = [var_Element2.ID = "C"]
endwith
var_Links = oSurface.Links
var_Links.Add(oSurface.Elements.Item("A"),oSurface.Elements.Item("B"))
var_Links.Add(oSurface.Elements.Item("A"),oSurface.Elements.Item("C"))
|
111
|
I've tried to insert a "<br>" in the Caption property text and it just ignores it
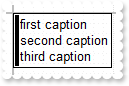
local oSurface,var_Element,var_Elements
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
var_Elements = oSurface.Elements
var_Element = var_Elements.Add("caption")
var_Element.CaptionSingleLine = false
var_Element.Caption = "first caption<br>second caption<br>third caption"
oSurface.EndUpdate()
|
110
|
How do I align the extra-caption
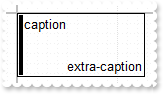
local oSurface,var_Element,var_Elements
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
var_Elements = oSurface.Elements
var_Element = var_Elements.Add("caption")
var_Element.AutoSize = false
var_Element.Width = 128
var_Element.Height = 64
var_Element.ExtraCaption = "extra-caption"
var_Element.ExtraCaptionAlign = 34
oSurface.EndUpdate()
|
109
|
How can I add an extra caption
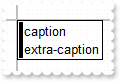
local oSurface,var_Element,var_Elements
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
var_Elements = oSurface.Elements
// var_Elements.Add("caption").ExtraCaption = "extra-caption"
var_Element = var_Elements.Add("caption")
with (oSurface)
TemplateDef = [dim var_Element]
TemplateDef = var_Element
Template = [var_Element.ExtraCaption = "extra-caption"]
endwith
oSurface.EndUpdate()
|
108
|
I am using the reserve-neighbors feature, the question is how to shift left/right the neighbors instead of up/down
local oSurface,var_Elements
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.AllowMoveNeighbors = 2
oSurface.Template = [DefArrange(0) = 1] // oSurface.DefArrange(0) = 1
oSurface.AllowInsertObject = false
var_Elements = oSurface.Elements
var_Elements.Add("Element <sha ;;0>A")
var_Elements.Add("Element <sha ;;0>B ( move it )",16,32)
var_Elements.Add("Element <sha ;;0>C",128,0)
oSurface.EndUpdate()
|
107
|
I am using the reserve-neighbors feature, the question is if possible to specify the distance between neighbors
local oSurface,var_Elements
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.AllowMoveNeighbors = 1
oSurface.Template = [DefArrange(1) = 0] // oSurface.DefArrange(1) = 0
oSurface.Template = [DefArrange(2) = 0] // oSurface.DefArrange(2) = 0
oSurface.AllowInsertObject = false
var_Elements = oSurface.Elements
var_Elements.Add("Element <sha ;;0>A")
var_Elements.Add("Element <sha ;;0>B ( move it )",0,32)
var_Elements.Add("Element <sha ;;0>C",0,64)
oSurface.EndUpdate()
|
106
|
How do I enable the reserve-neighbors feature
local oSurface,var_Elements
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
oSurface.BeginUpdate()
oSurface.AllowMoveNeighbors = 1
oSurface.AllowInsertObject = false
var_Elements = oSurface.Elements
var_Elements.Add("Element <sha ;;0>A")
var_Elements.Add("Element <sha ;;0>B ( move it )",0,32)
var_Elements.Add("Element <sha ;;0>C",0,64)
oSurface.EndUpdate()
|
105
|
I've noticed that recently, the elements get compacted once the Arrange method is performed. How can I prevent that
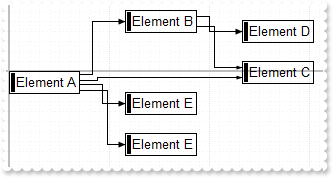
local oSurface,var_Element,var_Element1,var_Element2,var_Element3,var_Element4,var_Element5,var_Elements,var_Links
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
var_Elements = oSurface.Elements
// var_Elements.Add("Element A").ID = "A"
var_Element = var_Elements.Add("Element A")
with (oSurface)
TemplateDef = [dim var_Element]
TemplateDef = var_Element
Template = [var_Element.ID = "A"]
endwith
// var_Elements.Add("Element B").ID = "B"
var_Element1 = var_Elements.Add("Element B")
with (oSurface)
TemplateDef = [dim var_Element1]
TemplateDef = var_Element1
Template = [var_Element1.ID = "B"]
endwith
// var_Elements.Add("Element C").ID = "C"
var_Element2 = var_Elements.Add("Element C")
with (oSurface)
TemplateDef = [dim var_Element2]
TemplateDef = var_Element2
Template = [var_Element2.ID = "C"]
endwith
// var_Elements.Add("Element D").ID = "D"
var_Element3 = var_Elements.Add("Element D")
with (oSurface)
TemplateDef = [dim var_Element3]
TemplateDef = var_Element3
Template = [var_Element3.ID = "D"]
endwith
// var_Elements.Add("Element E").ID = "E"
var_Element4 = var_Elements.Add("Element E")
with (oSurface)
TemplateDef = [dim var_Element4]
TemplateDef = var_Element4
Template = [var_Element4.ID = "E"]
endwith
// var_Elements.Add("Element E").ID = "F"
var_Element5 = var_Elements.Add("Element E")
with (oSurface)
TemplateDef = [dim var_Element5]
TemplateDef = var_Element5
Template = [var_Element5.ID = "F"]
endwith
var_Links = oSurface.Links
var_Links.Add(oSurface.Elements.Item("A"),oSurface.Elements.Item("B"))
var_Links.Add(oSurface.Elements.Item("A"),oSurface.Elements.Item("C"))
var_Links.Add(oSurface.Elements.Item("B"),oSurface.Elements.Item("D"))
var_Links.Add(oSurface.Elements.Item("B"),oSurface.Elements.Item("C"))
var_Links.Add(oSurface.Elements.Item("A"),oSurface.Elements.Item("E"))
var_Links.Add(oSurface.Elements.Item("A"),oSurface.Elements.Item("F"))
oSurface.Template = [DefArrange(4) = False] // oSurface.DefArrange(4) = false
oSurface.Arrange()
|
104
|
Is it possible to add a link to show from bottom/down to top/up, rather that right to left (method-2)
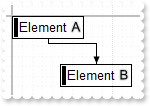
/*
with (this.EXSURFACEACTIVEXCONTROL1.nativeObject)
AddLink = class::nativeObject_AddLink
endwith
*/
// A new link has been added to the links collection.
function nativeObject_AddLink(Link)
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
return
local oSurface,var_Elements,var_Links
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
var_Elements = oSurface.Elements
var_Elements.Add("Element <sha ;;0>A")
var_Elements.Add("Element <sha ;;0>B",48,48)
var_Links = oSurface.Links
var_Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2))
|
103
|
Is it possible to add a link to show from bottom/down to top/up, rather that right to left (method-1)
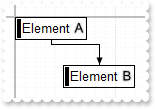
local oSurface,var_Elements,var_Link,var_Links
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
var_Elements = oSurface.Elements
var_Elements.Add("Element <sha ;;0>A")
var_Elements.Add("Element <sha ;;0>B",48,48)
var_Links = oSurface.Links
var_Link = var_Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2))
var_Link.StartPos = 4
var_Link.EndPos = 3
|
102
|
How do I enable the cross link support ( mixed )
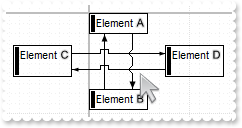
local oSurface,var_Element,var_Element1,var_Elements,var_Link,var_Link1,var_Link2,var_Links
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
var_Elements = oSurface.Elements
var_Elements.Add("Element <sha ;;0>A")
var_Elements.Add("Element <sha ;;0>B",0,76)
var_Element = var_Elements.Add("Element <sha ;;0>C",-76,32)
var_Element.AutoSize = false
var_Element.Height = 32
var_Element1 = var_Elements.Add("Element <sha ;;0>D",76,32)
var_Element1.AutoSize = false
var_Element1.Height = 32
var_Links = oSurface.Links
var_Link = var_Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2))
var_Link.StartPos = 1
var_Link.EndPos = 1
var_Link1 = var_Links.Add(oSurface.Elements.Item(2),oSurface.Elements.Item(1))
var_Link1.StartPos = 1
var_Link1.EndPos = 1
var_Links.Add(oSurface.Elements.Item(3),oSurface.Elements.Item(4))
var_Link2 = var_Links.Add(oSurface.Elements.Item(4),oSurface.Elements.Item(3))
var_Link2.StartPos = 0
var_Link2.EndPos = 2
oSurface.ShowLinks = 97 /*exShowCrossLinksMixt | exShowExtendedLinks*/
|
101
|
How do I enable the cross link support ( triangular )
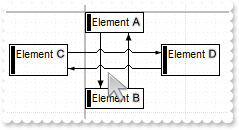
local oSurface,var_Element,var_Element1,var_Elements,var_Link,var_Link1,var_Link2,var_Links
oSurface = form.EXSURFACEACTIVEXCONTROL1.nativeObject
var_Elements = oSurface.Elements
var_Elements.Add("Element <sha ;;0>A")
var_Elements.Add("Element <sha ;;0>B",0,76)
var_Element = var_Elements.Add("Element <sha ;;0>C",-76,32)
var_Element.AutoSize = false
var_Element.Height = 32
var_Element1 = var_Elements.Add("Element <sha ;;0>D",76,32)
var_Element1.AutoSize = false
var_Element1.Height = 32
var_Links = oSurface.Links
var_Link = var_Links.Add(oSurface.Elements.Item(1),oSurface.Elements.Item(2))
var_Link.StartPos = 1
var_Link.EndPos = 1
var_Link1 = var_Links.Add(oSurface.Elements.Item(2),oSurface.Elements.Item(1))
var_Link1.StartPos = 1
var_Link1.EndPos = 1
var_Links.Add(oSurface.Elements.Item(3),oSurface.Elements.Item(4))
var_Link2 = var_Links.Add(oSurface.Elements.Item(4),oSurface.Elements.Item(3))
var_Link2.StartPos = 0
var_Link2.EndPos = 2
oSurface.ShowLinks = 65 /*exShowCrossLinksTriangle | exShowExtendedLinks*/
|