52
|
Print and Print Preview
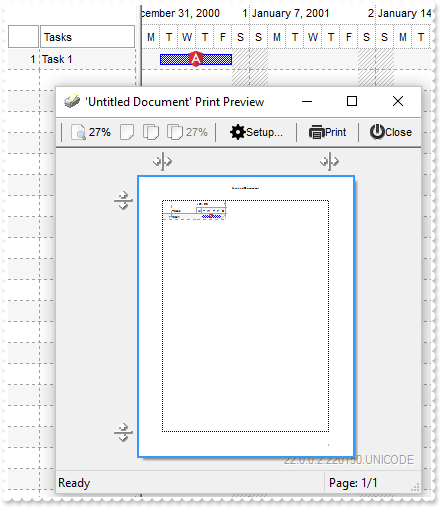
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
spG2Host1->PutHostReadOnly(EXG2HOSTLib::exHostReadOnly);
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2ANTTLib' for the library: 'ExG2antt 1.0 Control Library'
#import <ExG2antt.dll>
using namespace EXG2ANTTLib;
*/
EXG2ANTTLib::IG2anttPtr var_G2antt = ((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()));
var_G2antt->BeginUpdate();
var_G2antt->ReplaceIcon(_bstr_t("gAAAABgYACEHgUJFEEAAWhUJCEJEEJggEhMCYEXjUbjkJQECj8gj8hAEjkshYEpk8kf8ClsulsvAExmcvf83js5nU7nkCeEcn8boMaocXosCB9Hn09pkzcEuoL/fE+O") +
"kYB0gB9YhIHrddgVcr9aktZADAD8+P8CgIA==",vtMissing);
var_G2antt->ReplaceIcon("C:\\images\\favicon.ico",long(0));
EXG2ANTTLib::IChartPtr var_Chart = var_G2antt->GetChart();
var_Chart->PutFirstVisibleDate(COleDateTime(2001,1,1,0,00,00).operator DATE());
var_Chart->PutPaneWidth(VARIANT_FALSE,128);
var_Chart->PutLevelCount(2);
EXG2ANTTLib::IItemsPtr var_Items = var_G2antt->GetItems();
long h = var_Items->AddItem("Task 1");
var_Items->AddBar(h,"Task",COleDateTime(2001,1,2,0,00,00).operator DATE(),COleDateTime(2001,1,6,0,00,00).operator DATE(),"K1",vtMissing);
var_Items->PutItemBar(h,"K1",EXG2ANTTLib::exBarCaption,"<img>1</img>");
var_G2antt->EndUpdate();
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXPRINTLib' for the library: 'ExPrint 1.0 Control Library'
#import <ExPrint.dll>
using namespace EXPRINTLib;
*/
EXPRINTLib::IExPrintPtr var_Print = ::CreateObject(L"Exontrol.Print");
var_Print->PutPrintExt(((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost())));
var_Print->Preview();
|
51
|
How can I replace or add an icon at runtime
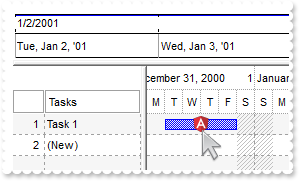
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2ANTTLib' for the library: 'ExG2antt 1.0 Control Library'
#import <ExG2antt.dll>
using namespace EXG2ANTTLib;
*/
EXG2ANTTLib::IG2anttPtr var_G2antt = ((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()));
var_G2antt->BeginUpdate();
var_G2antt->ReplaceIcon(_bstr_t("gAAAABgYACEHgUJFEEAAWhUJCEJEEJggEhMCYEXjUbjkJQECj8gj8hAEjkshYEpk8kf8ClsulsvAExmcvf83js5nU7nkCeEcn8boMaocXosCB9Hn09pkzcEuoL/fE+O") +
"kYB0gB9YhIHrddgVcr9aktZADAD8+P8CgIA==",vtMissing);
var_G2antt->ReplaceIcon("C:\\images\\favicon.ico",long(0));
EXG2ANTTLib::IChartPtr var_Chart = var_G2antt->GetChart();
var_Chart->PutFirstVisibleDate(COleDateTime(2001,1,1,0,00,00).operator DATE());
var_Chart->PutPaneWidth(VARIANT_FALSE,128);
var_Chart->PutLevelCount(2);
EXG2ANTTLib::IItemsPtr var_Items = var_G2antt->GetItems();
long h = var_Items->AddItem("Task 1");
var_Items->AddBar(h,"Task",COleDateTime(2001,1,2,0,00,00).operator DATE(),COleDateTime(2001,1,6,0,00,00).operator DATE(),"K1",vtMissing);
var_Items->PutItemBar(h,"K1",EXG2ANTTLib::exBarCaption,"<img>1</img>");
var_G2antt->EndUpdate();
|
50
|
How do I get the start/end of the bar once the BarResize/BarResizing event occurs
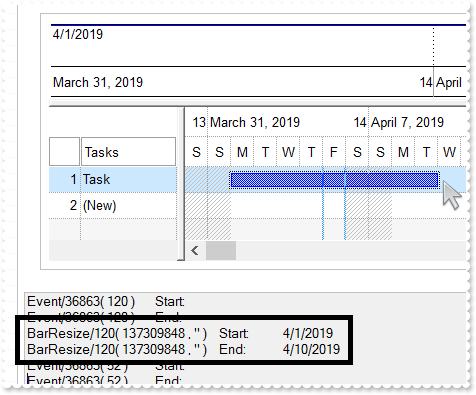
// HostEvent event - Notifies the application once the host fires an event.
void OnHostEventG2Host1(long EventID)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
_variant_t h = spG2Host1->GetHostEventParam(0);
_variant_t key = spG2Host1->GetHostEventParam(1);
OutputDebugStringW( _bstr_t(spG2Host1->GetHostEventParam(-2)) );
OutputDebugStringW( L"Start:" );
OutputDebugStringW( _bstr_t(spG2Host1->GetHost()->GetItems()->GetItemBar(h,key,EXG2ANTTLib::exBarStart)) );
OutputDebugStringW( _bstr_t(spG2Host1->GetHostEventParam(-2)) );
OutputDebugStringW( L"End:" );
OutputDebugStringW( _bstr_t(spG2Host1->GetHost()->GetItems()->GetItemBar(h,key,EXG2ANTTLib::exBarEnd)) );
}
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2ANTTLib' for the library: 'ExG2antt 1.0 Control Library'
#import <ExG2antt.dll>
using namespace EXG2ANTTLib;
*/
EXG2ANTTLib::IChartPtr var_Chart = ((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()))->GetChart();
var_Chart->PutPaneWidth(VARIANT_FALSE,128);
var_Chart->PutFirstVisibleDate(COleDateTime(2019,3,30,0,00,00).operator DATE());
EXG2ANTTLib::IItemsPtr var_Items = ((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()))->GetItems();
var_Items->AddBar(var_Items->AddItem("Task"),"Task",COleDateTime(2019,4,1,0,00,00).operator DATE(),COleDateTime(2019,4,14,0,00,00).operator DATE(),vtMissing,vtMissing);
|
49
|
How do I get the bar/task from the cursor
// HostEvent event - Notifies the application once the host fires an event.
void OnHostEventG2Host1(long EventID)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
OutputDebugStringW( L"Event:" );
OutputDebugStringW( _bstr_t(spG2Host1->GetHostEventParam(-2)) );
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2ANTTLib' for the library: 'ExG2antt 1.0 Control Library'
#import <ExG2antt.dll>
using namespace EXG2ANTTLib;
*/
EXG2ANTTLib::IG2anttPtr var_G2antt = ((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()));
long i = var_G2antt->GetItemFromPoint(-1,-1,c,h);
OutputDebugStringW( L"Cell:" );
OutputDebugStringW( var_G2antt->GetItems()->GetCellCaption(i,c) );
EXG2ANTTLib::IChartPtr var_Chart = var_G2antt->GetChart();
_variant_t b = var_Chart->GetBarFromPoint(-1,1);
OutputDebugStringW( L"Bar:" );
OutputDebugStringW( _bstr_t(((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()))->GetItems()->GetItemBar(i,b,EXG2ANTTLib::exBarName)) );
}
|
48
|
How do I get the cell from the cursor
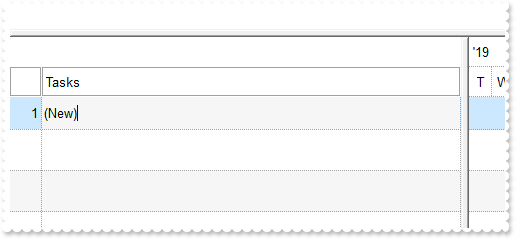
// HostEvent event - Notifies the application once the host fires an event.
void OnHostEventG2Host1(long EventID)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
OutputDebugStringW( L"Event:" );
OutputDebugStringW( _bstr_t(spG2Host1->GetHostEventParam(-2)) );
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2ANTTLib' for the library: 'ExG2antt 1.0 Control Library'
#import <ExG2antt.dll>
using namespace EXG2ANTTLib;
*/
EXG2ANTTLib::IG2anttPtr var_G2antt = ((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()));
long i = var_G2antt->GetItemFromPoint(-1,-1,c,h);
OutputDebugStringW( L"Cell:" );
OutputDebugStringW( var_G2antt->GetItems()->GetCellCaption(i,c) );
}
|
47
|
How can I highlights cells based on its value
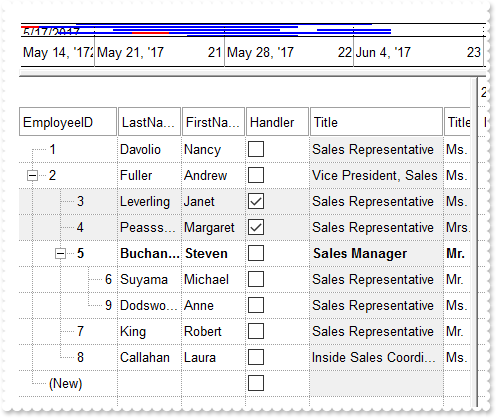
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
spG2Host1->PutDataSource(L"Items","C:\\Program Files\\Exontrol\\ExG2Host\\Sample\\Access\\sample.accdb");
spG2Host1->PutDataMember(L"Items",L"Employees");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsDataSource,L"Items");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsID,L"EmployeeID");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsParentID,L"ReportsTo");
spG2Host1->PutDataSource(L"Tasks",spG2Host1->GetDataSource(L"Items"));
spG2Host1->PutDataMember(L"Tasks",L"EmployeeDetails");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksDataSource,L"Tasks");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksItemID,L"EmployeeID");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksStart,L"DateStart");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksEnd,L"DateEnd");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksID,L"TaskID");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksColor,L"Color");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksCaption,L"TaskName");
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2ANTTLib' for the library: 'ExG2antt 1.0 Control Library'
#import <ExG2antt.dll>
using namespace EXG2ANTTLib;
*/
EXG2ANTTLib::IG2anttPtr var_G2antt = ((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()));
var_G2antt->BeginUpdate();
var_G2antt->PutBackColorAlternate(RGB(0,0,0));
EXG2ANTTLib::IColumnPtr var_Column = var_G2antt->GetColumns()->GetItem("Title");
var_Column->PutDef(EXG2ANTTLib::exCellBackColor,long(15790320));
var_Column->PutWidth(var_Column->GetAutoWidth());
EXG2ANTTLib::IConditionalFormatsPtr var_ConditionalFormats = var_G2antt->GetConditionalFormats();
var_ConditionalFormats->Add(L"lower(%4) contains `manager`",vtMissing)->PutBold(VARIANT_TRUE);
var_ConditionalFormats->Add(L"%3",vtMissing)->PutBackColor(RGB(240,240,240));
var_G2antt->GetItems()->PutExpandItem(0,VARIANT_TRUE);
EXG2ANTTLib::IColumnPtr var_Column1 = var_G2antt->GetColumns()->GetItem("EmployeeID");
var_Column1->PutWidth(var_Column1->GetAutoWidth());
var_G2antt->EndUpdate();
|
46
|
How do I programatically hide a column
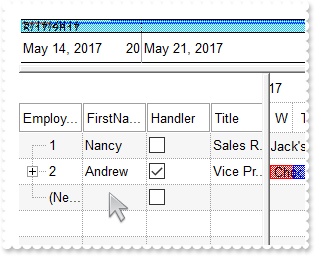
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
spG2Host1->PutDataSource(L"Items","C:\\Program Files\\Exontrol\\ExG2Host\\Sample\\Access\\sample.accdb");
spG2Host1->PutDataMember(L"Items",L"Employees");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsDataSource,L"Items");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsID,L"EmployeeID");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsParentID,L"ReportsTo");
spG2Host1->PutDataSource(L"Tasks",spG2Host1->GetDataSource(L"Items"));
spG2Host1->PutDataMember(L"Tasks",L"EmployeeDetails");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksDataSource,L"Tasks");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksItemID,L"EmployeeID");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksStart,L"DateStart");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksEnd,L"DateEnd");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksID,L"TaskID");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksColor,L"Color");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksCaption,L"TaskName");
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2ANTTLib' for the library: 'ExG2antt 1.0 Control Library'
#import <ExG2antt.dll>
using namespace EXG2ANTTLib;
*/
((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()))->GetColumns()->GetItem("LastName")->PutVisible(VARIANT_FALSE);
|
45
|
Is it possible to get the information from the control when we click on the bar/item
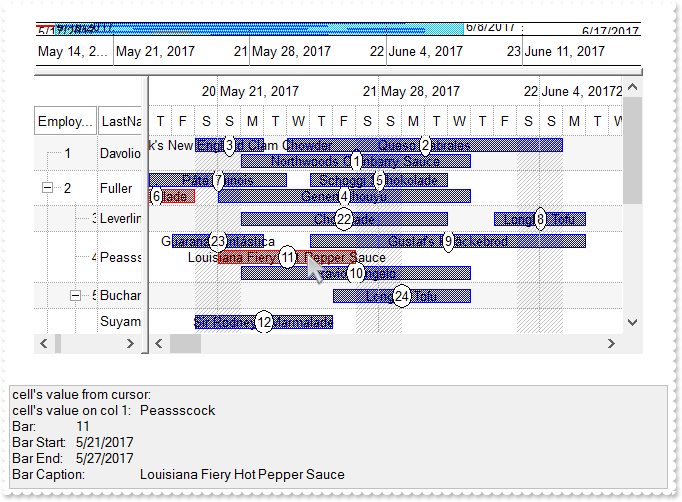
// HostEvent event - Notifies the application once the host fires an event.
void OnHostEventG2Host1(long EventID)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
OutputDebugStringW( _bstr_t(spG2Host1->GetHostEventParam(-2)) );
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2ANTTLib' for the library: 'ExG2antt 1.0 Control Library'
#import <ExG2antt.dll>
using namespace EXG2ANTTLib;
*/
EXG2ANTTLib::IG2anttPtr var_G2antt = ((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()));
long i = var_G2antt->GetItemFromPoint(-1,-1,c,hit);
OutputDebugStringW( L"cell's value from cursor: " );
OutputDebugStringW( _bstr_t(var_G2antt->GetItems()->GetCellValue(i,c)) );
OutputDebugStringW( L"cell's value on col 1: " );
OutputDebugStringW( _bstr_t(var_G2antt->GetItems()->GetCellValue(i,long(1))) );
_variant_t b = var_G2antt->GetChart()->GetBarFromPoint(-1,-1);
OutputDebugStringW( L"Bar:" );
OutputDebugStringW( L"b" );
OutputDebugStringW( L"Bar Start:" );
OutputDebugStringW( _bstr_t(var_G2antt->GetItems()->GetItemBar(i,b,EXG2ANTTLib::exBarStart)) );
OutputDebugStringW( L"Bar End:" );
OutputDebugStringW( _bstr_t(var_G2antt->GetItems()->GetItemBar(i,b,EXG2ANTTLib::exBarEnd)) );
OutputDebugStringW( L"Bar Caption:" );
OutputDebugStringW( _bstr_t(var_G2antt->GetItems()->GetItemBar(i,b,EXG2ANTTLib::exBarCaption)) );
}
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
EXG2ANTTLib::IG2anttPtr var_G2antt = ((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()));
var_G2antt->PutDebug(VARIANT_TRUE);
EXG2ANTTLib::IBarPtr var_Bar = var_G2antt->GetChart()->GetBars()->GetItem("Task");
var_Bar->PutOverlaidType(EXG2ANTTLib::OverlaidBarsTypeEnum(EXG2ANTTLib::exOverlaidBarsStackAutoArrange | EXG2ANTTLib::exOverlaidBarsStack));
var_Bar->PutOverlaidGroup(L"Task,Progress");
spG2Host1->PutDataSource(L"Items","C:\\Program Files\\Exontrol\\ExG2Host\\Sample\\Access\\sample.accdb");
spG2Host1->PutDataMember(L"Items",L"Employees");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsDataSource,L"Items");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsID,L"EmployeeID");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsParentID,L"ReportsTo");
spG2Host1->PutDataSource(L"Tasks",spG2Host1->GetDataSource(L"Items"));
spG2Host1->PutDataMember(L"Tasks",L"EmployeeDetails");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksDataSource,L"Tasks");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksItemID,L"EmployeeID");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksStart,L"DateStart");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksEnd,L"DateEnd");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksID,L"TaskID");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksColor,L"Color");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksCaption,L"TaskName");
((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()))->GetItems()->PutExpandItem(0,VARIANT_TRUE);
EXG2ANTTLib::IChartPtr var_Chart = ((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()))->GetChart();
var_Chart->PutPaneWidth(VARIANT_FALSE,128);
var_Chart->ScrollTo(COleDateTime(2017,5,27,0,00,00).operator DATE(),long(1));
|
44
|
How do I get the bar from the cursor
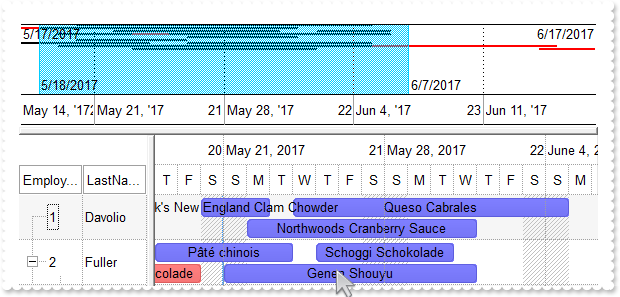
// HostEvent event - Notifies the application once the host fires an event.
void OnHostEventG2Host1(long EventID)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
OutputDebugStringW( _bstr_t(spG2Host1->GetHostEventParam(-2)) );
OutputDebugStringW( _bstr_t(spG2Host1->GetHost()->GetChart()->GetBarFromPoint(-1,-1)) );
}
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2ANTTLib' for the library: 'ExG2antt 1.0 Control Library'
#import <ExG2antt.dll>
using namespace EXG2ANTTLib;
*/
EXG2ANTTLib::IG2anttPtr var_G2antt = ((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()));
var_G2antt->GetVisualAppearance()->Add(1,"C:\\Program Files\\Exontrol\\ExG2Host\\Sample\\EBN\\Assorted\\wbs-ass.ebn");
EXG2ANTTLib::IBarPtr var_Bar = var_G2antt->GetChart()->GetBars()->GetItem("Task");
var_Bar->PutHeight(15);
var_Bar->PutColor(0x1ff0000);
var_Bar->PutOverlaidType(EXG2ANTTLib::OverlaidBarsTypeEnum(EXG2ANTTLib::exOverlaidBarsStackAutoArrange | EXG2ANTTLib::exOverlaidBarsStack));
var_Bar->PutOverlaidGroup(L"Task,Progress");
spG2Host1->PutDataSource(L"Items","C:\\Program Files\\Exontrol\\ExG2Host\\Sample\\Access\\sample.accdb");
spG2Host1->PutDataMember(L"Items",L"Employees");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsDataSource,L"Items");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsID,L"EmployeeID");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsParentID,L"ReportsTo");
spG2Host1->PutDataSource(L"Tasks",spG2Host1->GetDataSource(L"Items"));
spG2Host1->PutDataMember(L"Tasks",L"EmployeeDetails");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksDataSource,L"Tasks");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksItemID,L"EmployeeID");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksStart,L"DateStart");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksEnd,L"DateEnd");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksID,L"TaskID");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksColor,L"Color");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksCaption,L"TaskName");
((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()))->GetItems()->PutExpandItem(0,VARIANT_TRUE);
EXG2ANTTLib::IChartPtr var_Chart = ((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()))->GetChart();
var_Chart->PutPaneWidth(VARIANT_FALSE,128);
var_Chart->ScrollTo(COleDateTime(2017,5,27,0,00,00).operator DATE(),long(1));
|
43
|
How do I add Start/End columns
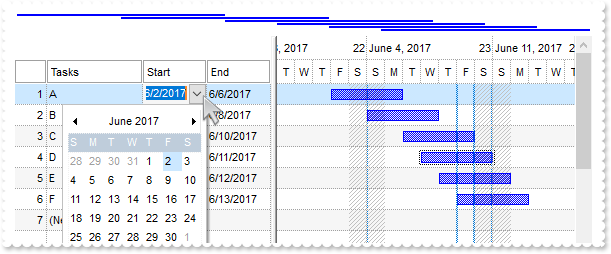
// HostEvent event - Notifies the application once the host fires an event.
void OnHostEventG2Host1(long EventID)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
OutputDebugStringW( _bstr_t(spG2Host1->GetHostEventParam(-2)) );
}
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
spG2Host1->PutHostReadOnly(EXG2HOSTLib::HostReadOnlyEnum(EXG2HOSTLib::exHostReadWrite | EXG2HOSTLib::exHostAllowAddEmptyItem));
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2ANTTLib' for the library: 'ExG2antt 1.0 Control Library'
#import <ExG2antt.dll>
using namespace EXG2ANTTLib;
*/
EXG2ANTTLib::IG2anttPtr var_G2antt = ((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()));
var_G2antt->PutSingleSel(VARIANT_FALSE);
var_G2antt->PutOnResizeControl(EXG2ANTTLib::exResizeChart);
var_G2antt->PutScrollBars(EXG2ANTTLib::ScrollBarsEnum(EXG2ANTTLib::exVScrollEmptySpace | EXG2ANTTLib::exDisableNoVertical));
EXG2ANTTLib::IColumnPtr var_Column = ((EXG2ANTTLib::IColumnPtr)(var_G2antt->GetColumns()->Add(L"Start")));
var_Column->PutAllowSizing(VARIANT_FALSE);
var_Column->PutDef(EXG2ANTTLib::exCellValueToItemBarProperty,long(1));
var_Column->GetEditor()->PutEditType(EXG2ANTTLib::DateType);
EXG2ANTTLib::IColumnPtr var_Column1 = ((EXG2ANTTLib::IColumnPtr)(var_G2antt->GetColumns()->Add(L"End")));
var_Column1->PutAllowSizing(VARIANT_FALSE);
var_Column1->PutDef(EXG2ANTTLib::exCellValueToItemBarProperty,long(2));
var_Column1->GetEditor()->PutEditType(EXG2ANTTLib::DateType);
var_G2antt->GetItems()->PutAllowCellValueToItemBar(VARIANT_TRUE);
EXG2ANTTLib::IChartPtr var_Chart = var_G2antt->GetChart();
var_Chart->PutAllowCreateBar(EXG2ANTTLib::exCreateBarAuto);
var_Chart->PutPaneWidth(VARIANT_FALSE,256);
var_Chart->GetBars()->GetItem("Task")->PutOverlaidType(EXG2ANTTLib::OverlaidBarsTypeEnum(EXG2ANTTLib::exOverlaidBarsStackAutoArrange | EXG2ANTTLib::exOverlaidBarsStack));
|
42
|
How do I hide the left/items/columns part of the control
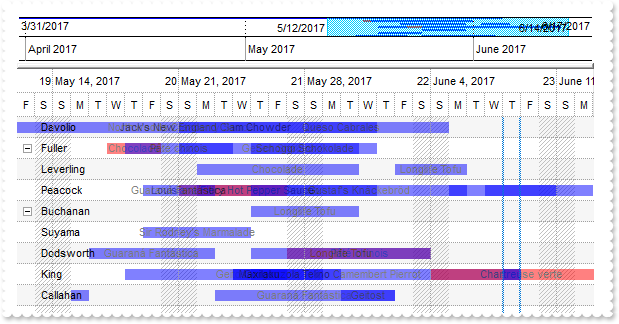
// Error event - Fired when an internal error occurs.
void OnErrorG2Host1(long Error,LPCTSTR Description)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
OutputDebugStringW( L"Error" );
OutputDebugStringW( L"Description" );
}
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2ANTTLib' for the library: 'ExG2antt 1.0 Control Library'
#import <ExG2antt.dll>
using namespace EXG2ANTTLib;
*/
EXG2ANTTLib::IBarPtr var_Bar = ((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()))->GetChart()->GetBars()->GetItem("Task");
var_Bar->PutPattern(EXG2ANTTLib::exPatternSolid);
var_Bar->PutColor(RGB(0,0,255));
var_Bar->PutDef(EXG2ANTTLib::exBarTransparent,long(50));
spG2Host1->PutDataSource(L"Items","C:\\Program Files\\Exontrol\\ExG2Host\\Sample\\Access\\sample.accdb");
spG2Host1->PutDataMember(L"Items",L"Employees");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsDataSource,L"Items");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsID,L"EmployeeID");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsParentID,L"ReportsTo");
spG2Host1->PutDataSource(L"Tasks",spG2Host1->GetDataSource(L"Items"));
spG2Host1->PutDataMember(L"Tasks",L"EmployeeDetails");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksDataSource,L"Tasks");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksItemID,L"EmployeeID");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksStart,L"DateStart");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksEnd,L"DateEnd");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksID,L"TaskID");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksColor,L"Color");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksCaption,L"TaskName");
((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()))->GetItems()->PutExpandItem(0,VARIANT_TRUE);
spG2Host1->PutHostReadOnly(EXG2HOSTLib::HostReadOnlyEnum(EXG2HOSTLib::exHostReadWrite | EXG2HOSTLib::exHostAllowAddEmptyItem));
EXG2ANTTLib::IG2anttPtr var_G2antt = ((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()));
var_G2antt->PutOnResizeControl(EXG2ANTTLib::OnResizeControlEnum(EXG2ANTTLib::exDisableSplitter | EXG2ANTTLib::exResizeChart));
EXG2ANTTLib::IChartPtr var_Chart = var_G2antt->GetChart();
var_Chart->PutColumnsFormatLevel(L"1");
var_Chart->PutPaneWidth(VARIANT_FALSE,0);
var_Chart->ScrollTo(COleDateTime(2017,5,27,0,00,00).operator DATE(),long(1));
|
41
|
How do I hide the right/chart/tasks part of the control
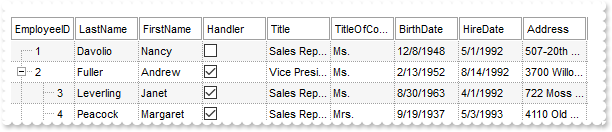
// Error event - Fired when an internal error occurs.
void OnErrorG2Host1(long Error,LPCTSTR Description)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
OutputDebugStringW( L"Error" );
OutputDebugStringW( L"Description" );
}
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2ANTTLib' for the library: 'ExG2antt 1.0 Control Library'
#import <ExG2antt.dll>
using namespace EXG2ANTTLib;
*/
EXG2ANTTLib::IBarPtr var_Bar = ((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()))->GetChart()->GetBars()->GetItem("Task");
var_Bar->PutPattern(EXG2ANTTLib::exPatternSolid);
var_Bar->PutColor(RGB(0,0,255));
var_Bar->PutDef(EXG2ANTTLib::exBarTransparent,long(50));
spG2Host1->PutDataSource(L"Items","C:\\Program Files\\Exontrol\\ExG2Host\\Sample\\Access\\sample.accdb");
spG2Host1->PutDataMember(L"Items",L"Employees");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsDataSource,L"Items");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsID,L"EmployeeID");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsParentID,L"ReportsTo");
spG2Host1->PutDataSource(L"Tasks",spG2Host1->GetDataSource(L"Items"));
spG2Host1->PutDataMember(L"Tasks",L"EmployeeDetails");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksDataSource,L"Tasks");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksItemID,L"EmployeeID");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksStart,L"DateStart");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksEnd,L"DateEnd");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksID,L"TaskID");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksColor,L"Color");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksCaption,L"TaskName");
((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()))->GetItems()->PutExpandItem(0,VARIANT_TRUE);
EXG2ANTTLib::IG2anttPtr var_G2antt = ((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()));
EXG2ANTTLib::IChartPtr var_Chart = var_G2antt->GetChart();
var_Chart->PutPaneWidth(VARIANT_TRUE,0);
var_Chart->PutOverviewVisible(EXG2ANTTLib::exOverviewHidden);
|
40
|
How do hide the top/overview part of the control
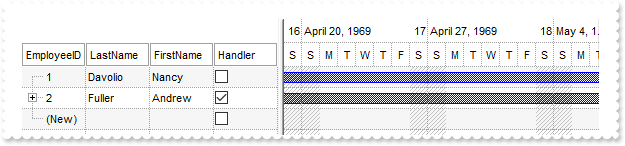
// Error event - Fired when an internal error occurs.
void OnErrorG2Host1(long Error,LPCTSTR Description)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
OutputDebugStringW( L"Error" );
OutputDebugStringW( L"Description" );
}
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
spG2Host1->PutDataSource(L"Items","C:\\Program Files\\Exontrol\\ExG2Host\\Sample\\Access\\sample.accdb");
spG2Host1->PutDataMember(L"Items",L"Employees");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsDataSource,L"Items");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsID,L"EmployeeID");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsParentID,L"ReportsTo");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksDataSource,spG2Host1->GetDataField(EXG2HOSTLib::exItemsDataSource));
spG2Host1->PutDataField(EXG2HOSTLib::exTasksStart,L"BirthDate");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksEnd,L"HireDate");
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2ANTTLib' for the library: 'ExG2antt 1.0 Control Library'
#import <ExG2antt.dll>
using namespace EXG2ANTTLib;
*/
EXG2ANTTLib::IG2anttPtr var_G2antt = ((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()));
EXG2ANTTLib::IChartPtr var_Chart = var_G2antt->GetChart();
var_Chart->PutOverviewVisible(EXG2ANTTLib::exOverviewHidden);
var_Chart->PutPaneWidth(VARIANT_FALSE,256);
var_Chart->ScrollTo(COleDateTime(1969,4,27,0,00,00).operator DATE(),long(1));
|
39
|
How do I resize the panels
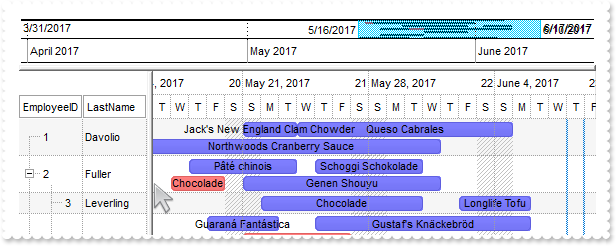
// Error event - Fired when an internal error occurs.
void OnErrorG2Host1(long Error,LPCTSTR Description)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
OutputDebugStringW( L"Error" );
OutputDebugStringW( L"Description" );
}
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2ANTTLib' for the library: 'ExG2antt 1.0 Control Library'
#import <ExG2antt.dll>
using namespace EXG2ANTTLib;
*/
EXG2ANTTLib::IG2anttPtr var_G2antt = ((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()));
var_G2antt->GetVisualAppearance()->Add(1,"C:\\Program Files\\Exontrol\\ExG2Host\\Sample\\EBN\\Assorted\\wbs-ass.ebn");
EXG2ANTTLib::IBarPtr var_Bar = var_G2antt->GetChart()->GetBars()->GetItem("Task");
var_Bar->PutHeight(15);
var_Bar->PutColor(0x1ff0000);
var_Bar->PutOverlaidType(EXG2ANTTLib::OverlaidBarsTypeEnum(EXG2ANTTLib::exOverlaidBarsStackAutoArrange | EXG2ANTTLib::exOverlaidBarsStack));
var_Bar->PutOverlaidGroup(L"Task,Progress");
spG2Host1->PutDataSource(L"Items","C:\\Program Files\\Exontrol\\ExG2Host\\Sample\\Access\\sample.accdb");
spG2Host1->PutDataMember(L"Items",L"Employees");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsDataSource,L"Items");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsID,L"EmployeeID");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsParentID,L"ReportsTo");
spG2Host1->PutDataSource(L"Tasks",spG2Host1->GetDataSource(L"Items"));
spG2Host1->PutDataMember(L"Tasks",L"EmployeeDetails");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksDataSource,L"Tasks");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksItemID,L"EmployeeID");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksStart,L"DateStart");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksEnd,L"DateEnd");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksID,L"TaskID");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksColor,L"Color");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksCaption,L"TaskName");
((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()))->GetItems()->PutExpandItem(0,VARIANT_TRUE);
EXG2ANTTLib::IChartPtr var_Chart = ((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()))->GetChart();
var_Chart->PutPaneWidth(VARIANT_FALSE,128);
var_Chart->ScrollTo(COleDateTime(2017,5,27,0,00,00).operator DATE(),long(1));
|
38
|
How do I lock the first column
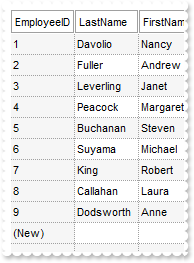
// Error event - Fired when an internal error occurs.
void OnErrorG2Host1(long Error,LPCTSTR Description)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
OutputDebugStringW( L"Error" );
OutputDebugStringW( L"Description" );
}
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
spG2Host1->PutDataSource(L"Items","C:\\Program Files\\Exontrol\\ExG2Host\\Sample\\Access\\sample.accdb");
spG2Host1->PutDataMember(L"Items",L"Employees");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsDataSource,L"Items");
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2ANTTLib' for the library: 'ExG2antt 1.0 Control Library'
#import <ExG2antt.dll>
using namespace EXG2ANTTLib;
*/
EXG2ANTTLib::IG2anttPtr var_G2antt = ((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()));
var_G2antt->PutCountLockedColumns(1);
var_G2antt->PutBackColorLock(var_G2antt->GetBackColorAlternate());
|
37
|
How do I specify a different color for the tasks ( EBN color )
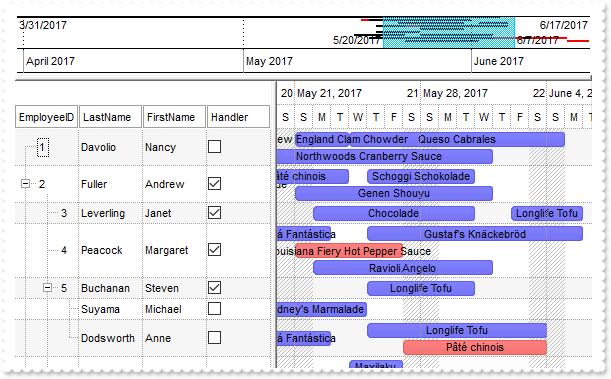
// Error event - Fired when an internal error occurs.
void OnErrorG2Host1(long Error,LPCTSTR Description)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
OutputDebugStringW( L"Error" );
OutputDebugStringW( L"Description" );
}
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2ANTTLib' for the library: 'ExG2antt 1.0 Control Library'
#import <ExG2antt.dll>
using namespace EXG2ANTTLib;
*/
EXG2ANTTLib::IG2anttPtr var_G2antt = ((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()));
var_G2antt->GetVisualAppearance()->Add(1,"C:\\Program Files\\Exontrol\\ExG2Host\\Sample\\EBN\\Assorted\\wbs-ass.ebn");
EXG2ANTTLib::IBarPtr var_Bar = var_G2antt->GetChart()->GetBars()->GetItem("Task");
var_Bar->PutHeight(15);
var_Bar->PutColor(0x1ff0000);
var_Bar->PutOverlaidType(EXG2ANTTLib::OverlaidBarsTypeEnum(EXG2ANTTLib::exOverlaidBarsStackAutoArrange | EXG2ANTTLib::exOverlaidBarsStack));
var_Bar->PutOverlaidGroup(L"Task,Progress");
spG2Host1->PutDataSource(L"Items","C:\\Program Files\\Exontrol\\ExG2Host\\Sample\\Access\\sample.accdb");
spG2Host1->PutDataMember(L"Items",L"Employees");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsDataSource,L"Items");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsID,L"EmployeeID");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsParentID,L"ReportsTo");
spG2Host1->PutDataSource(L"Tasks",spG2Host1->GetDataSource(L"Items"));
spG2Host1->PutDataMember(L"Tasks",L"EmployeeDetails");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksDataSource,L"Tasks");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksItemID,L"EmployeeID");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksStart,L"DateStart");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksEnd,L"DateEnd");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksID,L"TaskID");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksColor,L"Color");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksCaption,L"TaskName");
((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()))->GetItems()->PutExpandItem(0,VARIANT_TRUE);
((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()))->GetChart()->PutPaneWidth(VARIANT_FALSE,256);
((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()))->GetChart()->ScrollTo(COleDateTime(2017,5,27,0,00,00).operator DATE(),long(1));
|
36
|
How do I specify a different color for the tasks ( solid color, transparent )
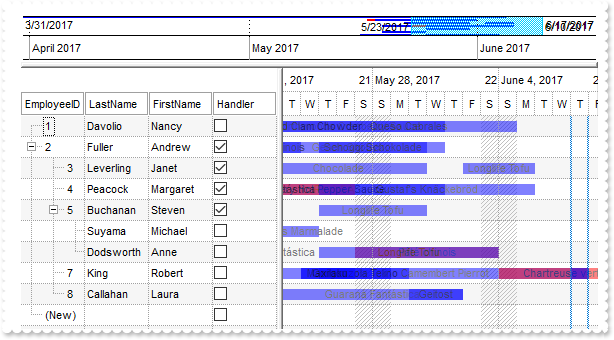
// Error event - Fired when an internal error occurs.
void OnErrorG2Host1(long Error,LPCTSTR Description)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
OutputDebugStringW( L"Error" );
OutputDebugStringW( L"Description" );
}
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2ANTTLib' for the library: 'ExG2antt 1.0 Control Library'
#import <ExG2antt.dll>
using namespace EXG2ANTTLib;
*/
EXG2ANTTLib::IBarPtr var_Bar = ((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()))->GetChart()->GetBars()->GetItem("Task");
var_Bar->PutPattern(EXG2ANTTLib::exPatternSolid);
var_Bar->PutColor(RGB(0,0,255));
var_Bar->PutDef(EXG2ANTTLib::exBarTransparent,long(50));
spG2Host1->PutDataSource(L"Items","C:\\Program Files\\Exontrol\\ExG2Host\\Sample\\Access\\sample.accdb");
spG2Host1->PutDataMember(L"Items",L"Employees");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsDataSource,L"Items");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsID,L"EmployeeID");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsParentID,L"ReportsTo");
spG2Host1->PutDataSource(L"Tasks",spG2Host1->GetDataSource(L"Items"));
spG2Host1->PutDataMember(L"Tasks",L"EmployeeDetails");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksDataSource,L"Tasks");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksItemID,L"EmployeeID");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksStart,L"DateStart");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksEnd,L"DateEnd");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksID,L"TaskID");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksColor,L"Color");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksCaption,L"TaskName");
((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()))->GetItems()->PutExpandItem(0,VARIANT_TRUE);
((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()))->GetChart()->PutPaneWidth(VARIANT_FALSE,256);
((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()))->GetChart()->ScrollTo(COleDateTime(2017,5,27,0,00,00).operator DATE(),long(1));
|
35
|
GroupBy
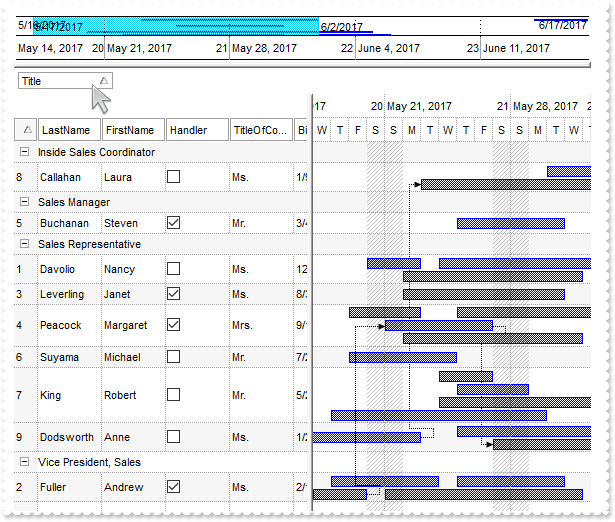
// Error event - Fired when an internal error occurs.
void OnErrorG2Host1(long Error,LPCTSTR Description)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
OutputDebugStringW( L"Error" );
OutputDebugStringW( L"Description" );
}
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
spG2Host1->PutDataSource(L"Items","C:\\Program Files\\Exontrol\\ExG2Host\\Sample\\Access\\sample.accdb");
spG2Host1->PutDataMember(L"Items",L"Employees");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsDataSource,L"Items");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsID,L"EmployeeID");
spG2Host1->PutDataSource(L"Tasks",spG2Host1->GetDataSource(L"Items"));
spG2Host1->PutDataMember(L"Tasks",L"EmployeeDetails");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksDataSource,L"Tasks");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksItemID,L"EmployeeID");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksStart,L"DateStart");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksEnd,L"DateEnd");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksID,L"TaskID");
spG2Host1->PutDataSource(L"Links",spG2Host1->GetDataSource(L"Items"));
spG2Host1->PutDataMember(L"Links",L"EmployeeLinks");
spG2Host1->PutDataField(EXG2HOSTLib::exLinksDataSource,L"Links");
spG2Host1->PutDataField(EXG2HOSTLib::exLinksStart,L"Start");
spG2Host1->PutDataField(EXG2HOSTLib::exLinksEnd,L"End");
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2ANTTLib' for the library: 'ExG2antt 1.0 Control Library'
#import <ExG2antt.dll>
using namespace EXG2ANTTLib;
*/
EXG2ANTTLib::IG2anttPtr var_G2antt = ((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()));
var_G2antt->PutAllowGroupBy(VARIANT_TRUE);
var_G2antt->PutSortBarVisible(VARIANT_TRUE);
var_G2antt->PutBackColorSortBar(((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()))->GetBackColor());
var_G2antt->PutBackColorSortBarCaption(var_G2antt->GetBackColorSortBar());
var_G2antt->PutSortBarCaption(L"<sha ;;0><fgcolor=FF0000>Drag a <b>column</b> header here to sort by that column.");
EXG2ANTTLib::IBarPtr var_Bar = var_G2antt->GetChart()->GetBars()->GetItem("Task");
var_Bar->PutOverlaidType(EXG2ANTTLib::OverlaidBarsTypeEnum(EXG2ANTTLib::exOverlaidBarsStackAutoArrange | EXG2ANTTLib::exOverlaidBarsStack));
var_Bar->PutOverlaidGroup(L"Task,Progress");
var_G2antt->GetItems()->PutExpandItem(0,VARIANT_TRUE);
var_G2antt->PutCountLockedColumns(1);
var_G2antt->PutBackColorLock(var_G2antt->GetBackColorAlternate());
EXG2ANTTLib::IColumnPtr var_Column = var_G2antt->GetColumns()->GetItem("EmployeeID");
var_Column->PutAllowGroupBy(VARIANT_FALSE);
var_Column->PutDef(EXG2ANTTLib::exHeaderBackColor,((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()))->GetBackColorAlternate());
var_G2antt->GetColumns()->GetItem("Title")->PutSortOrder(EXG2ANTTLib::SortAscending);
((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()))->GetChart()->ScrollTo(COleDateTime(2017,5,27,0,00,00).operator DATE(),long(1));
|
34
|
How can I hide a column
// Error event - Fired when an internal error occurs.
void OnErrorG2Host1(long Error,LPCTSTR Description)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
OutputDebugStringW( L"Error" );
OutputDebugStringW( L"Description" );
}
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
spG2Host1->PutDataSource(L"Items","C:\\Program Files\\Exontrol\\ExG2Host\\Sample\\Access\\sample.accdb");
spG2Host1->PutDataMember(L"Items",L"Employees");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsDataSource,L"Items");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsID,L"EmployeeID");
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2ANTTLib' for the library: 'ExG2antt 1.0 Control Library'
#import <ExG2antt.dll>
using namespace EXG2ANTTLib;
*/
((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()))->GetColumns()->GetItem(long(0))->PutVisible(VARIANT_FALSE);
|
33
|
Can row errors being highligted until the user correct them, not to clear them as soon a change occurs
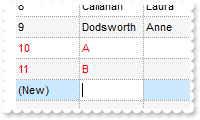
// Error event - Fired when an internal error occurs.
void OnErrorG2Host1(long Error,LPCTSTR Description)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
OutputDebugStringW( L"Error" );
OutputDebugStringW( L"Description" );
}
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
spG2Host1->PutDataSource(L"Items","C:\\Program Files\\Exontrol\\ExG2Host\\Sample\\Access\\sample.accdb");
spG2Host1->PutDataMember(L"Items",L"Employees");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsDataSource,L"Items");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsID,L"EmployeeID");
spG2Host1->PutHostDef(EXG2HOSTLib::exErrorClearOnChange,VARIANT_FALSE);
|
32
|
No error is highligthed
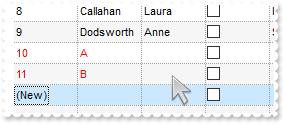
// Error event - Fired when an internal error occurs.
void OnErrorG2Host1(long Error,LPCTSTR Description)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
OutputDebugStringW( L"Error" );
OutputDebugStringW( L"Description" );
}
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
spG2Host1->PutDataSource(L"Items","C:\\Program Files\\Exontrol\\ExG2Host\\Sample\\Access\\sample.accdb");
spG2Host1->PutDataMember(L"Items",L"Employees");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsDataSource,L"Items");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsID,L"EmployeeID");
|
31
|
I've noticed that rows with errors are shown in red. Is it possible to change the colors
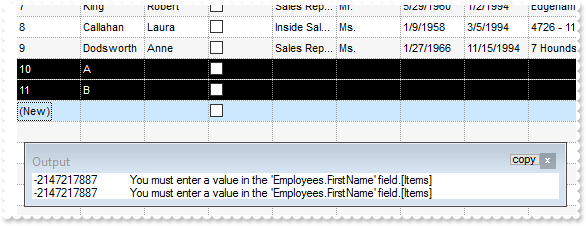
// Error event - Fired when an internal error occurs.
void OnErrorG2Host1(long Error,LPCTSTR Description)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
OutputDebugStringW( L"Error" );
OutputDebugStringW( L"Description" );
}
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
spG2Host1->PutDataSource(L"Items","C:\\Program Files\\Exontrol\\ExG2Host\\Sample\\Access\\sample.accdb");
spG2Host1->PutDataMember(L"Items",L"Employees");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsDataSource,L"Items");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsID,L"EmployeeID");
spG2Host1->PutHostDef(EXG2HOSTLib::exErrorBackColor,long(0));
spG2Host1->PutHostDef(EXG2HOSTLib::exErrorForeColor,long(16777215));
|
30
|
Is it possible to rename the (New) to something else
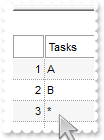
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
spG2Host1->PutHostDef(EXG2HOSTLib::exNew,"*");
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2ANTTLib' for the library: 'ExG2antt 1.0 Control Library'
#import <ExG2antt.dll>
using namespace EXG2ANTTLib;
*/
EXG2ANTTLib::IG2anttPtr var_G2antt = ((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()));
var_G2antt->BeginUpdate();
var_G2antt->GetItems()->AddItem("A");
var_G2antt->GetItems()->AddItem("B");
var_G2antt->EndUpdate();
spG2Host1->Refresh();
|
29
|
How can I hide the (New) item (sample 2)
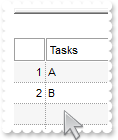
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
spG2Host1->PutHostReadOnly(EXG2HOSTLib::HostReadOnlyEnum(EXG2HOSTLib::exHostAllowUpdate | EXG2HOSTLib::exHostAllowDelete));
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2ANTTLib' for the library: 'ExG2antt 1.0 Control Library'
#import <ExG2antt.dll>
using namespace EXG2ANTTLib;
*/
EXG2ANTTLib::IG2anttPtr var_G2antt = ((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()));
var_G2antt->BeginUpdate();
var_G2antt->GetItems()->AddItem("A");
var_G2antt->GetItems()->AddItem("B");
var_G2antt->EndUpdate();
|
28
|
How can I hide the (New) item (sample 1)
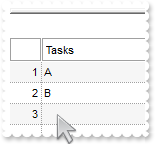
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
spG2Host1->PutHostDef(EXG2HOSTLib::exNew,"");
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2ANTTLib' for the library: 'ExG2antt 1.0 Control Library'
#import <ExG2antt.dll>
using namespace EXG2ANTTLib;
*/
EXG2ANTTLib::IG2anttPtr var_G2antt = ((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()));
var_G2antt->BeginUpdate();
var_G2antt->GetItems()->AddItem("A");
var_G2antt->GetItems()->AddItem("B");
var_G2antt->EndUpdate();
spG2Host1->Refresh();
|
27
|
Read-Only
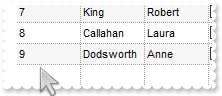
// Error event - Fired when an internal error occurs.
void OnErrorG2Host1(long Error,LPCTSTR Description)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
OutputDebugStringW( L"Error" );
OutputDebugStringW( L"Description" );
}
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
spG2Host1->PutDataSource(L"Items","C:\\Program Files\\Exontrol\\ExG2Host\\Sample\\Access\\sample.accdb");
spG2Host1->PutDataMember(L"Items",L"Employees");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsDataSource,L"Items");
spG2Host1->PutHostReadOnly(EXG2HOSTLib::exHostReadOnly);
|
26
|
How can I prevent user create new /delete tasks ( only move or resize then )
// Error event - Fired when an internal error occurs.
void OnErrorG2Host1(long Error,LPCTSTR Description)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
OutputDebugStringW( L"Error" );
OutputDebugStringW( L"Description" );
}
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
spG2Host1->PutDataSource(L"Items","C:\\Program Files\\Exontrol\\ExG2Host\\Sample\\Access\\sample.accdb");
spG2Host1->PutDataMember(L"Items",L"Employees");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsDataSource,L"Items");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsID,L"EmployeeID");
spG2Host1->PutDataSource(L"Tasks",spG2Host1->GetDataSource(L"Items"));
spG2Host1->PutDataMember(L"Tasks",L"EmployeeDetails");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksDataSource,L"Tasks");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksItemID,L"EmployeeID");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksStart,L"DateStart");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksEnd,L"DateEnd");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksID,L"TaskID");
spG2Host1->PutDataSource(L"Links",spG2Host1->GetDataSource(L"Items"));
spG2Host1->PutDataMember(L"Links",L"EmployeeLinks");
spG2Host1->PutDataField(EXG2HOSTLib::exLinksDataSource,L"Links");
spG2Host1->PutDataField(EXG2HOSTLib::exLinksStart,L"Start");
spG2Host1->PutDataField(EXG2HOSTLib::exLinksEnd,L"End");
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2ANTTLib' for the library: 'ExG2antt 1.0 Control Library'
#import <ExG2antt.dll>
using namespace EXG2ANTTLib;
*/
EXG2ANTTLib::IBarPtr var_Bar = ((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()))->GetChart()->GetBars()->GetItem("Task");
var_Bar->PutOverlaidType(EXG2ANTTLib::OverlaidBarsTypeEnum(EXG2ANTTLib::exOverlaidBarsStackAutoArrange | EXG2ANTTLib::exOverlaidBarsStack));
var_Bar->PutOverlaidGroup(L"Task,Progress");
((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()))->GetItems()->PutExpandItem(0,VARIANT_TRUE);
spG2Host1->PutHostReadOnly(EXG2HOSTLib::exHostAllowUpdate);
((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()))->GetChart()->ScrollTo(COleDateTime(2017,5,27,0,00,00).operator DATE(),long(1));
|
25
|
How do I get the row/item/task/link from the cursor
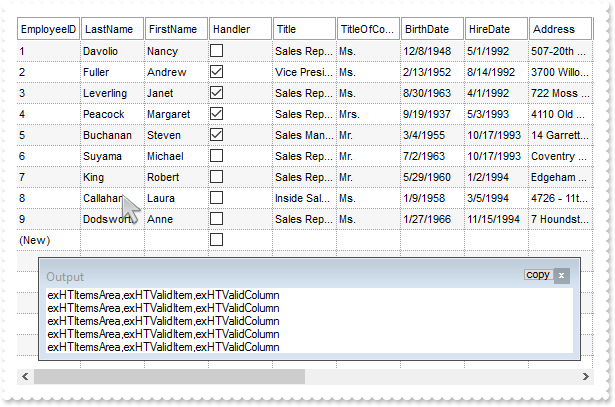
// Error event - Fired when an internal error occurs.
void OnErrorG2Host1(long Error,LPCTSTR Description)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
OutputDebugStringW( L"Error" );
OutputDebugStringW( L"Description" );
}
// HostEvent event - Notifies the application once the host fires an event.
void OnHostEventG2Host1(long EventID)
{
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
OutputDebugStringW( spG2Host1->GetHostContext()->GetToString() );
}
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
spG2Host1->PutDataSource(L"Items","C:\\Program Files\\Exontrol\\ExG2Host\\Sample\\Access\\sample.accdb");
spG2Host1->PutDataMember(L"Items",L"Employees");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsDataSource,L"Items");
spG2Host1->PutHostReadOnly(EXG2HOSTLib::HostReadOnlyEnum(EXG2HOSTLib::exHostAllowUpdate | EXG2HOSTLib::exHostAllowAddNew));
|
24
|
Disable Delete
// Error event - Fired when an internal error occurs.
void OnErrorG2Host1(long Error,LPCTSTR Description)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
OutputDebugStringW( L"Error" );
OutputDebugStringW( L"Description" );
}
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
spG2Host1->PutDataSource(L"Items","C:\\Program Files\\Exontrol\\ExG2Host\\Sample\\Access\\sample.accdb");
spG2Host1->PutDataMember(L"Items",L"Employees");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsDataSource,L"Items");
spG2Host1->PutHostReadOnly(EXG2HOSTLib::HostReadOnlyEnum(EXG2HOSTLib::exHostAllowUpdate | EXG2HOSTLib::exHostAllowAddNew));
|
23
|
Disable AddNew
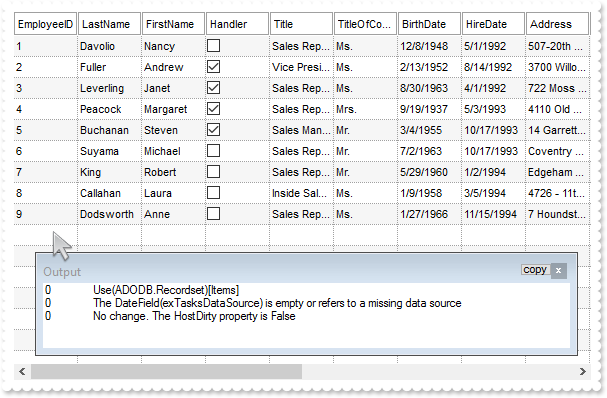
// Error event - Fired when an internal error occurs.
void OnErrorG2Host1(long Error,LPCTSTR Description)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
OutputDebugStringW( L"Error" );
OutputDebugStringW( L"Description" );
}
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
spG2Host1->PutDataSource(L"Items","C:\\Program Files\\Exontrol\\ExG2Host\\Sample\\Access\\sample.accdb");
spG2Host1->PutDataMember(L"Items",L"Employees");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsDataSource,L"Items");
spG2Host1->PutHostReadOnly(EXG2HOSTLib::HostReadOnlyEnum(EXG2HOSTLib::exHostAllowUpdate | EXG2HOSTLib::exHostAllowDelete));
|
22
|
ACCDB sample ( file )
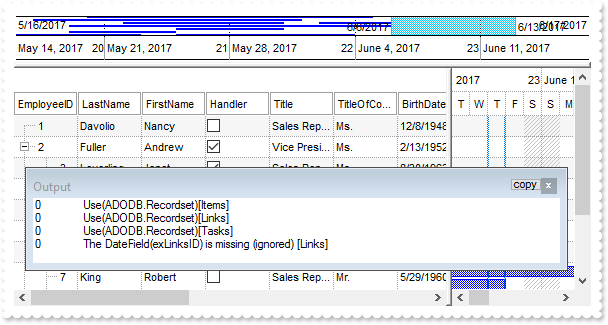
// Error event - Fired when an internal error occurs.
void OnErrorG2Host1(long Error,LPCTSTR Description)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
OutputDebugStringW( L"Error" );
OutputDebugStringW( L"Description" );
}
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
spG2Host1->PutDataSource(L"Items","C:\\Program Files\\Exontrol\\ExG2Host\\Sample\\Access\\sample.accdb");
spG2Host1->PutDataMember(L"Items",L"Employees");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsDataSource,L"Items");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsID,L"EmployeeID");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsParentID,L"ReportsTo");
spG2Host1->PutDataSource(L"Tasks",spG2Host1->GetDataSource(L"Items"));
spG2Host1->PutDataMember(L"Tasks",L"EmployeeDetails");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksDataSource,L"Tasks");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksItemID,L"EmployeeID");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksStart,L"DateStart");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksEnd,L"DateEnd");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksID,L"TaskID");
spG2Host1->PutDataSource(L"Links",spG2Host1->GetDataSource(L"Items"));
spG2Host1->PutDataMember(L"Links",L"EmployeeLinks");
spG2Host1->PutDataField(EXG2HOSTLib::exLinksDataSource,L"Links");
spG2Host1->PutDataField(EXG2HOSTLib::exLinksStart,L"Start");
spG2Host1->PutDataField(EXG2HOSTLib::exLinksEnd,L"End");
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2ANTTLib' for the library: 'ExG2antt 1.0 Control Library'
#import <ExG2antt.dll>
using namespace EXG2ANTTLib;
*/
EXG2ANTTLib::IBarPtr var_Bar = ((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()))->GetChart()->GetBars()->GetItem("Task");
var_Bar->PutOverlaidType(EXG2ANTTLib::OverlaidBarsTypeEnum(EXG2ANTTLib::exOverlaidBarsStackAutoArrange | EXG2ANTTLib::exOverlaidBarsStack));
var_Bar->PutOverlaidGroup(L"Task,Progress");
((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()))->GetItems()->PutExpandItem(0,VARIANT_TRUE);
((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()))->GetChart()->ScrollTo(COleDateTime(2017,5,27,0,00,00).operator DATE(),long(1));
|
21
|
MDB sample ( file )
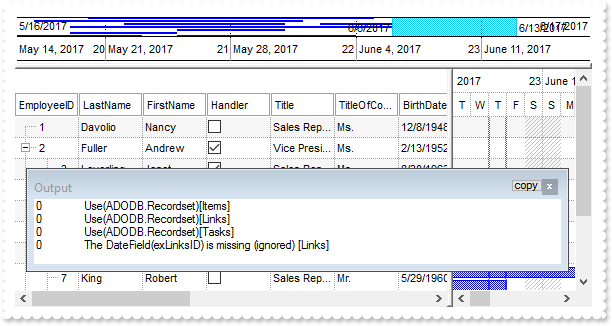
// Error event - Fired when an internal error occurs.
void OnErrorG2Host1(long Error,LPCTSTR Description)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
OutputDebugStringW( L"Error" );
OutputDebugStringW( L"Description" );
}
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
spG2Host1->PutDataSource(L"Items","C:\\Program Files\\Exontrol\\ExG2Host\\Sample\\sample.mdb");
spG2Host1->PutDataMember(L"Items",L"Employees");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsDataSource,L"Items");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsID,L"EmployeeID");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsParentID,L"ReportsTo");
spG2Host1->PutDataSource(L"Tasks",spG2Host1->GetDataSource(L"Items"));
spG2Host1->PutDataMember(L"Tasks",L"EmployeeDetails");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksDataSource,L"Tasks");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksItemID,L"EmployeeID");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksStart,L"DateStart");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksEnd,L"DateEnd");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksID,L"TaskID");
spG2Host1->PutDataSource(L"Links",spG2Host1->GetDataSource(L"Items"));
spG2Host1->PutDataMember(L"Links",L"EmployeeLinks");
spG2Host1->PutDataField(EXG2HOSTLib::exLinksDataSource,L"Links");
spG2Host1->PutDataField(EXG2HOSTLib::exLinksStart,L"Start");
spG2Host1->PutDataField(EXG2HOSTLib::exLinksEnd,L"End");
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2ANTTLib' for the library: 'ExG2antt 1.0 Control Library'
#import <ExG2antt.dll>
using namespace EXG2ANTTLib;
*/
EXG2ANTTLib::IBarPtr var_Bar = ((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()))->GetChart()->GetBars()->GetItem("Task");
var_Bar->PutOverlaidType(EXG2ANTTLib::OverlaidBarsTypeEnum(EXG2ANTTLib::exOverlaidBarsStackAutoArrange | EXG2ANTTLib::exOverlaidBarsStack));
var_Bar->PutOverlaidGroup(L"Task,Progress");
((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()))->GetItems()->PutExpandItem(0,VARIANT_TRUE);
((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()))->GetChart()->ScrollTo(COleDateTime(2017,5,27,0,00,00).operator DATE(),long(1));
|
20
|
DBF sample ( file )
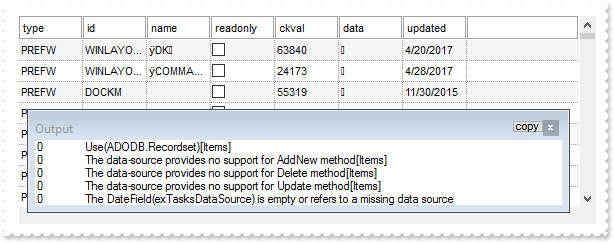
// Error event - Fired when an internal error occurs.
void OnErrorG2Host1(long Error,LPCTSTR Description)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
OutputDebugStringW( L"Error" );
OutputDebugStringW( L"Description" );
}
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
spG2Host1->PutDataSource(L"Items","C:\\Program Files\\Exontrol\\ExG2Host\\Sample\\sample.dbf");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsDataSource,L"Items");
|
19
|
DAO sample ( object, DAO.DBEngine.120, multiple tasks, multiple tables )
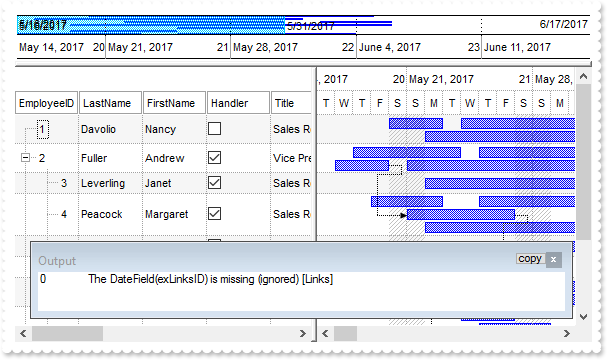
// Error event - Fired when an internal error occurs.
void OnErrorG2Host1(long Error,LPCTSTR Description)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
OutputDebugStringW( L"Error" );
OutputDebugStringW( L"Description" );
}
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'DAO' for the library: 'Microsoft Office 15.0 Access database engine Object Library'
#import <ACEDAO.DLL>
*/
DAO::_DBEnginePtr var_PrivDBEngine = ::CreateObject(L"DAO.DBEngine.120");
DAO::DatabasePtr var_Database = var_PrivDBEngine->OpenDatabase(L"C:\\Program Files\\Exontrol\\ExG2Host\\Sample\\Access\\sample.accdb",vtMissing,vtMissing,vtMissing);
DAO::Recordset2Ptr rsEmployees = ((DAO::Recordset2Ptr)(var_Database->OpenRecordset(L"Employees",vtMissing,vtMissing,vtMissing)));
DAO::Recordset2Ptr rsTasks = ((DAO::Recordset2Ptr)(var_Database->OpenRecordset(L"EmployeeDetails",vtMissing,vtMissing,vtMissing)));
DAO::Recordset2Ptr rsLinks = ((DAO::Recordset2Ptr)(var_Database->OpenRecordset(L"EmployeeLinks",vtMissing,vtMissing,vtMissing)));
spG2Host1->PutDataSource(L"Items",((DAO::Recordset2Ptr)(rsEmployees)));
spG2Host1->PutDataMember(L"Items",L"Employees");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsDataSource,L"Items");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsID,L"EmployeeID");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsParentID,L"ReportsTo");
spG2Host1->PutDataSource(L"Tasks",((DAO::Recordset2Ptr)(rsTasks)));
spG2Host1->PutDataField(EXG2HOSTLib::exTasksDataSource,L"Tasks");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksItemID,L"EmployeeID");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksStart,L"DateStart");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksEnd,L"DateEnd");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksID,L"TaskID");
spG2Host1->PutDataSource(L"Links",((DAO::Recordset2Ptr)(rsLinks)));
spG2Host1->PutDataField(EXG2HOSTLib::exLinksDataSource,L"Links");
spG2Host1->PutDataField(EXG2HOSTLib::exLinksStart,L"Start");
spG2Host1->PutDataField(EXG2HOSTLib::exLinksEnd,L"End");
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2ANTTLib' for the library: 'ExG2antt 1.0 Control Library'
#import <ExG2antt.dll>
using namespace EXG2ANTTLib;
*/
EXG2ANTTLib::IBarPtr var_Bar = ((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()))->GetChart()->GetBars()->GetItem("Task");
var_Bar->PutOverlaidType(EXG2ANTTLib::OverlaidBarsTypeEnum(EXG2ANTTLib::exOverlaidBarsStackAutoArrange | EXG2ANTTLib::exOverlaidBarsStack));
var_Bar->PutOverlaidGroup(L"Task,Progress");
((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()))->GetItems()->PutExpandItem(0,VARIANT_TRUE);
((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()))->GetChart()->ScrollTo(COleDateTime(2017,5,27,0,00,00).operator DATE(),long(1));
|
18
|
DAO sample ( file, multiple tasks, multiple tables )
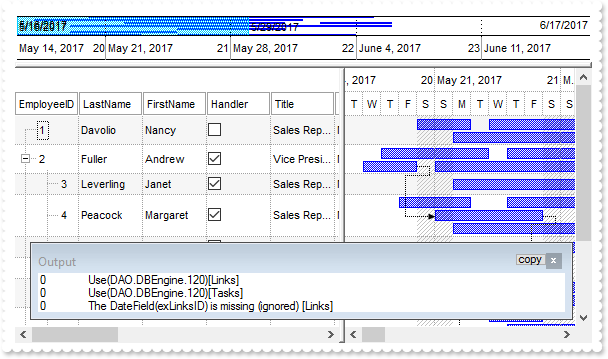
// Error event - Fired when an internal error occurs.
void OnErrorG2Host1(long Error,LPCTSTR Description)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
OutputDebugStringW( L"Error" );
OutputDebugStringW( L"Description" );
}
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
spG2Host1->PutDataTechnology(L"Items",L"DAO.DBEngine.120;DAO.DBEngine.36");
spG2Host1->PutDataSource(L"Items","C:\\Program Files\\Exontrol\\ExG2Host\\Sample\\Access\\sample.accdb");
spG2Host1->PutDataMember(L"Items",L"Employees");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsDataSource,L"Items");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsID,L"EmployeeID");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsParentID,L"ReportsTo");
spG2Host1->PutDataTechnology(L"Tasks",L"DAO.DBEngine.120;DAO.DBEngine.36");
spG2Host1->PutDataSource(L"Tasks",spG2Host1->GetDataSource(L"Items"));
spG2Host1->PutDataMember(L"Tasks",L"EmployeeDetails");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksDataSource,L"Tasks");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksItemID,L"EmployeeID");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksStart,L"DateStart");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksEnd,L"DateEnd");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksID,L"TaskID");
spG2Host1->PutDataTechnology(L"Links",L"DAO.DBEngine.120;DAO.DBEngine.36");
spG2Host1->PutDataSource(L"Links",spG2Host1->GetDataSource(L"Items"));
spG2Host1->PutDataMember(L"Links",L"EmployeeLinks");
spG2Host1->PutDataField(EXG2HOSTLib::exLinksDataSource,L"Links");
spG2Host1->PutDataField(EXG2HOSTLib::exLinksStart,L"Start");
spG2Host1->PutDataField(EXG2HOSTLib::exLinksEnd,L"End");
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2ANTTLib' for the library: 'ExG2antt 1.0 Control Library'
#import <ExG2antt.dll>
using namespace EXG2ANTTLib;
*/
EXG2ANTTLib::IBarPtr var_Bar = ((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()))->GetChart()->GetBars()->GetItem("Task");
var_Bar->PutOverlaidType(EXG2ANTTLib::OverlaidBarsTypeEnum(EXG2ANTTLib::exOverlaidBarsStackAutoArrange | EXG2ANTTLib::exOverlaidBarsStack));
var_Bar->PutOverlaidGroup(L"Task,Progress");
((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()))->GetItems()->PutExpandItem(0,VARIANT_TRUE);
((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()))->GetChart()->ScrollTo(COleDateTime(2017,5,27,0,00,00).operator DATE(),long(1));
|
17
|
DAO sample ( object, DAO.DBEngine.120, single task, single table )
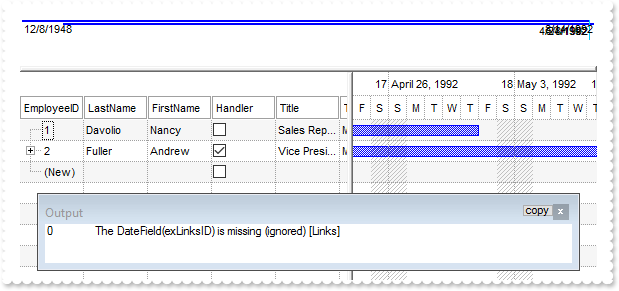
// Error event - Fired when an internal error occurs.
void OnErrorG2Host1(long Error,LPCTSTR Description)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
OutputDebugStringW( L"Error" );
OutputDebugStringW( L"Description" );
}
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'DAO' for the library: 'Microsoft Office 15.0 Access database engine Object Library'
#import <ACEDAO.DLL>
*/
DAO::_DBEnginePtr var_PrivDBEngine = ::CreateObject(L"DAO.DBEngine.120");
DAO::DatabasePtr var_Database = var_PrivDBEngine->OpenDatabase(L"C:\\Program Files\\Exontrol\\ExG2Host\\Sample\\Access\\sample.accdb",vtMissing,vtMissing,vtMissing);
DAO::Recordset2Ptr rsEmployees = ((DAO::Recordset2Ptr)(var_Database->OpenRecordset(L"Employees",vtMissing,vtMissing,vtMissing)));
DAO::Recordset2Ptr rsLinks = ((DAO::Recordset2Ptr)(var_Database->OpenRecordset(L"EmployeeLinks",vtMissing,vtMissing,vtMissing)));
spG2Host1->PutDataSource(L"Items",((DAO::Recordset2Ptr)(rsEmployees)));
spG2Host1->PutDataField(EXG2HOSTLib::exItemsDataSource,L"Items");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsID,L"EmployeeID");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsParentID,L"ReportsTo");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksDataSource,spG2Host1->GetDataField(EXG2HOSTLib::exItemsDataSource));
spG2Host1->PutDataField(EXG2HOSTLib::exTasksStart,L"BirthDate");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksEnd,L"HireDate");
spG2Host1->PutDataSource(L"Links",((DAO::Recordset2Ptr)(rsLinks)));
spG2Host1->PutDataField(EXG2HOSTLib::exLinksDataSource,L"Links");
spG2Host1->PutDataField(EXG2HOSTLib::exLinksStart,L"Start");
spG2Host1->PutDataField(EXG2HOSTLib::exLinksEnd,L"End");
|
16
|
DAO sample ( file, single task, single table )
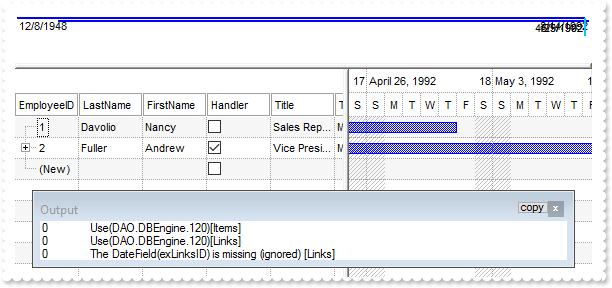
// Error event - Fired when an internal error occurs.
void OnErrorG2Host1(long Error,LPCTSTR Description)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
OutputDebugStringW( L"Error" );
OutputDebugStringW( L"Description" );
}
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
spG2Host1->PutDataTechnology(L"Items",L"DAO.DBEngine.120;DAO.DBEngine.36");
spG2Host1->PutDataSource(L"Items","C:\\Program Files\\Exontrol\\ExG2Host\\Sample\\Access\\sample.accdb");
spG2Host1->PutDataMember(L"Items",L"Employees");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsDataSource,L"Items");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsID,L"EmployeeID");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsParentID,L"ReportsTo");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksDataSource,spG2Host1->GetDataField(EXG2HOSTLib::exItemsDataSource));
spG2Host1->PutDataField(EXG2HOSTLib::exTasksStart,L"BirthDate");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksEnd,L"HireDate");
spG2Host1->PutDataTechnology(L"Links",L"DAO.DBEngine.120;DAO.DBEngine.36");
spG2Host1->PutDataSource(L"Links",spG2Host1->GetDataSource(L"Items"));
spG2Host1->PutDataMember(L"Links",L"EmployeeLinks");
spG2Host1->PutDataField(EXG2HOSTLib::exLinksDataSource,L"Links");
spG2Host1->PutDataField(EXG2HOSTLib::exLinksStart,L"Start");
spG2Host1->PutDataField(EXG2HOSTLib::exLinksEnd,L"End");
|
15
|
DAO sample ( tree recordset )
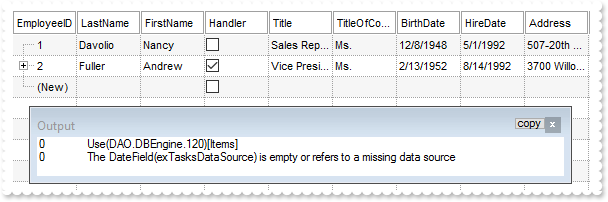
// Error event - Fired when an internal error occurs.
void OnErrorG2Host1(long Error,LPCTSTR Description)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
OutputDebugStringW( L"Error" );
OutputDebugStringW( L"Description" );
}
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
spG2Host1->PutDataTechnology(L"Items",L"DAO.DBEngine.120;DAO.DBEngine.36");
spG2Host1->PutDataSource(L"Items","C:\\Program Files\\Exontrol\\ExG2Host\\Sample\\Access\\sample.accdb");
spG2Host1->PutDataMember(L"Items",L"Employees");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsDataSource,L"Items");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsID,L"EmployeeID");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsParentID,L"ReportsTo");
|
14
|
DAO sample ( flat recordset )
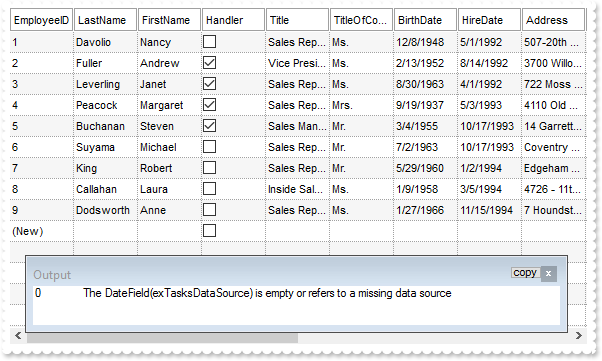
// Error event - Fired when an internal error occurs.
void OnErrorG2Host1(long Error,LPCTSTR Description)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
OutputDebugStringW( L"Error" );
OutputDebugStringW( L"Description" );
}
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'DAO' for the library: 'Microsoft Office 15.0 Access database engine Object Library'
#import <ACEDAO.DLL>
*/
DAO::_DBEnginePtr var_PrivDBEngine = ::CreateObject(L"DAO.DBEngine.120");
DAO::DatabasePtr var_Database = var_PrivDBEngine->OpenDatabase(L"C:\\Program Files\\Exontrol\\ExG2Host\\Sample\\Access\\sample.accdb",vtMissing,vtMissing,vtMissing);
DAO::Recordset2Ptr rsEmployees = ((DAO::Recordset2Ptr)(var_Database->OpenRecordset(L"Employees",vtMissing,vtMissing,vtMissing)));
spG2Host1->PutDataSource(L"Items",((DAO::Recordset2Ptr)(rsEmployees)));
spG2Host1->PutDataField(EXG2HOSTLib::exItemsDataSource,L"Items");
|
13
|
DAO sample ( flat )
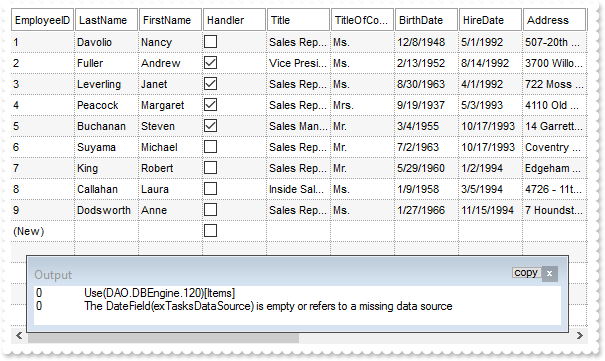
// Error event - Fired when an internal error occurs.
void OnErrorG2Host1(long Error,LPCTSTR Description)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
OutputDebugStringW( L"Error" );
OutputDebugStringW( L"Description" );
}
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
spG2Host1->PutDataTechnology(L"Items",L"DAO.DBEngine.120;DAO.DBEngine.36");
spG2Host1->PutDataSource(L"Items","C:\\Program Files\\Exontrol\\ExG2Host\\Sample\\Access\\sample.accdb");
spG2Host1->PutDataMember(L"Items",L"Employees");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsDataSource,L"Items");
|
12
|
ADO sample ( object, ADODB.Recordset, multiple tasks )
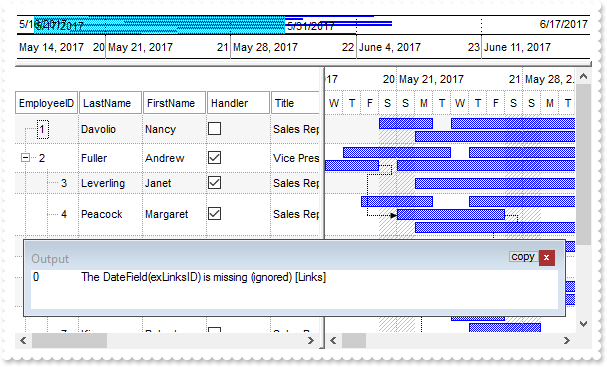
// Error event - Fired when an internal error occurs.
void OnErrorG2Host1(long Error,LPCTSTR Description)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
OutputDebugStringW( L"Error" );
OutputDebugStringW( L"Description" );
}
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'ADODB' for the library: 'Microsoft ActiveX Data Objects 6.1 Library'
#import <msado15.dll> rename("EOF","REOF")
*/
ADODB::_RecordsetPtr rsEmployees = ::CreateObject(L"ADODB.Recordset");
rsEmployees->Open("Employees","Provider=Microsoft.ACE.OLEDB.12.0;Data Source=C:\\Program Files\\Exontrol\\ExG2Host\\Sample\\Access\\sample.accdb",ADODB::adOpenStatic,ADODB::adLockOptimistic,0);
spG2Host1->PutDataSource(L"Items",((ADODB::_RecordsetPtr)(rsEmployees)));
spG2Host1->PutDataField(EXG2HOSTLib::exItemsDataSource,L"Items");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsID,L"EmployeeID");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsParentID,L"ReportsTo");
ADODB::_RecordsetPtr rsTasks = ::CreateObject(L"ADODB.Recordset");
rsTasks->Open("EmployeeDetails","Provider=Microsoft.ACE.OLEDB.12.0;Data Source=C:\\Program Files\\Exontrol\\ExG2Host\\Sample\\Access\\sample.accdb",ADODB::adOpenStatic,ADODB::adLockOptimistic,0);
spG2Host1->PutDataSource(L"Tasks",((ADODB::_RecordsetPtr)(rstasks)));
spG2Host1->PutDataField(EXG2HOSTLib::exTasksDataSource,L"Tasks");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksItemID,L"EmployeeID");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksStart,L"DateStart");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksEnd,L"DateEnd");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksID,L"TaskID");
ADODB::_RecordsetPtr rsLinks = ::CreateObject(L"ADODB.Recordset");
rsLinks->Open("EmployeeLinks","Provider=Microsoft.ACE.OLEDB.12.0;Data Source=C:\\Program Files\\Exontrol\\ExG2Host\\Sample\\Access\\sample.accdb",ADODB::adOpenStatic,ADODB::adLockOptimistic,0);
spG2Host1->PutDataSource(L"Links",((ADODB::_RecordsetPtr)(rsLinks)));
spG2Host1->PutDataField(EXG2HOSTLib::exLinksDataSource,L"Links");
spG2Host1->PutDataField(EXG2HOSTLib::exLinksStart,L"Start");
spG2Host1->PutDataField(EXG2HOSTLib::exLinksEnd,L"End");
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2ANTTLib' for the library: 'ExG2antt 1.0 Control Library'
#import <ExG2antt.dll>
using namespace EXG2ANTTLib;
*/
EXG2ANTTLib::IBarPtr var_Bar = ((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()))->GetChart()->GetBars()->GetItem("Task");
var_Bar->PutOverlaidType(EXG2ANTTLib::OverlaidBarsTypeEnum(EXG2ANTTLib::exOverlaidBarsStackAutoArrange | EXG2ANTTLib::exOverlaidBarsStack));
var_Bar->PutOverlaidGroup(L"Task,Progress");
((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()))->GetItems()->PutExpandItem(0,VARIANT_TRUE);
((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()))->GetChart()->ScrollTo(COleDateTime(2017,5,27,0,00,00).operator DATE(),long(1));
|
11
|
ADO sample ( file, multiple tasks, multiple tables )
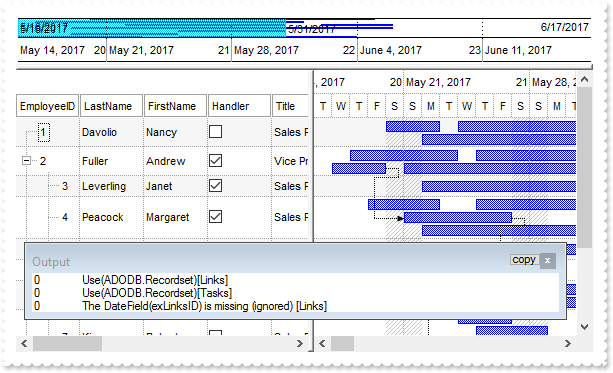
// Error event - Fired when an internal error occurs.
void OnErrorG2Host1(long Error,LPCTSTR Description)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
OutputDebugStringW( L"Error" );
OutputDebugStringW( L"Description" );
}
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
spG2Host1->PutDataTechnology(L"Items",L"ADODB.Recordset;ADOR.Recordset");
spG2Host1->PutDataSource(L"Items","C:\\Program Files\\Exontrol\\ExG2Host\\Sample\\Access\\sample.accdb");
spG2Host1->PutDataMember(L"Items",L"Employees");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsDataSource,L"Items");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsID,L"EmployeeID");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsParentID,L"ReportsTo");
spG2Host1->PutDataTechnology(L"Tasks",L"ADODB.Recordset;ADOR.Recordset");
spG2Host1->PutDataSource(L"Tasks",spG2Host1->GetDataSource(L"Items"));
spG2Host1->PutDataMember(L"Tasks",L"EmployeeDetails");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksDataSource,L"Tasks");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksItemID,L"EmployeeID");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksStart,L"DateStart");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksEnd,L"DateEnd");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksID,L"TaskID");
spG2Host1->PutDataTechnology(L"Links",L"ADODB.Recordset;ADOR.Recordset");
spG2Host1->PutDataSource(L"Links",spG2Host1->GetDataSource(L"Items"));
spG2Host1->PutDataMember(L"Links",L"EmployeeLinks");
spG2Host1->PutDataField(EXG2HOSTLib::exLinksDataSource,L"Links");
spG2Host1->PutDataField(EXG2HOSTLib::exLinksStart,L"Start");
spG2Host1->PutDataField(EXG2HOSTLib::exLinksEnd,L"End");
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2ANTTLib' for the library: 'ExG2antt 1.0 Control Library'
#import <ExG2antt.dll>
using namespace EXG2ANTTLib;
*/
EXG2ANTTLib::IBarPtr var_Bar = ((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()))->GetChart()->GetBars()->GetItem("Task");
var_Bar->PutOverlaidType(EXG2ANTTLib::OverlaidBarsTypeEnum(EXG2ANTTLib::exOverlaidBarsStackAutoArrange | EXG2ANTTLib::exOverlaidBarsStack));
var_Bar->PutOverlaidGroup(L"Task,Progress");
((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()))->GetItems()->PutExpandItem(0,VARIANT_TRUE);
((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()))->GetChart()->ScrollTo(COleDateTime(2017,5,27,0,00,00).operator DATE(),long(1));
|
10
|
ADO sample ( object, ADODB.Recordset, single task, single table )
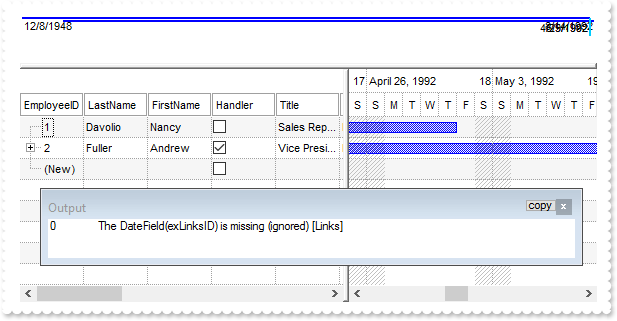
// Error event - Fired when an internal error occurs.
void OnErrorG2Host1(long Error,LPCTSTR Description)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
OutputDebugStringW( L"Error" );
OutputDebugStringW( L"Description" );
}
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'ADODB' for the library: 'Microsoft ActiveX Data Objects 6.1 Library'
#import <msado15.dll> rename("EOF","REOF")
*/
ADODB::_RecordsetPtr rsEmployees = ::CreateObject(L"ADODB.Recordset");
rsEmployees->Open("Employees","Provider=Microsoft.ACE.OLEDB.12.0;Data Source=C:\\Program Files\\Exontrol\\ExG2Host\\Sample\\Access\\sample.accdb",ADODB::adOpenStatic,ADODB::adLockOptimistic,0);
spG2Host1->PutDataSource(L"Items",((ADODB::_RecordsetPtr)(rsEmployees)));
spG2Host1->PutDataField(EXG2HOSTLib::exItemsDataSource,L"Items");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsID,L"EmployeeID");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsParentID,L"ReportsTo");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksDataSource,spG2Host1->GetDataField(EXG2HOSTLib::exItemsDataSource));
spG2Host1->PutDataField(EXG2HOSTLib::exTasksStart,L"BirthDate");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksEnd,L"HireDate");
ADODB::_RecordsetPtr rsLinks = ::CreateObject(L"ADODB.Recordset");
rsLinks->Open("EmployeeLinks","Provider=Microsoft.ACE.OLEDB.12.0;Data Source=C:\\Program Files\\Exontrol\\ExG2Host\\Sample\\Access\\sample.accdb",ADODB::adOpenStatic,ADODB::adLockOptimistic,0);
spG2Host1->PutDataSource(L"Links",((ADODB::_RecordsetPtr)(rsLinks)));
spG2Host1->PutDataField(EXG2HOSTLib::exLinksDataSource,L"Links");
spG2Host1->PutDataField(EXG2HOSTLib::exLinksStart,L"Start");
spG2Host1->PutDataField(EXG2HOSTLib::exLinksEnd,L"End");
|
9
|
ADO sample ( file, single task, single table )
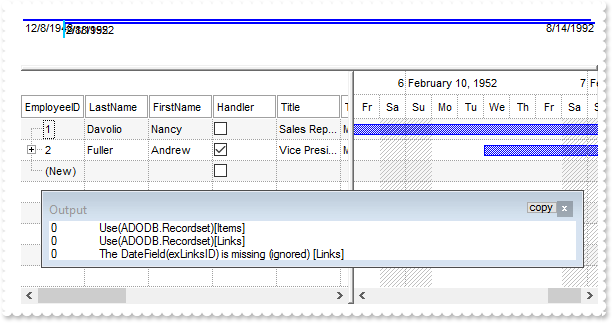
// Error event - Fired when an internal error occurs.
void OnErrorG2Host1(long Error,LPCTSTR Description)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
OutputDebugStringW( L"Error" );
OutputDebugStringW( L"Description" );
}
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
spG2Host1->PutDataTechnology(L"Items",L"ADODB.Recordset;ADOR.Recordset");
spG2Host1->PutDataSource(L"Items","C:\\Program Files\\Exontrol\\ExG2Host\\Sample\\Access\\sample.accdb");
spG2Host1->PutDataMember(L"Items",L"Employees");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsDataSource,L"Items");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsID,L"EmployeeID");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsParentID,L"ReportsTo");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksDataSource,spG2Host1->GetDataField(EXG2HOSTLib::exItemsDataSource));
spG2Host1->PutDataField(EXG2HOSTLib::exTasksStart,L"BirthDate");
spG2Host1->PutDataField(EXG2HOSTLib::exTasksEnd,L"HireDate");
spG2Host1->PutDataTechnology(L"Links",L"ADODB.Recordset;ADOR.Recordset");
spG2Host1->PutDataSource(L"Links",spG2Host1->GetDataSource(L"Items"));
spG2Host1->PutDataMember(L"Links",L"EmployeeLinks");
spG2Host1->PutDataField(EXG2HOSTLib::exLinksDataSource,L"Links");
spG2Host1->PutDataField(EXG2HOSTLib::exLinksStart,L"Start");
spG2Host1->PutDataField(EXG2HOSTLib::exLinksEnd,L"End");
|
8
|
ADO sample ( tree recordset )
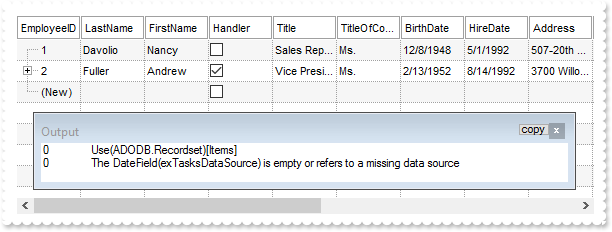
// Error event - Fired when an internal error occurs.
void OnErrorG2Host1(long Error,LPCTSTR Description)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
OutputDebugStringW( L"Error" );
OutputDebugStringW( L"Description" );
}
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
spG2Host1->PutDataTechnology(L"Items",L"ADODB.Recordset;ADOR.Recordset");
spG2Host1->PutDataSource(L"Items","C:\\Program Files\\Exontrol\\ExG2Host\\Sample\\Access\\sample.accdb");
spG2Host1->PutDataMember(L"Items",L"Employees");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsDataSource,L"Items");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsID,L"EmployeeID");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsParentID,L"ReportsTo");
|
7
|
ADO sample ( flat recordset )
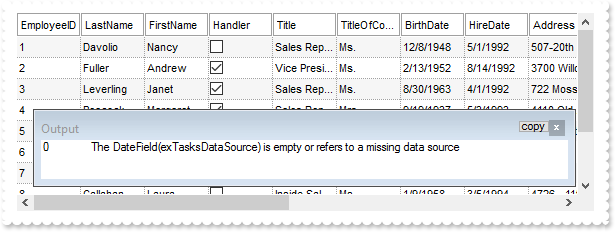
// Error event - Fired when an internal error occurs.
void OnErrorG2Host1(long Error,LPCTSTR Description)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
OutputDebugStringW( L"Error" );
OutputDebugStringW( L"Description" );
}
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'ADODB' for the library: 'Microsoft ActiveX Data Objects 6.1 Library'
#import <msado15.dll> rename("EOF","REOF")
*/
ADODB::_RecordsetPtr rsEmployees = ::CreateObject(L"ADODB.Recordset");
rsEmployees->Open("Employees","Provider=Microsoft.ACE.OLEDB.12.0;Data Source=C:\\Program Files\\Exontrol\\ExG2Host\\Sample\\Access\\sample.accdb",ADODB::adOpenStatic,ADODB::adLockOptimistic,0);
spG2Host1->PutDataSource(L"Items",((ADODB::_RecordsetPtr)(rsEmployees)));
spG2Host1->PutDataField(EXG2HOSTLib::exItemsDataSource,L"Items");
|
6
|
ADO sample ( flat table )
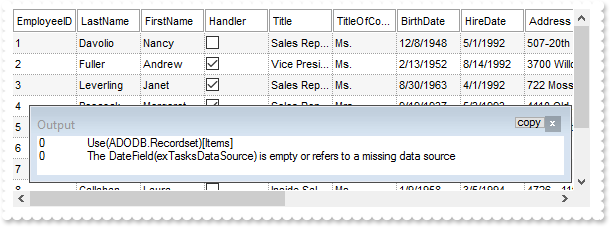
// Error event - Fired when an internal error occurs.
void OnErrorG2Host1(long Error,LPCTSTR Description)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
OutputDebugStringW( L"Error" );
OutputDebugStringW( L"Description" );
}
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
spG2Host1->PutDataTechnology(L"Items",L"ADODB.Recordset;ADOR.Recordset");
spG2Host1->PutDataSource(L"Items","C:\\Program Files\\Exontrol\\ExG2Host\\Sample\\Access\\sample.accdb");
spG2Host1->PutDataMember(L"Items",L"Employees");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsDataSource,L"Items");
|
5
|
XML sample ( object, MSXML.DOMDocument )
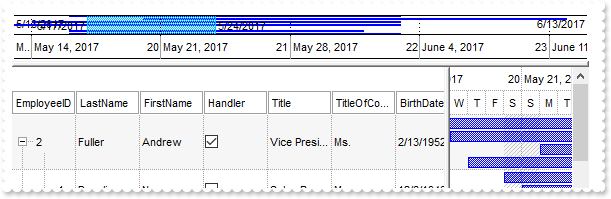
// Error event - Fired when an internal error occurs.
void OnErrorG2Host1(long Error,LPCTSTR Description)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
OutputDebugStringW( L"Error" );
OutputDebugStringW( L"Description" );
}
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'MSXML2' for the library: 'Microsoft XML, v3.0'
#import <msxml3.dll>
*/
MSXML2::IXMLDOMDocument2Ptr xml = ::CreateObject(L"MSXML.DOMDocument");
xml->Putasync(VARIANT_FALSE);
xml->load("C:\\Program Files\\Exontrol\\ExG2Host\\Sample\\sample.xml");
spG2Host1->PutDataSource(L"Items",((MSXML2::IXMLDOMDocument2Ptr)(xml)));
spG2Host1->PutDataField(EXG2HOSTLib::exItemsDataSource,L"Items");
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2ANTTLib' for the library: 'ExG2antt 1.0 Control Library'
#import <ExG2antt.dll>
using namespace EXG2ANTTLib;
*/
EXG2ANTTLib::IG2anttPtr var_G2antt = ((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()));
var_G2antt->PutLinesAtRoot(EXG2ANTTLib::exLinesAtRoot);
var_G2antt->PutSingleSel(VARIANT_FALSE);
var_G2antt->PutAutoDrag(EXG2ANTTLib::exAutoDragPositionAny);
|
4
|
XML sample ( file tree )
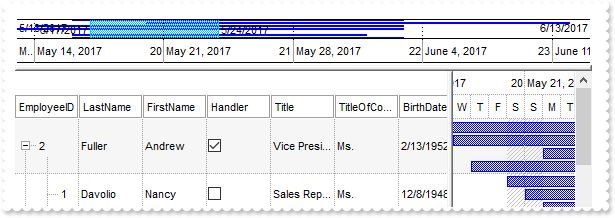
// Error event - Fired when an internal error occurs.
void OnErrorG2Host1(long Error,LPCTSTR Description)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
OutputDebugStringW( L"Error" );
OutputDebugStringW( L"Description" );
}
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
spG2Host1->PutDataTechnology(L"Items",L"MSXML.DOMDocument");
spG2Host1->PutDataSource(L"Items","C:\\Program Files\\Exontrol\\ExG2Host\\Sample\\sample.xml");
spG2Host1->PutDataField(EXG2HOSTLib::exItemsDataSource,L"Items");
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2ANTTLib' for the library: 'ExG2antt 1.0 Control Library'
#import <ExG2antt.dll>
using namespace EXG2ANTTLib;
*/
EXG2ANTTLib::IG2anttPtr var_G2antt = ((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()));
var_G2antt->PutLinesAtRoot(EXG2ANTTLib::exLinesAtRoot);
var_G2antt->PutSingleSel(VARIANT_FALSE);
var_G2antt->PutAutoDrag(EXG2ANTTLib::exAutoDragPositionAny);
|
3
|
How can I let user create new items/bars when clicking the empty area of the control
// HostEvent event - Notifies the application once the host fires an event.
void OnHostEventG2Host1(long EventID)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
OutputDebugStringW( _bstr_t(spG2Host1->GetHostEventParam(-2)) );
}
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
spG2Host1->PutHostReadOnly(EXG2HOSTLib::HostReadOnlyEnum(EXG2HOSTLib::exHostReadWrite | EXG2HOSTLib::exHostAllowAddEmptyItem));
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2ANTTLib' for the library: 'ExG2antt 1.0 Control Library'
#import <ExG2antt.dll>
using namespace EXG2ANTTLib;
*/
EXG2ANTTLib::IG2anttPtr var_G2antt = ((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()));
var_G2antt->PutScrollBars(EXG2ANTTLib::ScrollBarsEnum(EXG2ANTTLib::exVScrollEmptySpace | EXG2ANTTLib::exDisableNoVertical));
EXG2ANTTLib::IChartPtr var_Chart = var_G2antt->GetChart();
var_Chart->PutPaneWidth(VARIANT_FALSE,128);
var_Chart->PutAllowCreateBar(EXG2ANTTLib::exCreateBarAuto);
|
2
|
How do I handle events of the host
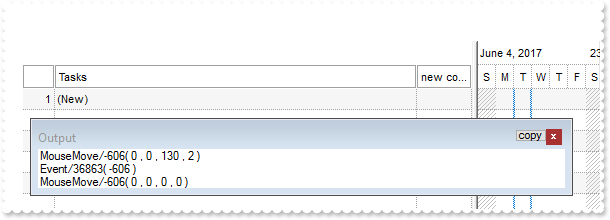
// HostEvent event - Notifies the application once the host fires an event.
void OnHostEventG2Host1(long EventID)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
OutputDebugStringW( _bstr_t(spG2Host1->GetHostEventParam(-2)) );
}
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2ANTTLib' for the library: 'ExG2antt 1.0 Control Library'
#import <ExG2antt.dll>
using namespace EXG2ANTTLib;
*/
EXG2ANTTLib::IG2anttPtr var_G2antt = ((EXG2ANTTLib::IG2anttPtr)(spG2Host1->GetHost()));
var_G2antt->BeginUpdate();
var_G2antt->GetColumns()->Add(L"new column");
var_G2antt->EndUpdate();
|
1
|
How can I get the version of the host/exg2antt control
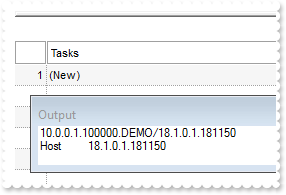
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXG2HOSTLib' for the library: 'ExG2Host 1.0 Control Library'
#import <ExG2Host.dll>
using namespace EXG2HOSTLib;
*/
EXG2HOSTLib::IG2HostPtr spG2Host1 = GetDlgItem(IDC_G2HOST1)->GetControlUnknown();
OutputDebugStringW( spG2Host1->GetVersion() );
OutputDebugStringW( L"Host" );
OutputDebugStringW( spG2Host1->GetHost()->GetVersion() );
|